filmov
tv
Efficiently Perform Operations on Arrays in Python with Numpy
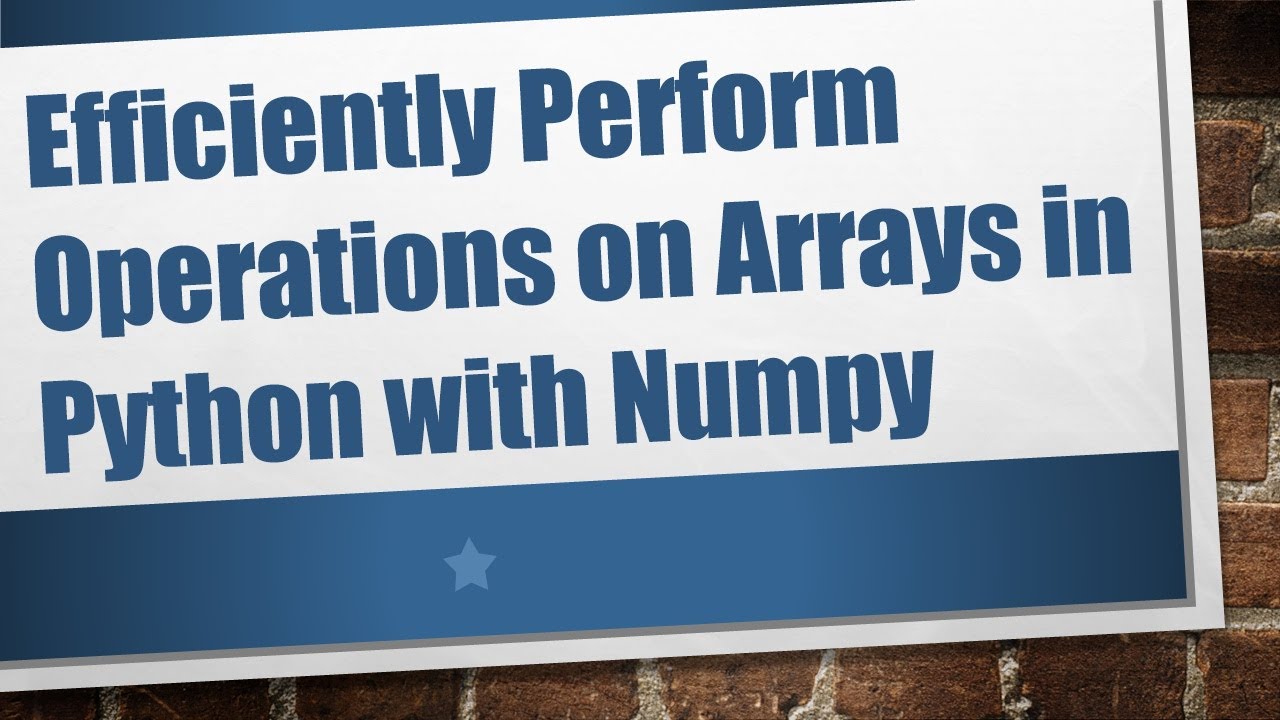
Показать описание
Learn how to perform operations on each element of an array and store the results in a new array using Python and Numpy. This guide covers loops and list comprehensions for efficient computation.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How would I perform an operation on each element in an array and add the new value to a new array in Python?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Efficiently Perform Operations on Arrays in Python with Numpy
Working with arrays in Python can sometimes feel overwhelming, especially when you want to perform operations on each element and store the results in a new array. If you've been wondering how to do this effectively using Numpy, you’re in the right place! In this guide, we’ll explore how to loop through an array, perform operations on its elements, and store the results in another array.
Understanding the Problem
Suppose you have an array of ten random values generated using Numpy. Your task is to square each of these values and store the results in a new array. While setting up the initial loop may seem straightforward, you might be confused about how to effectively handle array updates in Python. Let's break this down!
Example Code Provided
Here's the code you originally provided:
[[See Video to Reveal this Text or Code Snippet]]
While you set empty_array to zeros, the next step of updating this array with the squared values is where the confusion lies.
Solution Approaches
1. Using a List and append()
A classic way to handle this task is by initializing an empty list and using the append() method to add the squared values:
[[See Video to Reveal this Text or Code Snippet]]
Key Points:
The append() function adds a new item to the end of the list.
This method is straightforward and readable.
2. Leveraging List Comprehensions
If you prefer a more Pythonic approach, consider using list comprehensions. They provide a compact way to apply operations to each element of an array:
[[See Video to Reveal this Text or Code Snippet]]
Key Points:
This approach is concise and often more readable for those familiar with Python.
It avoids the explicit use of loops and is typically faster for large datasets.
Conclusion
Whether you opt for a traditional loop with append() or a more modern list comprehension, both methods effectively provide a solution to your problem of performing operations on an array and storing the results in a new array.
Remember:
Using append() is straightforward and works well for small tasks.
List comprehensions can simplify your code and improve performance for larger operations.
Now, you can handle arrays in Python with confidence! So go ahead, choose the method that best suits your style, and start manipulating arrays efficiently with Numpy.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How would I perform an operation on each element in an array and add the new value to a new array in Python?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Efficiently Perform Operations on Arrays in Python with Numpy
Working with arrays in Python can sometimes feel overwhelming, especially when you want to perform operations on each element and store the results in a new array. If you've been wondering how to do this effectively using Numpy, you’re in the right place! In this guide, we’ll explore how to loop through an array, perform operations on its elements, and store the results in another array.
Understanding the Problem
Suppose you have an array of ten random values generated using Numpy. Your task is to square each of these values and store the results in a new array. While setting up the initial loop may seem straightforward, you might be confused about how to effectively handle array updates in Python. Let's break this down!
Example Code Provided
Here's the code you originally provided:
[[See Video to Reveal this Text or Code Snippet]]
While you set empty_array to zeros, the next step of updating this array with the squared values is where the confusion lies.
Solution Approaches
1. Using a List and append()
A classic way to handle this task is by initializing an empty list and using the append() method to add the squared values:
[[See Video to Reveal this Text or Code Snippet]]
Key Points:
The append() function adds a new item to the end of the list.
This method is straightforward and readable.
2. Leveraging List Comprehensions
If you prefer a more Pythonic approach, consider using list comprehensions. They provide a compact way to apply operations to each element of an array:
[[See Video to Reveal this Text or Code Snippet]]
Key Points:
This approach is concise and often more readable for those familiar with Python.
It avoids the explicit use of loops and is typically faster for large datasets.
Conclusion
Whether you opt for a traditional loop with append() or a more modern list comprehension, both methods effectively provide a solution to your problem of performing operations on an array and storing the results in a new array.
Remember:
Using append() is straightforward and works well for small tasks.
List comprehensions can simplify your code and improve performance for larger operations.
Now, you can handle arrays in Python with confidence! So go ahead, choose the method that best suits your style, and start manipulating arrays efficiently with Numpy.