filmov
tv
C++Tutorial for Beginners 69 Creating an Explosion
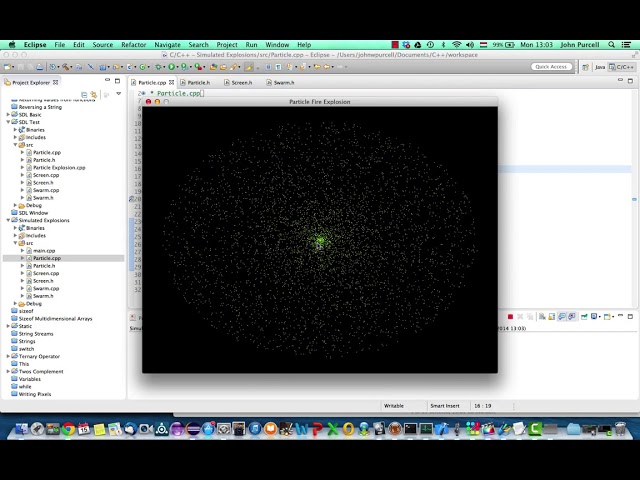
Показать описание
C++ is a multi-paradigm programming language that supports object-oriented programming (OOP), created by Bjarne Stroustrup in 1983 at Bell Labs, C++ is an extension(superset) of C programming and the programs are written in C language can run in C++ compilers.
C++ is used by programmers to create computer software. It is used to create general systems software, drivers for various computer devices, software for servers and software for specific applications and also widely used in the creation of video games.
C++ is used by many programmers of different types and coming from different fields. C++ is mostly used to write device driver programs, system software, and applications that depend on direct hardware manipulation under real-time constraints. It is also used to teach the basics of object-oriented features because it is simple and is also used in the fields of research. Also, many primary user interfaces and system files of Windows and Macintosh are written using C++. So, C++ is really a popular, strong and frequently used programming language of this modern programming era.
The main focus remains on data rather than procedures.
Object-oriented programs are segmented into parts called objects.
Data structures are designed to categorize the objects.
Data member and functions are tied together as a data structure.
Data can be hidden and cannot be accessed by external functions using access specifier.
Objects can communicate among themselves using functions.
New data and functions can be easily added anywhere within a program whenever required.
Since this is an object-oriented programming language, it follows a bottom up approach, i.e. the execution of codes starts from the main which resides at the lower section and then based on the member function call the working is done from the classes.
The object-oriented approach is a recent concept among programming paradigms and has various fields of progress. Object-oriented programming is a technique that provides a way of modularizing programs by creating memory area as a partition for both data and functions that can further be used as a template to create copies of modules on demand.
1 - Introduction
2 - Installation
3 - Hello World
4 - Outputting Text
5 - Variables
6 - Strings
7 - User Input
8 - Binary Numbers and Memory
9 - Integer Variable Types
10 - Floating Point Variable Types
11 - Char and Bool
12 - The "If" Statement
13 - If-Else
14 - Choosing Between Alternatives: If-ElseIf-Else
15 - Complex Conditions
16 - "While" Loops
17 - The Do-While Loops
18 - "For" Loops
19 - Break and Continue
20 - Arrays
21 - Multidimensional Arrays
22 - Size of and Arrays
23 - Sizeof Multidimensional Arrays
24 - Switch: Choosing Between Alternatives
25 - Functions: Using Subroutines in C++
26 - Return Values: Getting Data From Subroutines
27 - Function Parameters: Passing Data to Subroutines
28 - Headers and Prototypes
29 - Classes: The Foundation of Object-Oriented (OO) Programming
30 - Data Members, a.k.a. Instance Variables
31 - Constructors and Destructors
32 - Getters and Setters
33 - String Streams; Adding Number to Strings
34 - Overloading Constructors
35 - The "this" Keyword; A First Taste of Pointers
36 - Constructor Initialization Lists
37 - Pointers; Where C++ Starts to Get Tricky
38 - Arithmetic Operators
39 - Pointers and Arrays
40 - Pointer Arithmetic; Adding, Subtracting and Comparing Pointers
41 - Char Arrays: Primitive Strings in C++
42 - Reversing a String (Interview Question!)
43 - References; Nicer than Pointers!
44 - Const; a Vital Tool for Reducing Bugs
45 - Copy Constructors; Creating Copies of Objects
46 - The New Operator; Allocating Memory in C++
47 - Returning Objects from Functions
48 - Allocating Memory
49 - Arrays and Functions
50 - Namespaces; Organise Your Classes
51 - Inheritance
52 - Encapsulation
53 - Constructor Inheritance
54 - Twos Complement
55 - Static Variables
56 - Particle Fire Explosion
57 - Using C++ Libraries
58 - Aquiring Simple Direct Media Layer
59 - A Basic SDL Program
60 - Creating an SDL Window
61 - Textures, Renderers and Buffers
62 - Setting Pixel Colors
63 - Creating the Screen Class
64 - Bit Shifting and Colors
65 - Adding a Set Pixel Method
66 - Animating Colors
67 - Creating Particles (Starfields!)
