filmov
tv
Understanding the Singleton Pattern in C#: Different Implementations Explored
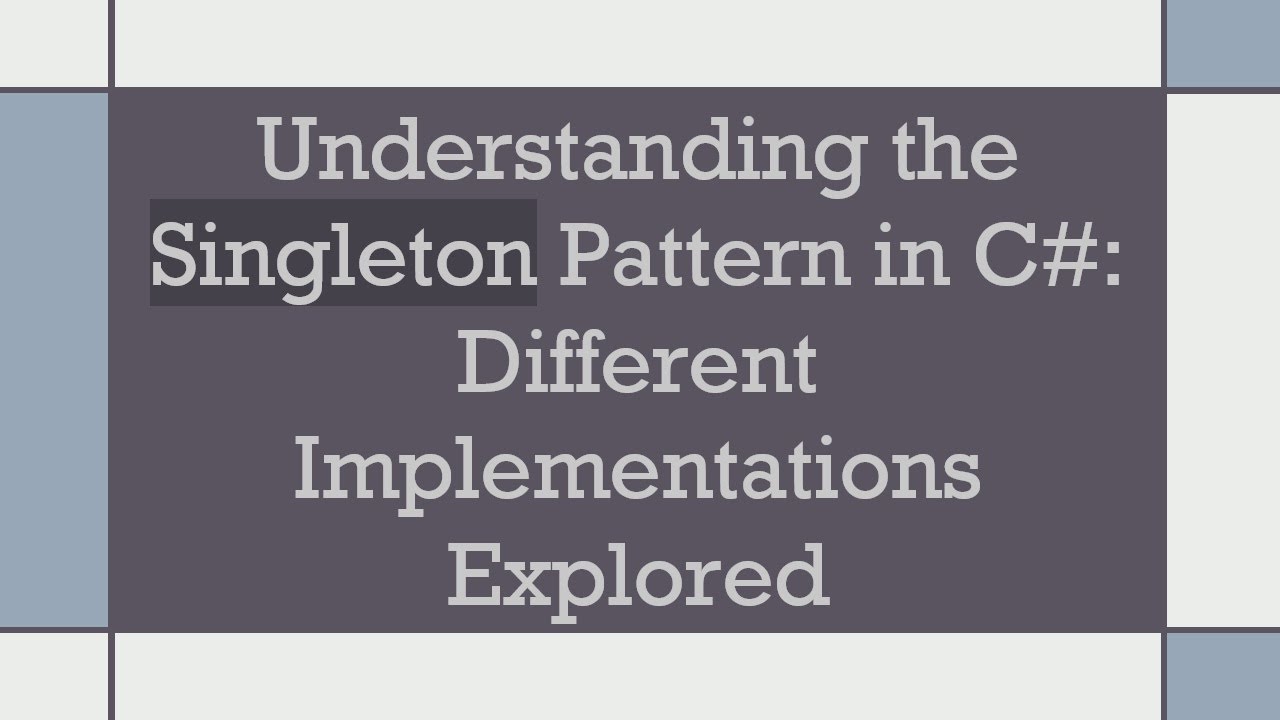
Показать описание
Dive into the nuances of the `Singleton` design pattern in C-. Learn about two different implementations and why proper testing methods are essential in your development process.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Proper singleton class implementation
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the Singleton Pattern in C-: Different Implementations Explored
The Singleton pattern is a fundamental design pattern that restricts the instantiation of a class to a single instance. When learning about design patterns, it's common to start with this pattern due to its straightforward nature and the numerous applications it has in software development. However, there are different ways to implement a Singleton, and understanding these variations can significantly impact the quality and testability of your code.
The Problem with Singleton Implementation
The typical Singleton implementation involves a static property to provide access to the instance. Here is a basic implementation:
[[See Video to Reveal this Text or Code Snippet]]
While this implementation is simple, it presents a challenge during unit testing. For example, consider a Client class that references the Singleton:
[[See Video to Reveal this Text or Code Snippet]]
This structure makes it almost impossible to mock the CallMe method for testing, creating a reliance on the Singleton that many developers aim to avoid.
