filmov
tv
How to Use the Spread Operator to Effectively Update Objects in an Array in JavaScript
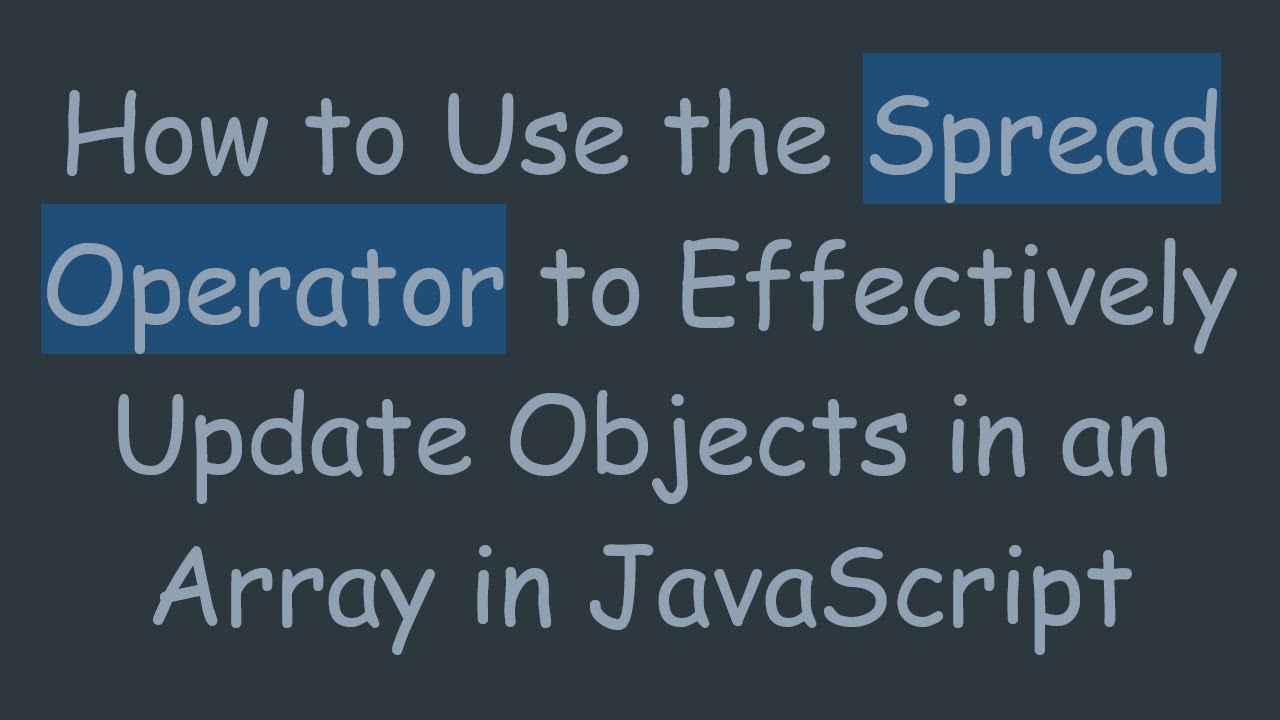
Показать описание
Learn how to efficiently update specific properties of an object in an array using the Spread Operator in JavaScript. This guide will help you streamline your code and enhance your React or TypeScript applications.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Spread operator to update array of objects
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering the Spread Operator for Array of Objects in JavaScript
Updating an object within an array can be a common task in JavaScript, especially in frameworks like React and TypeScript. You might encounter situations where you need to modify specific properties of an object while leaving the rest of the properties intact. This guide aims to solve such a problem using the Spread Operator — a powerful feature in JavaScript that allows you to create a shallow copy of an object or an array.
The Problem: Updating Properties in an Array of Objects
Let's say you have an array of objects structured in a certain way that represents required fields for a form. Each object in this array has various properties such as name, isValid, content, etc. You want to update just a few of these properties for a specific object in response to some input.
Here’s an example of the array structure you might be working with:
[[See Video to Reveal this Text or Code Snippet]]
If you try to find and update an object using a single assignment, you may run into issues where the state does not reflect the changes as intended.
The Solution: Using the Spread Operator
To effectively update specific properties in an object within an array, follow these structured steps:
Step 1: Find the Index of the Object
First, you need to locate the object you wish to update. You can use the findIndex method to get its index. The following code snippet illustrates how to do this:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Copy the Current State
Once you have the index, create a copy of the current state using the spread operator to avoid direct mutation, which is a vital step in maintaining state integrity.
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Update the Specific Object
Next, update only the properties you need, such as isValid, content, and isUnchanged for the found object at the specified index.
[[See Video to Reveal this Text or Code Snippet]]
Step 4: Set the New State
Finally, use your state update function (like setRequiredFields in React) to apply the updated state.
[[See Video to Reveal this Text or Code Snippet]]
Complete Code Example
Putting all these steps together, your complete function might look like this:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By using the Spread Operator, you can efficiently manage and update the specific properties of an object within an array in JavaScript. This method not only enhances the readability of your code but also helps you maintain the principles of immutability in state management, especially in React or Typescript applications. With these techniques, you are well-equipped to handle similar problems in your projects. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Spread operator to update array of objects
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering the Spread Operator for Array of Objects in JavaScript
Updating an object within an array can be a common task in JavaScript, especially in frameworks like React and TypeScript. You might encounter situations where you need to modify specific properties of an object while leaving the rest of the properties intact. This guide aims to solve such a problem using the Spread Operator — a powerful feature in JavaScript that allows you to create a shallow copy of an object or an array.
The Problem: Updating Properties in an Array of Objects
Let's say you have an array of objects structured in a certain way that represents required fields for a form. Each object in this array has various properties such as name, isValid, content, etc. You want to update just a few of these properties for a specific object in response to some input.
Here’s an example of the array structure you might be working with:
[[See Video to Reveal this Text or Code Snippet]]
If you try to find and update an object using a single assignment, you may run into issues where the state does not reflect the changes as intended.
The Solution: Using the Spread Operator
To effectively update specific properties in an object within an array, follow these structured steps:
Step 1: Find the Index of the Object
First, you need to locate the object you wish to update. You can use the findIndex method to get its index. The following code snippet illustrates how to do this:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Copy the Current State
Once you have the index, create a copy of the current state using the spread operator to avoid direct mutation, which is a vital step in maintaining state integrity.
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Update the Specific Object
Next, update only the properties you need, such as isValid, content, and isUnchanged for the found object at the specified index.
[[See Video to Reveal this Text or Code Snippet]]
Step 4: Set the New State
Finally, use your state update function (like setRequiredFields in React) to apply the updated state.
[[See Video to Reveal this Text or Code Snippet]]
Complete Code Example
Putting all these steps together, your complete function might look like this:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By using the Spread Operator, you can efficiently manage and update the specific properties of an object within an array in JavaScript. This method not only enhances the readability of your code but also helps you maintain the principles of immutability in state management, especially in React or Typescript applications. With these techniques, you are well-equipped to handle similar problems in your projects. Happy coding!