filmov
tv
C++ Weekly - Ep 97 - Lambda To Function Pointer Conversion
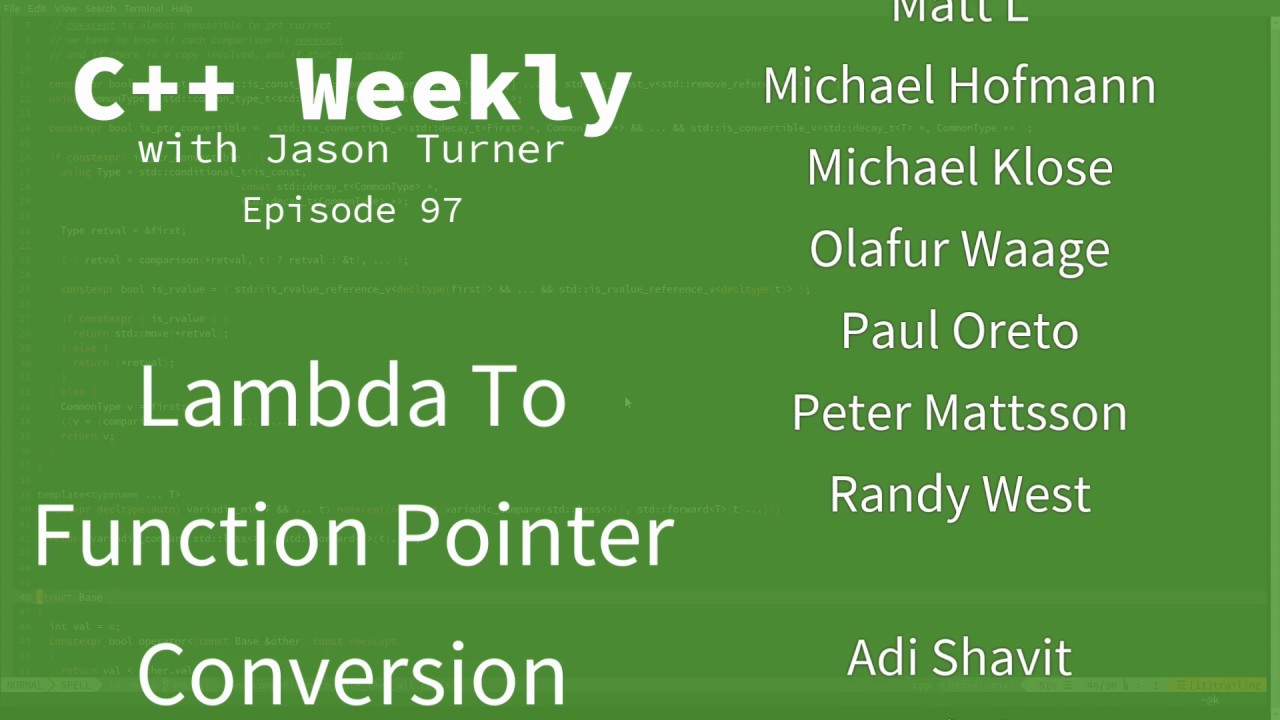
Показать описание
☟☟ Awesome T-Shirts! Sponsors! Books! ☟☟
T-SHIRTS AVAILABLE!
WANT MORE JASON?
SUPPORT THE CHANNEL
GET INVOLVED
JASON'S BOOKS
► C++23 Best Practices
► C++ Best Practices
JASON'S PUZZLE BOOKS
► Object Lifetime Puzzlers Book 1
► Object Lifetime Puzzlers Book 2
► Object Lifetime Puzzlers Book 3
► Copy and Reference Puzzlers Book 1
► Copy and Reference Puzzlers Book 2
► Copy and Reference Puzzlers Book 3
► OpCode Puzzlers Book 1
RECOMMENDED BOOKS
AWESOME PROJECTS
O'Reilly VIDEOS
Source:
T-SHIRTS AVAILABLE!
WANT MORE JASON?
SUPPORT THE CHANNEL
GET INVOLVED
JASON'S BOOKS
► C++23 Best Practices
► C++ Best Practices
JASON'S PUZZLE BOOKS
► Object Lifetime Puzzlers Book 1
► Object Lifetime Puzzlers Book 2
► Object Lifetime Puzzlers Book 3
► Copy and Reference Puzzlers Book 1
► Copy and Reference Puzzlers Book 2
► Copy and Reference Puzzlers Book 3
► OpCode Puzzlers Book 1
RECOMMENDED BOOKS
AWESOME PROJECTS
O'Reilly VIDEOS
Source:
Massive Cool Tech Unboxing - JAN 2025 - EP#97 (Must See Tech Haul)
Lavender Fields | Episode 97 (1/2) | January 14, 2025 (w/ English Subs)
Mehek - EPS 97 - Zindagi Ki Mehek - Version Française - Complet - HD
The CRICKETher Weekly - Episode 97
Mehek - EP 97 - Zindagi Ki Mehek Shaurya utilise Nikita pour rendre Mehek jalouse, Mehek le découvre...
Mehek - EPS 98 - Zindagi Ki Mehek - Version Française - Complet - HD
Mehek - EP 98 - Zindagi Ki Mehek || Shaurya soudoya Mohite pour saboter Mehek lors de la finale!
Le Triomphe de l'Amour - Episode 97 - Novelas Complète En Francais
Le Triomphe de l'amour épisode 96-97 | un témoin importante apparaît dans l'enquête du meu...
Episode 97 - Important Financial Metrics for Creative Agencies with David C. Baker
Sales-Commision Calculator (Exercise) | Ep. 97 | C Programming Language
SARFAROSH | Ep97 | Wife Of Jewish Man Saved Jasoos From Police | Roxen Original
MMU - EP 97 | Why you need a plan A, B & C
Let's Play Minecraft: Ep. 97 - Title Update 14 Part 1
Should I Open it? Or Should I Keep it Sealed? - Episode 97 - EX Dragon Frontier #pokemontcg
Mehek - EP 99 - Zindagi Ki Mehek || Mehek découvre la trahison de Mohite, la famille est choquée !...
Lavender Fields | Episode 96 (1/2) | January 13, 2025 (w/ English Subs)
Ton coeur, mon bonheur (Nokshi Kantha)- EP 97 - Complet en français - HD
Vitto Accidentally Shoots Iris | Lavender Fields | Netflix Philippines
Weekly ReLIV - Ali Baba - Dastaan-e-Kabul - Episodes 97 To 102 | 12 December To 17 December 2022
le sud c'est la chienneté episode 97/ abonne toi la chienneté
Krishna Ka Uddeshya | Yashomati Maiyaa Ke Nandlala - Ep 97 | Full Episode | 20 Oct 2022
Full Episode - 97 || May I Come In Madam || Kashmira se pyaar, Sanjana se shaadi! #starbharat
Ep. #97 Female History Making Trailblazers - Jewel Thais Williams & Director C.Fitz
Комментарии