filmov
tv
How to Set State to a Specific Type in React with TypeScript
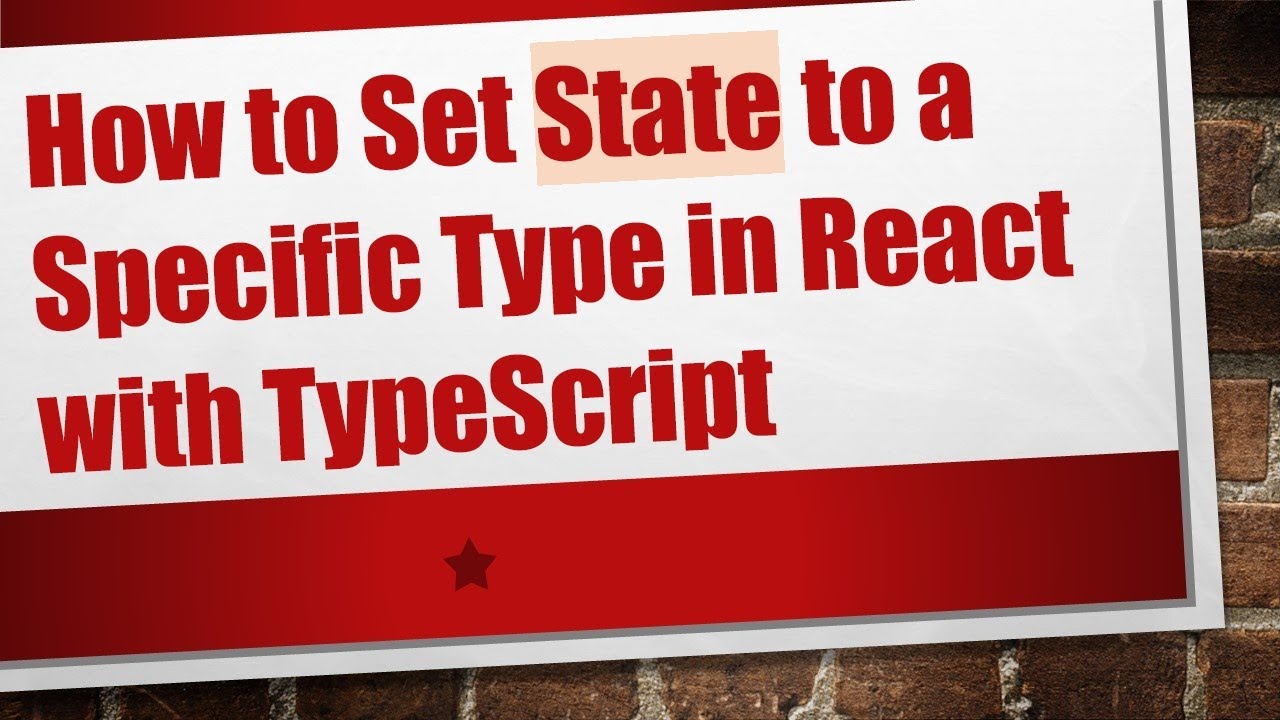
Показать описание
Learn how to effectively use `readonly` and set state to a specific type in React with TypeScript for better type safety and clarity in your components.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to readonly setState to a specific type?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding how to setState to a Specific Type in React with TypeScript
When working with React and TypeScript, you often face challenges related to typing states accurately. This becomes especially evident when typing state variables using hooks like useState. A common question is: How do I set state to a specific type while ensuring it remains readonly?
Let's explore this issue and provide a clear solution.
The Problem: Setting State and Type Compatibility
You may start with a state definition like this:
[[See Video to Reveal this Text or Code Snippet]]
[[See Video to Reveal this Text or Code Snippet]]
This indicates a mismatch in expected types when attempting to set the state. Specifically, it reveals that the type being assigned doesn't match the expected type of the state variable.
Analyzing the Types
The type defined for Parameters is intricate:
[[See Video to Reveal this Text or Code Snippet]]
The Solution: Correctly Defining the Types
Step 1: Adjust the State Declaration
The immediate solution is to redefine your state to make sure it aligns with what you want to store. Instead of using Parameters<any>[] | undefined, you should change your state definition to a more appropriate type that matches what you plan to assign.
Here’s the revision you should make to your useState declaration:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Ensuring Correct Data Types
Result Verification
After this adjustment, your component should work without throwing type errors. You can now safely call:
[[See Video to Reveal this Text or Code Snippet]]
This ensures that paramList only receives data that fits within your defined constraints.
Conclusion
Setting state to a specific type with TypeScript in React requires careful attention to type definitions and data structures. By redefining your state and ensuring type compliance, you can avoid frustrating type errors and create robust, type-safe components.
With these steps and the adjustments outlined, you’ll be able to effectively manage your state in React components using TypeScript, enhancing both clarity and maintainability in your code.
Now that you have a clear understanding, give it a try in your projects!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to readonly setState to a specific type?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding how to setState to a Specific Type in React with TypeScript
When working with React and TypeScript, you often face challenges related to typing states accurately. This becomes especially evident when typing state variables using hooks like useState. A common question is: How do I set state to a specific type while ensuring it remains readonly?
Let's explore this issue and provide a clear solution.
The Problem: Setting State and Type Compatibility
You may start with a state definition like this:
[[See Video to Reveal this Text or Code Snippet]]
[[See Video to Reveal this Text or Code Snippet]]
This indicates a mismatch in expected types when attempting to set the state. Specifically, it reveals that the type being assigned doesn't match the expected type of the state variable.
Analyzing the Types
The type defined for Parameters is intricate:
[[See Video to Reveal this Text or Code Snippet]]
The Solution: Correctly Defining the Types
Step 1: Adjust the State Declaration
The immediate solution is to redefine your state to make sure it aligns with what you want to store. Instead of using Parameters<any>[] | undefined, you should change your state definition to a more appropriate type that matches what you plan to assign.
Here’s the revision you should make to your useState declaration:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Ensuring Correct Data Types
Result Verification
After this adjustment, your component should work without throwing type errors. You can now safely call:
[[See Video to Reveal this Text or Code Snippet]]
This ensures that paramList only receives data that fits within your defined constraints.
Conclusion
Setting state to a specific type with TypeScript in React requires careful attention to type definitions and data structures. By redefining your state and ensuring type compliance, you can avoid frustrating type errors and create robust, type-safe components.
With these steps and the adjustments outlined, you’ll be able to effectively manage your state in React components using TypeScript, enhancing both clarity and maintainability in your code.
Now that you have a clear understanding, give it a try in your projects!