filmov
tv
How to Read XML Using XPath in Java
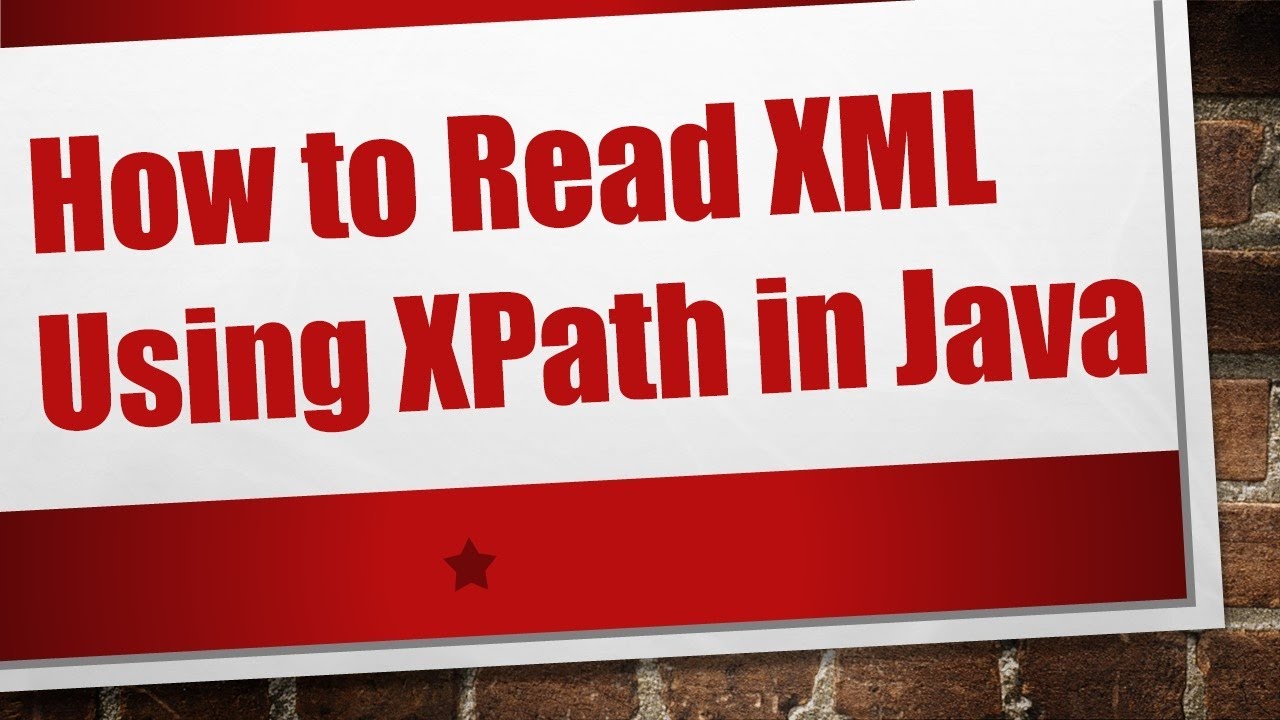
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn how to read XML files using XPath in Java, a powerful and efficient way to navigate through XML documents.
---
How to Read XML Using XPath in Java
XML (Extensible Markup Language) is widely used for storing and transporting data. If you're working with XML in Java, XPath is an excellent tool for navigating and querying XML documents. In this guide, we'll guide you through the process of reading XML files using XPath in Java, covering the essential steps and code snippets.
What is XPath?
XPath stands for XML Path Language. It is a query language that allows you to navigate through elements and attributes in an XML document. XPath provides a way to address parts of an XML document, making it easier to find specific data within the XML structure.
Setting Up the Environment
Before we dive into reading XML using XPath, let’s ensure we have the necessary setup:
Java Development Kit (JDK): Make sure you have the latest JDK installed.
XML File: Prepare an XML file that we will read using XPath.
[[See Video to Reveal this Text or Code Snippet]]
Reading XML Using XPath in Java
Step 1: Import Required Classes
To start, import the necessary classes from the Java API:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Parse the XML Document
Use DocumentBuilderFactory and DocumentBuilder to parse the XML file into a Document object:
[[See Video to Reveal this Text or Code Snippet]]
Explanation
Create a DocumentBuilderFactory: This factory is configured to return a parser that produces DOM objects from XML files.
Create a DocumentBuilder: This object parses the XML, returning a Document object.
Parse and Normalize: The parse method reads the XML file into the Document object, and normalize ensures the XML structure is in its canonical form.
Create XPath objects: Create an instance of XPathFactory, then create an XPath object to compile and evaluate the expressions.
Evaluate XPath expression: Use the evaluate method to execute the XPath query, returning the results in a NodeList.
Iterate and Output: Finally, iterate through the NodeList and print out the text content of each node.
Conclusion
XPath provides a flexible and powerful way to read and query XML documents in Java. By following these steps, you can easily navigate through your XML data to extract the information you need. The example provided offers a foundation to build upon for more complex XML processing requirements.
Happy coding!
---
Summary: Learn how to read XML files using XPath in Java, a powerful and efficient way to navigate through XML documents.
---
How to Read XML Using XPath in Java
XML (Extensible Markup Language) is widely used for storing and transporting data. If you're working with XML in Java, XPath is an excellent tool for navigating and querying XML documents. In this guide, we'll guide you through the process of reading XML files using XPath in Java, covering the essential steps and code snippets.
What is XPath?
XPath stands for XML Path Language. It is a query language that allows you to navigate through elements and attributes in an XML document. XPath provides a way to address parts of an XML document, making it easier to find specific data within the XML structure.
Setting Up the Environment
Before we dive into reading XML using XPath, let’s ensure we have the necessary setup:
Java Development Kit (JDK): Make sure you have the latest JDK installed.
XML File: Prepare an XML file that we will read using XPath.
[[See Video to Reveal this Text or Code Snippet]]
Reading XML Using XPath in Java
Step 1: Import Required Classes
To start, import the necessary classes from the Java API:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Parse the XML Document
Use DocumentBuilderFactory and DocumentBuilder to parse the XML file into a Document object:
[[See Video to Reveal this Text or Code Snippet]]
Explanation
Create a DocumentBuilderFactory: This factory is configured to return a parser that produces DOM objects from XML files.
Create a DocumentBuilder: This object parses the XML, returning a Document object.
Parse and Normalize: The parse method reads the XML file into the Document object, and normalize ensures the XML structure is in its canonical form.
Create XPath objects: Create an instance of XPathFactory, then create an XPath object to compile and evaluate the expressions.
Evaluate XPath expression: Use the evaluate method to execute the XPath query, returning the results in a NodeList.
Iterate and Output: Finally, iterate through the NodeList and print out the text content of each node.
Conclusion
XPath provides a flexible and powerful way to read and query XML documents in Java. By following these steps, you can easily navigate through your XML data to extract the information you need. The example provided offers a foundation to build upon for more complex XML processing requirements.
Happy coding!