filmov
tv
How to Read JSON File in Qt C++: A Beginner’s Guide
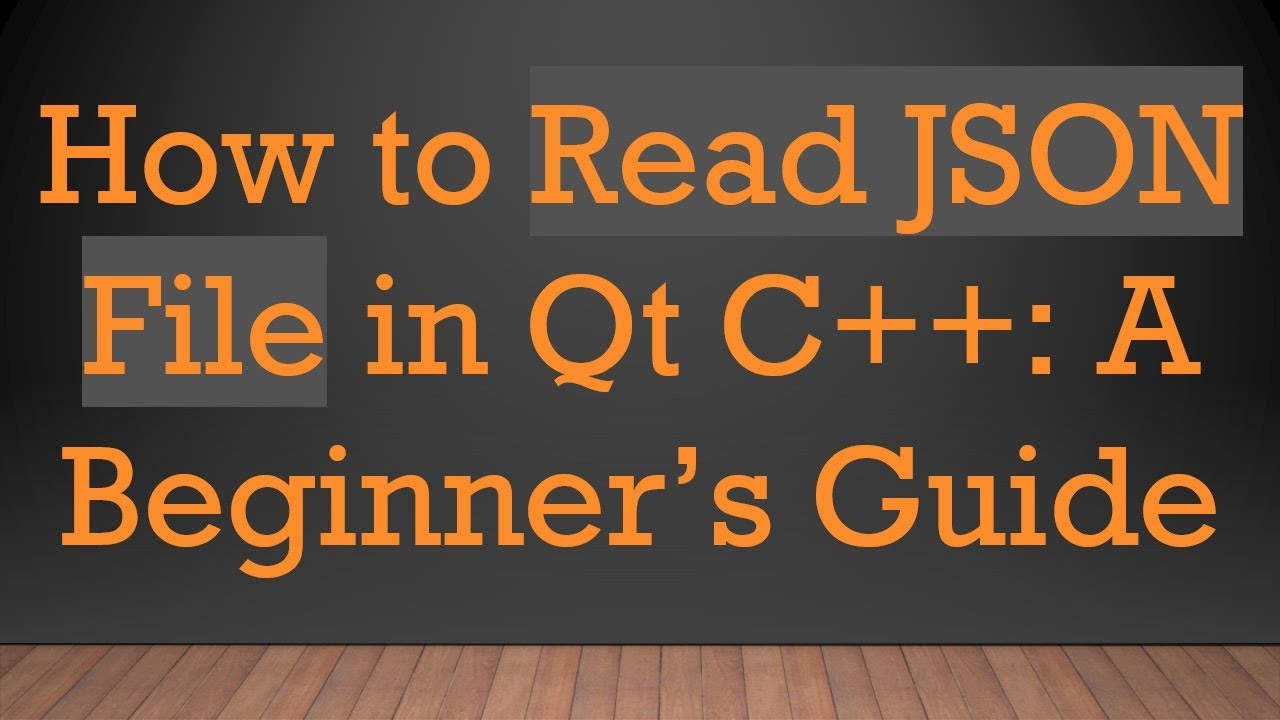
Показать описание
Discover how to effectively read and parse a JSON file in Qt C++ in this beginner-friendly guide, complete with practical examples and tips.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to read JSON file in Qt C++?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Read JSON File in Qt C++: A Beginner’s Guide
If you’re delving into the world of Qt C++, you may find yourself needing to read and parse JSON files for your application. JSON (JavaScript Object Notation) is a lightweight data interchange format that's easy for humans to read and write and for machines to parse and generate. In this guide, we’ll walk through how to read a JSON file and display its contents using Qt, which can help enhance your application’s functionality.
Understanding the Problem
[[See Video to Reveal this Text or Code Snippet]]
In your application, you wish to read this JSON file and display its contents in various labels upon the button click event. Let’s break down how to accomplish this in a step-by-step manner.
