filmov
tv
Edit Distance Between 2 Strings - The Levenshtein Distance ('Edit Distance' on LeetCode)
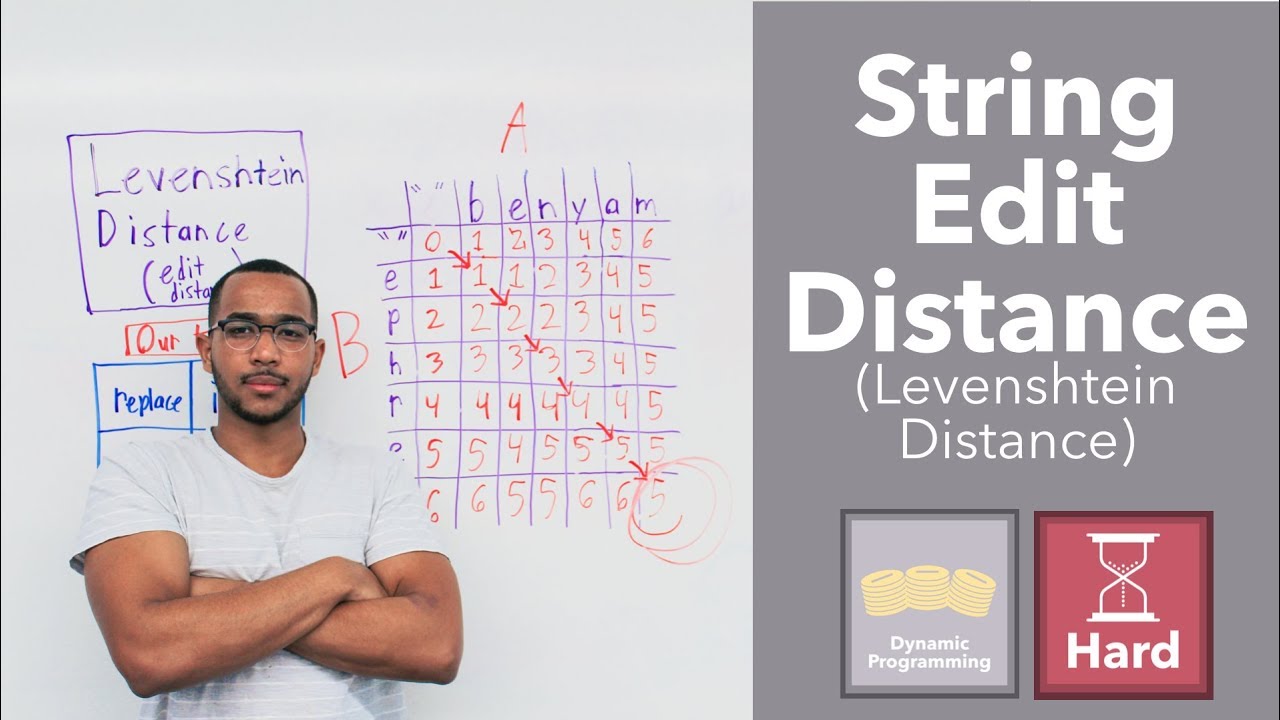
Показать описание
📹 Intuitive Video Explanations
🏃 Run Code As You Learn
💾 Save Progress
❓New Unseen Questions
🔎 Get All Solutions
Question: Write a program that takes two strings and computes the minimum number of edits needed to transform the first string into the second string.
Examples:
Input: "Saturday" and "Sundays"
Output: 4
Why:
In: "Saturday"
1.) Delete the first 'a' ("Sturday")
2.) Delete the first 't' ("Surday")
3.) Replace 'r' with 'n' ("Sunday")
4.) Insert an 's' at the end ("Sundays")
Out: "Sundays"
Our 3 Operations To Fix Character Mismatch:
- Insert
- Delete
- Replacement
++++++++++++++++++++++++++++++++++++++++++++++++++
++++++++++++++++++++++++++++++++++++++++++++++++++
This question is number 17.2 in "Elements of Programming Interviews" by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash.
🏃 Run Code As You Learn
💾 Save Progress
❓New Unseen Questions
🔎 Get All Solutions
Question: Write a program that takes two strings and computes the minimum number of edits needed to transform the first string into the second string.
Examples:
Input: "Saturday" and "Sundays"
Output: 4
Why:
In: "Saturday"
1.) Delete the first 'a' ("Sturday")
2.) Delete the first 't' ("Surday")
3.) Replace 'r' with 'n' ("Sunday")
4.) Insert an 's' at the end ("Sundays")
Out: "Sundays"
Our 3 Operations To Fix Character Mismatch:
- Insert
- Delete
- Replacement
++++++++++++++++++++++++++++++++++++++++++++++++++
++++++++++++++++++++++++++++++++++++++++++++++++++
This question is number 17.2 in "Elements of Programming Interviews" by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash.
Комментарии