filmov
tv
Named functions in JavaScript - #16 @Everyday-Be-Coding
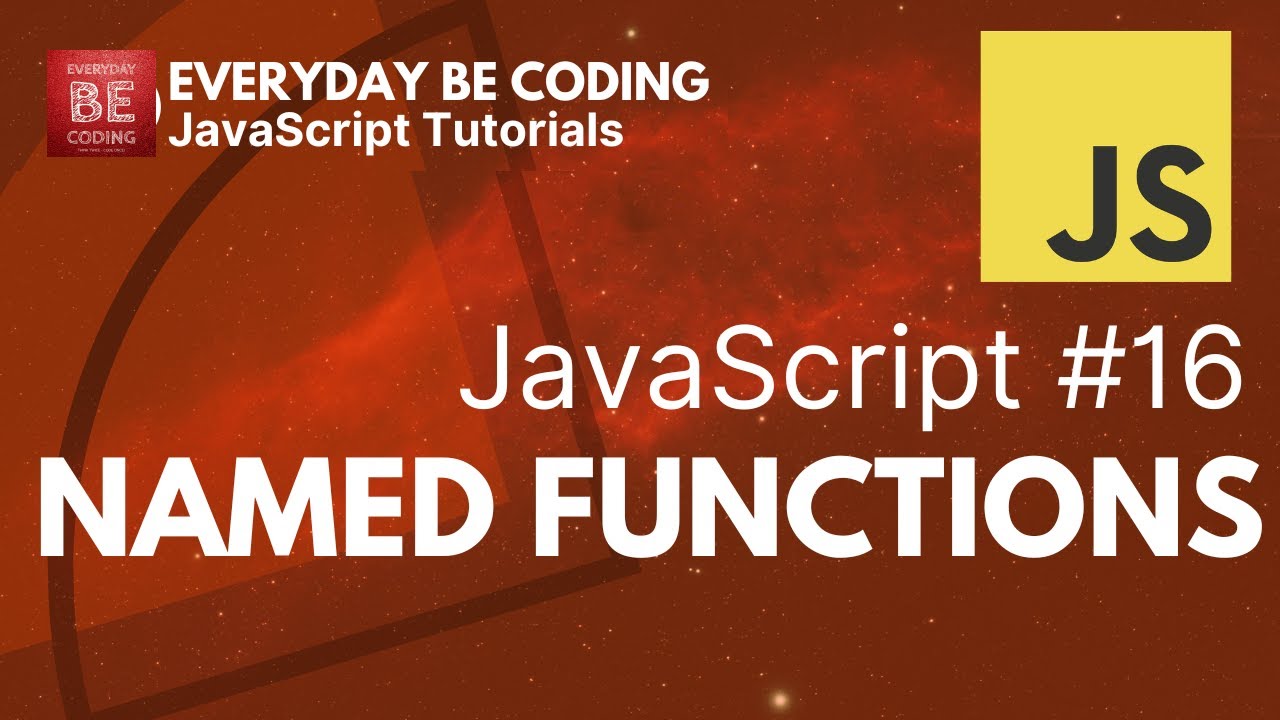
Показать описание
Named functions in JavaScript are functions that are declared with a specified name. They are created using the function keyword followed by the name of the function. Named functions are defined using function declarations or function expressions with named identifiers. Here's how they work:
Function Declarations:
In a function declaration, the function name is declared as a variable in the current scope.
JavaScript code:
function add(a, b) {
return a + b;
}
You can then call the function by its name:
JavaScript code:
Function Expressions:
Function expressions involve assigning a function to a variable. The function can still have a name in this case.
JavaScript Code
var multiply = function multiply(a, b) {
return a * b;
};
The function can be called using the variable name:
JavaScript code
Named functions are beneficial because:
They provide a meaningful name to the function, which improves code readability and maintainability.
They can be called recursively within their own scope.
They facilitate debugging, as the name of the function appears in stack traces, making it easier to identify where an error occurred.
However, it's worth noting that function expressions with names are optional. You can have anonymous function expressions as well. For example:
JavaScript code:
var greet = function(name) {
return "Hello, " + name + "!";
};
In this case, the function is anonymous, as it doesn't have a name. Named functions are particularly useful when you need to reference the function within its own body or when you want more descriptive stack traces for debugging purposes.
Chapter :
00:00 Introduction
00:20 Function Declarations
00:56 Function Expressions
01:20 Beneficial
Thank you for watching this video
Everyday Be Coding