filmov
tv
C implementation of Binary Search Tree
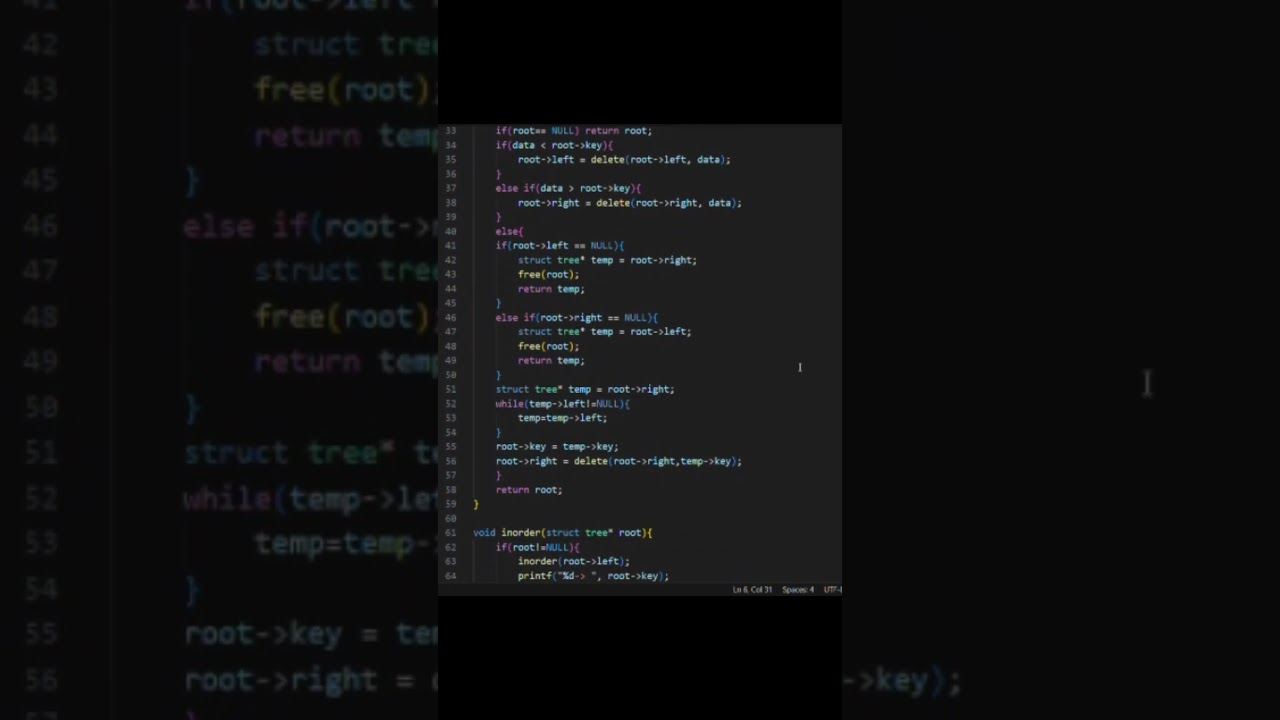
Показать описание
Music credits-
Music : Another time
Musician : LiQWYD
For more related content, visit channel.
Music : Another time
Musician : LiQWYD
For more related content, visit channel.
C Program to implement Binary Search || Coding Guide for Beginners|| #programming
Binary Search Algorithm | C Programming Example
Binary Search Algorithm in 100 Seconds
Binary Search | Logical Programming in C | by Mr.Srinivas
Binary search tree - Implementation in C/C++
7.2 What is Binary Search | Binary Search Algorithm with example | Data Structures Tutorials
Learn Binary Search in 10 minutes 🪓
Binary Search Algorithm - Simply Explained
10- Data Structures & Algorithms (CS505) Session 5
C++ Programming: Binary Search Algorithm
Binary Search examples | Successful Search | DAA | Lec-13 | Bhanu Priya
2.6.2 Binary Search Recursive Method
Program of Binary Search Tree in C Language
Understand and Implement a Binary Search Tree in C
Linear search vs Binary search
Binary Search - Recursive implementation
That's How Binary Search Works For Finding Friends!!! :)
Linear Vs Binary Search + Code in C Language (With Notes)
Binary Search - Iterative Implementation and common errors
Lec-15: Binary Search in Data Structure by #Naina Mam
Find an Element Using BINARY SEARCH in C | C Programming
How to Do a Binary Search in C# (Simple)
How to invert a binary tree - in 20 seconds
8.2 Searching in Arrays | Linear and Binary Search | C++ Placement Course |
Комментарии