filmov
tv
Creating a Tuple in Python: A Simple Function to Sort Numbers and Return a Sum
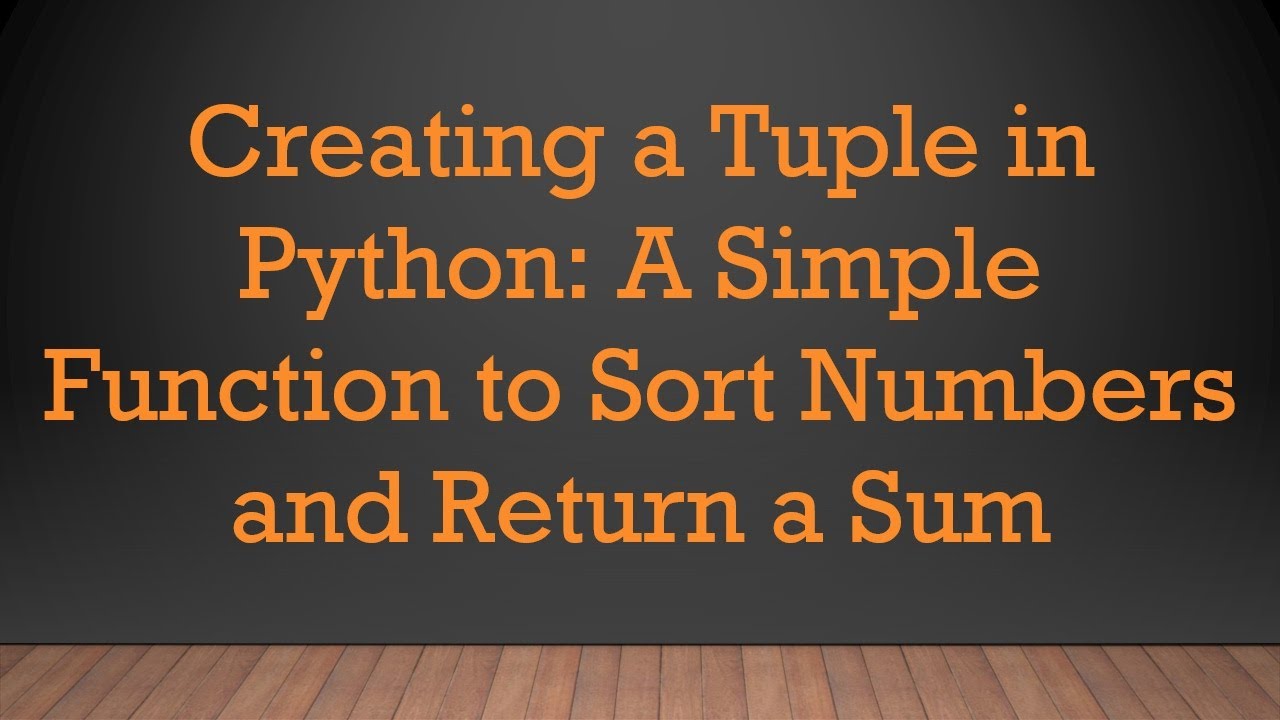
Показать описание
Discover how to create a Python function that takes three numbers and returns a tuple with the smallest, largest, and the sum of the numbers in a well-structured format.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Tuple function that returns certain parameters
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Creating a Tuple in Python: A Simple Function to Sort Numbers and Return a Sum
Have you ever found yourself stuck on a programming exercise that seems straightforward but leaves you scratching your head? If you’re learning Python and want to create a function that returns a tuple based on certain criteria, you’re in the right place. In this guide, we’ll walk through a common problem involving tuples and collaborate to formulate an elegant solution.
The Problem
You need to write a function in Python that receives three numbers as inputs and returns a tuple that meets specific rules:
The first element must be the smallest number.
The second element must be the largest number.
The third element is the sum of all three numbers.
Here’s an example of how the function should work:
[[See Video to Reveal this Text or Code Snippet]]
As you can see, from the numbers 5, 3, and -1, the smallest is -1, the largest is 5, and their total sum is 7. Now, let’s dive into the solution for achieving this.
The Solution
To write the function, we will follow these steps:
Accept input parameters - The function will take three integer arguments.
Create a tuple - Compile the input numbers into a tuple.
Find the minimum and maximum values - Utilize Python’s built-in min() and max() functions.
Calculate the sum - Add the three parameters to get their total.
Return the tuple - Structure and return the final tuple in the required format.
Here’s how the code should look:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Code
Function Definition: def do_tuple(x: int, y: int, z: int) allows us to define a function that takes three integers as input.
Tuple Creation: tuple_ = (x, y, z) gathers the three numbers into a tuple structure.
Sum Calculation: We then calculate the sum of the three inputs with summ = x + y + z.
Finding Extremes: By using mini = min(tuple_) and maxi = max(tuple_), you can find the smallest and largest inputs easily.
Return Statement: The return (mini, maxi, summ) returns the desired tuple with sorted values and the total.
Conclusion
Python’s flexibility allows for simple solutions to what might initially seem complex. By following the structured steps above, you can effectively manage inputs and outputs through tuples. With practice, you’ll find that writing these functions becomes second nature.
Give this function a try with different sets of numbers and see how it works! The key takeaway is understanding how to manipulate data structures like tuples to fit specific needs. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Tuple function that returns certain parameters
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Creating a Tuple in Python: A Simple Function to Sort Numbers and Return a Sum
Have you ever found yourself stuck on a programming exercise that seems straightforward but leaves you scratching your head? If you’re learning Python and want to create a function that returns a tuple based on certain criteria, you’re in the right place. In this guide, we’ll walk through a common problem involving tuples and collaborate to formulate an elegant solution.
The Problem
You need to write a function in Python that receives three numbers as inputs and returns a tuple that meets specific rules:
The first element must be the smallest number.
The second element must be the largest number.
The third element is the sum of all three numbers.
Here’s an example of how the function should work:
[[See Video to Reveal this Text or Code Snippet]]
As you can see, from the numbers 5, 3, and -1, the smallest is -1, the largest is 5, and their total sum is 7. Now, let’s dive into the solution for achieving this.
The Solution
To write the function, we will follow these steps:
Accept input parameters - The function will take three integer arguments.
Create a tuple - Compile the input numbers into a tuple.
Find the minimum and maximum values - Utilize Python’s built-in min() and max() functions.
Calculate the sum - Add the three parameters to get their total.
Return the tuple - Structure and return the final tuple in the required format.
Here’s how the code should look:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Code
Function Definition: def do_tuple(x: int, y: int, z: int) allows us to define a function that takes three integers as input.
Tuple Creation: tuple_ = (x, y, z) gathers the three numbers into a tuple structure.
Sum Calculation: We then calculate the sum of the three inputs with summ = x + y + z.
Finding Extremes: By using mini = min(tuple_) and maxi = max(tuple_), you can find the smallest and largest inputs easily.
Return Statement: The return (mini, maxi, summ) returns the desired tuple with sorted values and the total.
Conclusion
Python’s flexibility allows for simple solutions to what might initially seem complex. By following the structured steps above, you can effectively manage inputs and outputs through tuples. With practice, you’ll find that writing these functions becomes second nature.
Give this function a try with different sets of numbers and see how it works! The key takeaway is understanding how to manipulate data structures like tuples to fit specific needs. Happy coding!