68 - Animating Particles
69 - Creating an Explosion
70 - Ensuring Constant Speed
71 - Biwise "And"
72 - Implementing Box Blur
73 - Realistic Particle Motion
74 - Languages Overview
75 - What Next
76 - Object Oriented Design Considerations
77 - Understanding Postfix and Prefix
78 - Static Creating Libraries
C++ is used by programmers to create computer software. It is used to create general systems software, drivers for various computer devices, software for servers and software for specific applications and also widely used in the creation of video games.
C++ is used by many programmers of different types and coming from different fields. C++ is mostly used to write device driver programs, system software, and applications that depend on direct hardware manipulation under real-time constraints. It is also used to teach the basics of object-oriented features because it is simple and is also used in the fields of research. Also, many primary user interfaces and system files of Windows and Macintosh are written using C++. So, C++ is really a popular, strong and frequently used programming language of this modern programming era.
The main focus remains on data rather than procedures.
Object-oriented programs are segmented into parts called objects.
Data structures are designed to categorize the objects.
Data member and functions are tied together as a data structure.
Data can be hidden and cannot be accessed by external functions using access specifier.
Objects can communicate among themselves using functions.
New data and functions can be easily added anywhere within a program whenever required.
Since this is an object-oriented programming language, it follows a bottom up approach, i.e. the execution of codes starts from the main which resides at the lower section and then based on the member function call the working is done from the classes.
The object-oriented approach is a recent concept among programming paradigms and has various fields of progress. Object-oriented programming is a technique that provides a way of modularizing programs by creating memory area as a partition for both data and functions that can further be used as a template to create copies of modules on demand.
1 - Introduction
2 - Installation
3 - Hello World
4 - Outputting Text
5 - Variables
6 - Strings
7 - User Input
8 - Binary Numbers and Memory
9 - Integer Variable Types
10 - Floating Point Variable Types
11 - Char and Bool
12 - The "If" Statement
13 - If-Else
14 - Choosing Between Alternatives: If-ElseIf-Else
15 - Complex Conditions
16 - "While" Loops
17 - The Do-While Loops
18 - "For" Loops
19 - Break and Continue
20 - Arrays
21 - Multidimensional Arrays
22 - Size of and Arrays
23 - Sizeof Multidimensional Arrays
24 - Switch: Choosing Between Alternatives
25 - Functions: Using Subroutines in C++
26 - Return Values: Getting Data From Subroutines
27 - Function Parameters: Passing Data to Subroutines
28 - Headers and Prototypes
29 - Classes: The Foundation of Object-Oriented (OO) Programming
30 - Data Members, a.k.a. Instance Variables
31 - Constructors and Destructors
32 - Getters and Setters
33 - String Streams; Adding Number to Strings
34 - Overloading Constructors
35 - The "this" Keyword; A First Taste of Pointers
36 - Constructor Initialization Lists
37 - Pointers; Where C++ Starts to Get Tricky
38 - Arithmetic Operators
39 - Pointers and Arrays
40 - Pointer Arithmetic; Adding, Subtracting and Comparing Pointers
41 - Char Arrays: Primitive Strings in C++
42 - Reversing a String (Interview Question!)
43 - References; Nicer than Pointers!
44 - Const; a Vital Tool for Reducing Bugs
45 - Copy Constructors; Creating Copies of Objects
46 - The New Operator; Allocating Memory in C++
47 - Returning Objects from Functions
48 - Allocating Memory
49 - Arrays and Functions
50 - Namespaces; Organise Your Classes
51 - Inheritance
52 - Encapsulation
53 - Constructor Inheritance
54 - Twos Complement
55 - Static Variables
56 - Particle Fire Explosion
57 - Using C++ Libraries
58 - Aquiring Simple Direct Media Layer
59 - A Basic SDL Program
60 - Creating an SDL Window
61 - Textures, Renderers and Buffers
62 - Setting Pixel Colors
63 - Creating the Screen Class
64 - Bit Shifting and Colors
65 - Adding a Set Pixel Method
66 - Animating Colors
67 - Creating Particles (Starfields!)
68 - Animating Particles
69 - Creating an Explosion
70 - Ensuring Constant Speed
71 - Biwise "And"
72 - Implementing Box Blur
73 - Realistic Particle Motion
74 - Languages Overview
75 - What Next
76 - Object Oriented Design Considerations
77 - Understanding Postfix and Prefix
78 - Static Creating Libraries