filmov
tv
How to Fix Your JavaScript Function Not Getting Called in Conditional Statements
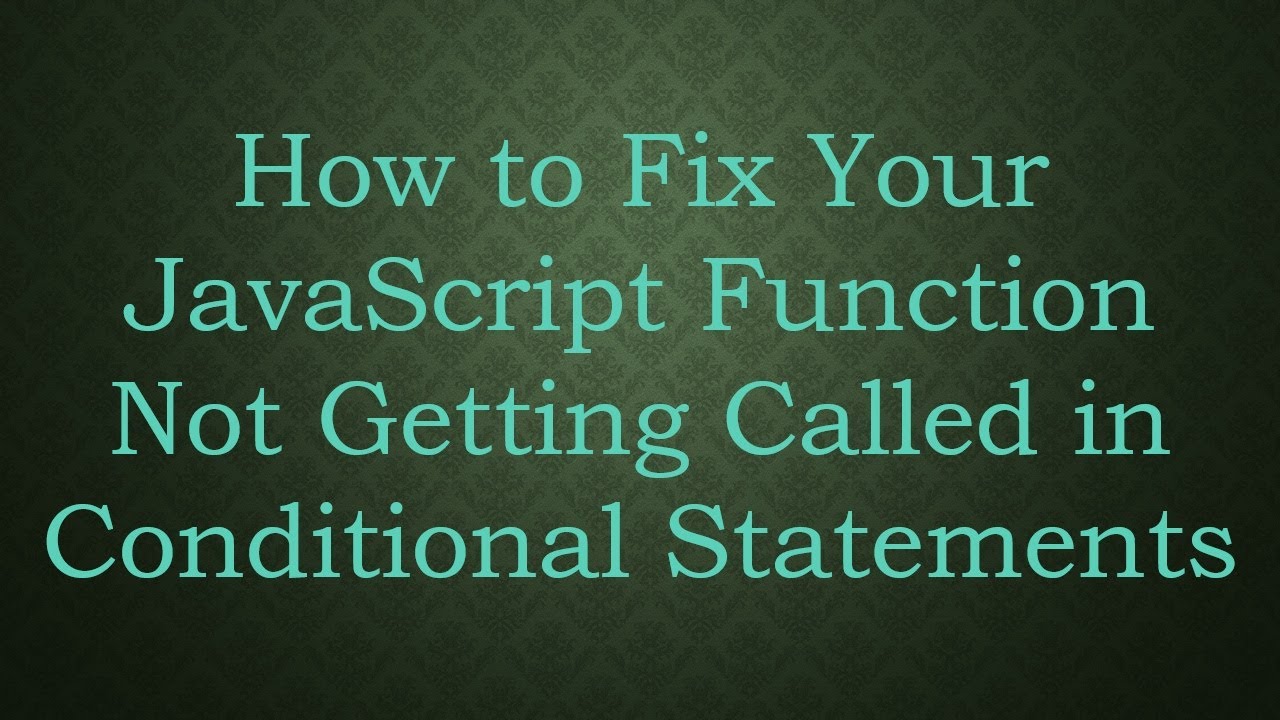
Показать описание
Learn how to troubleshoot and fix issues with JavaScript functions not executing in if conditions. Simple steps to ensure your code runs as expected!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: A function inside javascript if condition is not getting called
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Troubleshooting JavaScript Function Not Getting Called in Conditionals
If you’re new to JavaScript and programming, encountering issues where your functions don’t seem to execute as expected can be frustrating. This problem often arises due to missed steps or misunderstandings about how JavaScript works. In this guide, we’ll explore a common scenario where a JavaScript function defined within an if condition is not called properly, and how to fix it.
The Problem
In the provided code snippet, a function named myInterval3 is created within an if condition. You may notice that while this function is defined, it never actually runs. The code flows through the if statement without executing myInterval3. Here’s a simplified look at the relevant part of the code for context:
[[See Video to Reveal this Text or Code Snippet]]
What’s happening here? Let's break it down.
Understanding Function Definitions
In JavaScript, defining a function does not automatically execute it. For instance, the line:
[[See Video to Reveal this Text or Code Snippet]]
only creates the function. To run the code inside this function, you need to call it explicitly. This is typically done by writing the function name followed by parentheses:
[[See Video to Reveal this Text or Code Snippet]]
How to Call the Function Correctly
To ensure that myInterval3 runs under the right conditions, here are steps you should follow:
1. Define the Function
Continue to define your function as you have already done. Just ensure you know that this alone won’t execute it.
2. Call the Function
To have this function execute when the condition rightPos is met, you will need to add the function call after the function definition, like this:
[[See Video to Reveal this Text or Code Snippet]]
3. Use setInterval for Repeated Execution
If you want myInterval3 to execute periodically (like your other functions that move the object), wrap the function call inside setInterval. This way, it will run repeatedly at set intervals:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By understanding how to properly define and invoke functions in JavaScript, you can avoid common pitfalls that lead to functions not being called. Remember that defining a function is just the first step; you must also call it whenever you want its code to execute. Following the guidelines discussed in this post will enhance your programming skills and confidence in working with JavaScript.
Now that you know how to fix the problem of a function not executing in an if condition, go ahead and test it in your code! Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: A function inside javascript if condition is not getting called
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Troubleshooting JavaScript Function Not Getting Called in Conditionals
If you’re new to JavaScript and programming, encountering issues where your functions don’t seem to execute as expected can be frustrating. This problem often arises due to missed steps or misunderstandings about how JavaScript works. In this guide, we’ll explore a common scenario where a JavaScript function defined within an if condition is not called properly, and how to fix it.
The Problem
In the provided code snippet, a function named myInterval3 is created within an if condition. You may notice that while this function is defined, it never actually runs. The code flows through the if statement without executing myInterval3. Here’s a simplified look at the relevant part of the code for context:
[[See Video to Reveal this Text or Code Snippet]]
What’s happening here? Let's break it down.
Understanding Function Definitions
In JavaScript, defining a function does not automatically execute it. For instance, the line:
[[See Video to Reveal this Text or Code Snippet]]
only creates the function. To run the code inside this function, you need to call it explicitly. This is typically done by writing the function name followed by parentheses:
[[See Video to Reveal this Text or Code Snippet]]
How to Call the Function Correctly
To ensure that myInterval3 runs under the right conditions, here are steps you should follow:
1. Define the Function
Continue to define your function as you have already done. Just ensure you know that this alone won’t execute it.
2. Call the Function
To have this function execute when the condition rightPos is met, you will need to add the function call after the function definition, like this:
[[See Video to Reveal this Text or Code Snippet]]
3. Use setInterval for Repeated Execution
If you want myInterval3 to execute periodically (like your other functions that move the object), wrap the function call inside setInterval. This way, it will run repeatedly at set intervals:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By understanding how to properly define and invoke functions in JavaScript, you can avoid common pitfalls that lead to functions not being called. Remember that defining a function is just the first step; you must also call it whenever you want its code to execute. Following the guidelines discussed in this post will enhance your programming skills and confidence in working with JavaScript.
Now that you know how to fix the problem of a function not executing in an if condition, go ahead and test it in your code! Happy coding!