Step-by-Step Solution
1. Opening the JSON File
First and foremost, you need to open the JSON file for reading. Here's how you do it in Qt:
[[See Video to Reveal this Text or Code Snippet]]
This code attempts to open the file in read-only mode. If it fails, it outputs an error message.
2. Parsing the JSON Document
Once the file is opened successfully, you can read its contents into a QJsonDocument:
[[See Video to Reveal this Text or Code Snippet]]
This reads the entire file content and converts it to a QJsonDocument object which will allow us to access the data inside.
3. Navigating the JSON Structure
The key aspect here is that your JSON file has a root object with a key called userList, which is an array. You will need to access this array to display user details:
[[See Video to Reveal this Text or Code Snippet]]
This code extracts the userList, converts it to an array, and accesses the first user's details.
4. Extracting User Data
Now that you have the user's data in a QJsonObject, you can extract the required fields as shown below:
[[See Video to Reveal this Text or Code Snippet]]
This allows you to pull out specific values from the user object.
5. Displaying the Data in Labels
Finally, you can set the text of your labels using the extracted values:
[[See Video to Reveal this Text or Code Snippet]]
This will update your UI elements with the user information retrieved from the JSON file.
Important Considerations
JSON Format: When dealing with JSON, ensure that it adheres to the correct format. Your initial JSON had an extra comma at the end of the user list, which is not allowed per the JSON specification. Make sure to correct this before parsing.
Conclusion
Reading a JSON file in Qt C++ can seem daunting at first, but by following this structured approach, you can easily parse the data and display it in your application. Remember, practice makes perfect! Continue to experiment with more complex JSON structures as you grow your skills in Qt. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to read JSON file in Qt C++?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Read JSON File in Qt C++: A Beginner’s Guide
If you’re delving into the world of Qt C++, you may find yourself needing to read and parse JSON files for your application. JSON (JavaScript Object Notation) is a lightweight data interchange format that's easy for humans to read and write and for machines to parse and generate. In this guide, we’ll walk through how to read a JSON file and display its contents using Qt, which can help enhance your application’s functionality.
Understanding the Problem
[[See Video to Reveal this Text or Code Snippet]]
In your application, you wish to read this JSON file and display its contents in various labels upon the button click event. Let’s break down how to accomplish this in a step-by-step manner.
Step-by-Step Solution
1. Opening the JSON File
First and foremost, you need to open the JSON file for reading. Here's how you do it in Qt:
[[See Video to Reveal this Text or Code Snippet]]
This code attempts to open the file in read-only mode. If it fails, it outputs an error message.
2. Parsing the JSON Document
Once the file is opened successfully, you can read its contents into a QJsonDocument:
[[See Video to Reveal this Text or Code Snippet]]
This reads the entire file content and converts it to a QJsonDocument object which will allow us to access the data inside.
3. Navigating the JSON Structure
The key aspect here is that your JSON file has a root object with a key called userList, which is an array. You will need to access this array to display user details:
[[See Video to Reveal this Text or Code Snippet]]
This code extracts the userList, converts it to an array, and accesses the first user's details.
4. Extracting User Data
Now that you have the user's data in a QJsonObject, you can extract the required fields as shown below:
[[See Video to Reveal this Text or Code Snippet]]
This allows you to pull out specific values from the user object.
5. Displaying the Data in Labels
Finally, you can set the text of your labels using the extracted values:
[[See Video to Reveal this Text or Code Snippet]]
This will update your UI elements with the user information retrieved from the JSON file.
Important Considerations
JSON Format: When dealing with JSON, ensure that it adheres to the correct format. Your initial JSON had an extra comma at the end of the user list, which is not allowed per the JSON specification. Make sure to correct this before parsing.
Conclusion
Reading a JSON file in Qt C++ can seem daunting at first, but by following this structured approach, you can easily parse the data and display it in your application. Remember, practice makes perfect! Continue to experiment with more complex JSON structures as you grow your skills in Qt. Happy coding!