filmov
tv
Python Tutorial - How to make Text-Based Tables
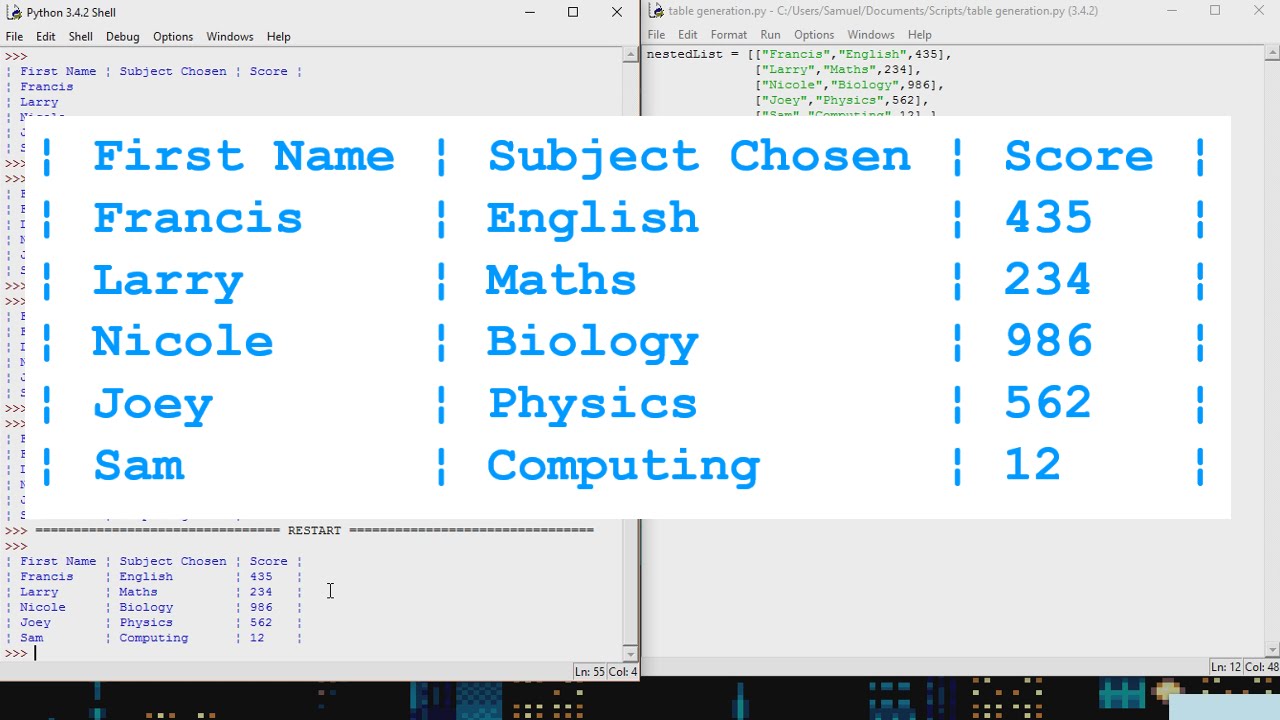
Показать описание
(See below for better code)
A note from 2022: ok so notice how I'm 15 in this video, and how i'm clearly not taking the video seriously; these are indicators that the code is going to be low quality too;)
= Why is it bad code? =
- The header row is hard-coded (i.e. there's one string that represents the whole header row, rather than one string for each column name)
- The lengths of each header row is hard coded (e.g. the numbers 9, 13, 4 in the big print line). This is bad because if we ever need to change the column names, we'll also need to update these, and the relationship between 'Subject Chosen' and 13 is a bit unclear.
- The number of columns themselves is hard-coded. Better code would be able to handle more columns automatically.
- The table just looks a bit ugly...?
- Also why are the entries left-aligned instead of right-aligned?! that looks silly for the column with numbers in.
- It's not in a function, so it can't be reused.
- Using camel case for variable names (i.e. namingYourVariablesLikeThis) is a bit ugly too...
= Updated code =
def print_table(nested_list, column_names):
num_cols = len(column_names)
header_row = (
'| '
+ ' | '.join(column_names)
+ ' |'
)
nice_horizontal_rule = ('|'+'-' * (len(header_row)-2)+'|')
print(nice_horizontal_rule)
print(header_row)
print(nice_horizontal_rule)
for item in nested_list:
# writing each row to a string,
# then printing the string, is better for performance:)
s = "|"
for i in range(num_cols):
entry = str(item[i])
s += (" "*(len(column_names[i]) - len(entry)+2) +
entry + "|")
print(s)
print(nice_horizontal_rule)
my_nested_list = [
["Francis", "English", 435],
["Larry","Maths", 234],
["Nicole", "Biology", 986],
["Joey", "Physics", 562],
["Sam", "Computing", 12],
]
my_column_names = ["First Name", "Subject Chosen", "Score"]
print_table(my_nested_list, my_column_names)
A note from 2022: ok so notice how I'm 15 in this video, and how i'm clearly not taking the video seriously; these are indicators that the code is going to be low quality too;)
= Why is it bad code? =
- The header row is hard-coded (i.e. there's one string that represents the whole header row, rather than one string for each column name)
- The lengths of each header row is hard coded (e.g. the numbers 9, 13, 4 in the big print line). This is bad because if we ever need to change the column names, we'll also need to update these, and the relationship between 'Subject Chosen' and 13 is a bit unclear.
- The number of columns themselves is hard-coded. Better code would be able to handle more columns automatically.
- The table just looks a bit ugly...?
- Also why are the entries left-aligned instead of right-aligned?! that looks silly for the column with numbers in.
- It's not in a function, so it can't be reused.
- Using camel case for variable names (i.e. namingYourVariablesLikeThis) is a bit ugly too...
= Updated code =
def print_table(nested_list, column_names):
num_cols = len(column_names)
header_row = (
'| '
+ ' | '.join(column_names)
+ ' |'
)
nice_horizontal_rule = ('|'+'-' * (len(header_row)-2)+'|')
print(nice_horizontal_rule)
print(header_row)
print(nice_horizontal_rule)
for item in nested_list:
# writing each row to a string,
# then printing the string, is better for performance:)
s = "|"
for i in range(num_cols):
entry = str(item[i])
s += (" "*(len(column_names[i]) - len(entry)+2) +
entry + "|")
print(s)
print(nice_horizontal_rule)
my_nested_list = [
["Francis", "English", 435],
["Larry","Maths", 234],
["Nicole", "Biology", 986],
["Joey", "Physics", 562],
["Sam", "Computing", 12],
]
my_column_names = ["First Name", "Subject Chosen", "Score"]
print_table(my_nested_list, my_column_names)
Комментарии