filmov
tv
Python Tutorial: Intro to Object Oriented Programming in Python
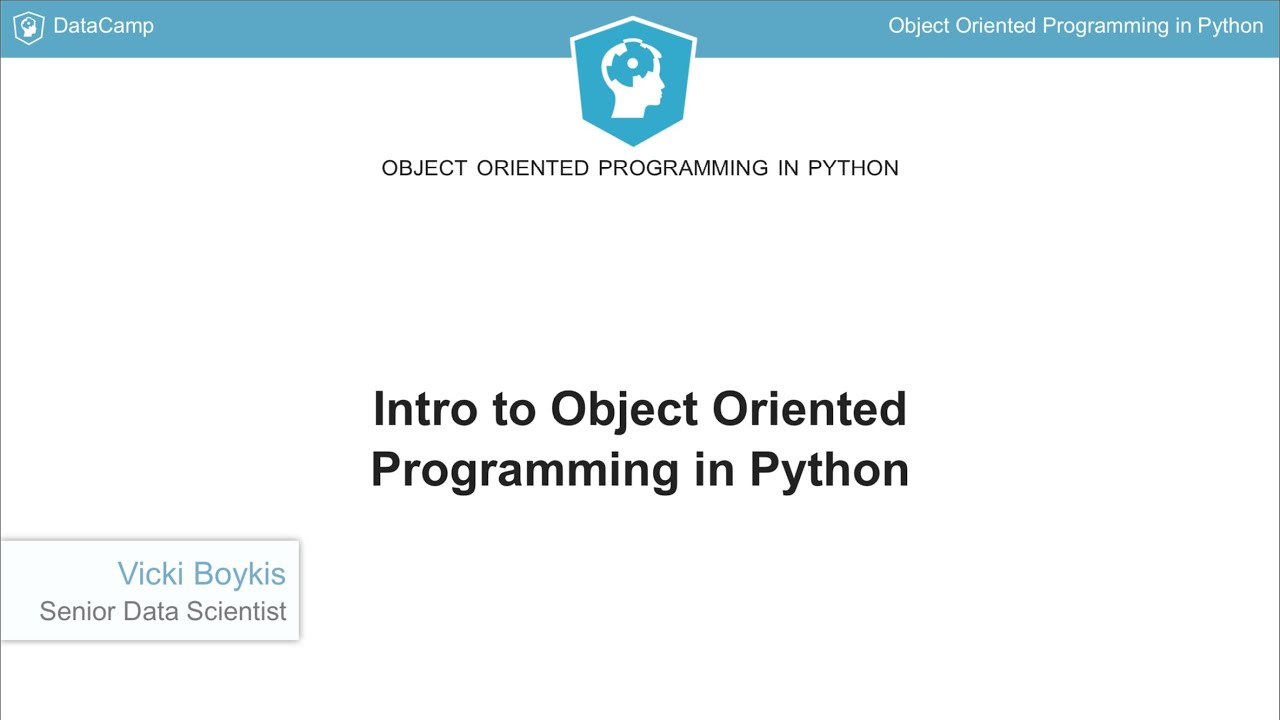
Показать описание
---
Hi! I'm Vicki Boykis, a senior data scientist and engineer.
Welcome to Object-Oriented Programming, or OOP, in Python. In this course, we'll take apart the Pandas DataFrame to see how NumPy arrays work. We'll do this to better understand the building blocks of Python objects and classes, and by the end, be able to build our own classes, as well.
Let's get started!
Have you ever heard the saying, "It's turtles all the way down?" The saying comes from a myth that the world rests on the back of a turtle, which itself sits on a large turtle, which itself sits on an even larger turtle, and so on to infinity.
Scientific programming is like that, too. All of the high-level packages we use as part of scientific computing in Python, including NumPy, Pandas, and Scikit-learn, are all based on a foundation, which builds into the complex libraries we use.
If you start with Pandas and work your way all the way to the bottom of the stack, you'll find NumPy arrays, which are being used to make a number of other, different things.
In this course, we'll get to the bottom of the turtles and figure out what the largest turtle, the Python object, really is.
So, what is object-oriented programming?
OOP, as it's otherwise known, is a way to make logical groups of variables and functions reusable for generalized tasks.
Think of a recipe when you're cooking. You work from a very specific list of ingredients in a very specific order to create a dish, as you can see in the picture on the upper left. This is OOP.
Not everything we do in Python has to be object-oriented.
Sometimes, we don't know what we'll need ahead of time, we plan and write functions and variables as we go, as you can see in the picture on the lower left.
We refer to this style of writing out functions and variables as imperative programming.
Either way, we make something delicious, the program on the right-hand side.
What do imperative and OOP styles look like?
You may be used to writing code that looks like the code in the first example. Here we have a list of numbers as a variable, our_list, and we print each item in that list from a function.
For small volumes of data, this works really well. But what if that list was part of another function, or we needed to call it and put in new values over and over again? That's the basis of object-oriented programming.
In the second example, we create a class, which is a template, or a recipe, that takes in a list of numbers and prints all the numbers in the list. We instantiate it with the PrintList call in the next-to-last line, and then call the print_list method of that class instance, or object.
How can we bridge these two concepts? Let's start at the bottom of the turtle.
Everything in Python is an object and the most powerful Python libraries we use every day include objects as building blocks.
A variable is a Python object. A list is a Python object that's build-out of variables. Here, we have a variable, 4, that then becomes part of a list [1,2,4]. A list is an advanced type of object with advanced logic for how to access elements.
At the second tier, we have, NumPy arrays, which are structured as lists of lists. We'll learn more about those in the next section. You can see that we took our list [1,2,4], and added another list to create an array or list of lists.
Finally, at the third tier, we have Pandas DataFrames, which are, are in turn built from NumPy arrays.
In this course, we'll cover how to go from basic Python building blocks like functions and variables, all the way to making our own version of a Pandas DataFrame that we call a DataShell in order to create more reproducible computing structures, and we'll do so with lots of analogies to explain the world of OOP.
Let's get started!