Improved Singleton Implementation
To improve on this, modern practices suggest incorporating Dependency Injection (DI) methods. Here's an alternate implementation that uses Lazy<T>, which ensures that the instance is created only when it's needed:
[[See Video to Reveal this Text or Code Snippet]]
This solution is better in terms of initialization, but it still poses a risk of introducing tight coupling to the singleton instance. This is where Dependency Injection shines.
Using Dependency Injection for Singleton
Consider a refactored approach that utilizes Dependency Injection, allowing for easier testing and flexibility:
[[See Video to Reveal this Text or Code Snippet]]
Why Is This Approach Better?
Testability: By taking the Singleton instance via constructor injection, you can easily mock the Singleton in unit tests, making it easier to verify behavior without relying on the actual singleton instance.
Loose Coupling: Implementing DI results in a design that adheres to the Dependency Inversion Principle, leading to an architecture that is more flexible and easier to maintain.
Separation of Concerns: Each class has a clear responsibility. The Singleton class is responsible for its own instance management, while the Client class doesn't concern itself with how the instance is created.
Final Thoughts
The Singleton pattern may seem simple, but its implementation impacts the maintainability and testability of your application. By understanding the different ways to create singleton instances and utilizing Dependency Injection, you can ensure your code remains clean, testable, and adheres to the best practices of software design.
Embrace these concepts as you move forward in your software development journey, and see how they can reduce complexity and improve your code's overall quality.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Proper singleton class implementation
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the Singleton Pattern in C-: Different Implementations Explored
The Singleton pattern is a fundamental design pattern that restricts the instantiation of a class to a single instance. When learning about design patterns, it's common to start with this pattern due to its straightforward nature and the numerous applications it has in software development. However, there are different ways to implement a Singleton, and understanding these variations can significantly impact the quality and testability of your code.
The Problem with Singleton Implementation
The typical Singleton implementation involves a static property to provide access to the instance. Here is a basic implementation:
[[See Video to Reveal this Text or Code Snippet]]
While this implementation is simple, it presents a challenge during unit testing. For example, consider a Client class that references the Singleton:
[[See Video to Reveal this Text or Code Snippet]]
This structure makes it almost impossible to mock the CallMe method for testing, creating a reliance on the Singleton that many developers aim to avoid.
Improved Singleton Implementation
To improve on this, modern practices suggest incorporating Dependency Injection (DI) methods. Here's an alternate implementation that uses Lazy<T>, which ensures that the instance is created only when it's needed:
[[See Video to Reveal this Text or Code Snippet]]
This solution is better in terms of initialization, but it still poses a risk of introducing tight coupling to the singleton instance. This is where Dependency Injection shines.
Using Dependency Injection for Singleton
Consider a refactored approach that utilizes Dependency Injection, allowing for easier testing and flexibility:
[[See Video to Reveal this Text or Code Snippet]]
Why Is This Approach Better?
Testability: By taking the Singleton instance via constructor injection, you can easily mock the Singleton in unit tests, making it easier to verify behavior without relying on the actual singleton instance.
Loose Coupling: Implementing DI results in a design that adheres to the Dependency Inversion Principle, leading to an architecture that is more flexible and easier to maintain.
Separation of Concerns: Each class has a clear responsibility. The Singleton class is responsible for its own instance management, while the Client class doesn't concern itself with how the instance is created.
Final Thoughts
The Singleton pattern may seem simple, but its implementation impacts the maintainability and testability of your application. By understanding the different ways to create singleton instances and utilizing Dependency Injection, you can ensure your code remains clean, testable, and adheres to the best practices of software design.
Embrace these concepts as you move forward in your software development journey, and see how they can reduce complexity and improve your code's overall quality.