filmov
tv
convert list to tuple python
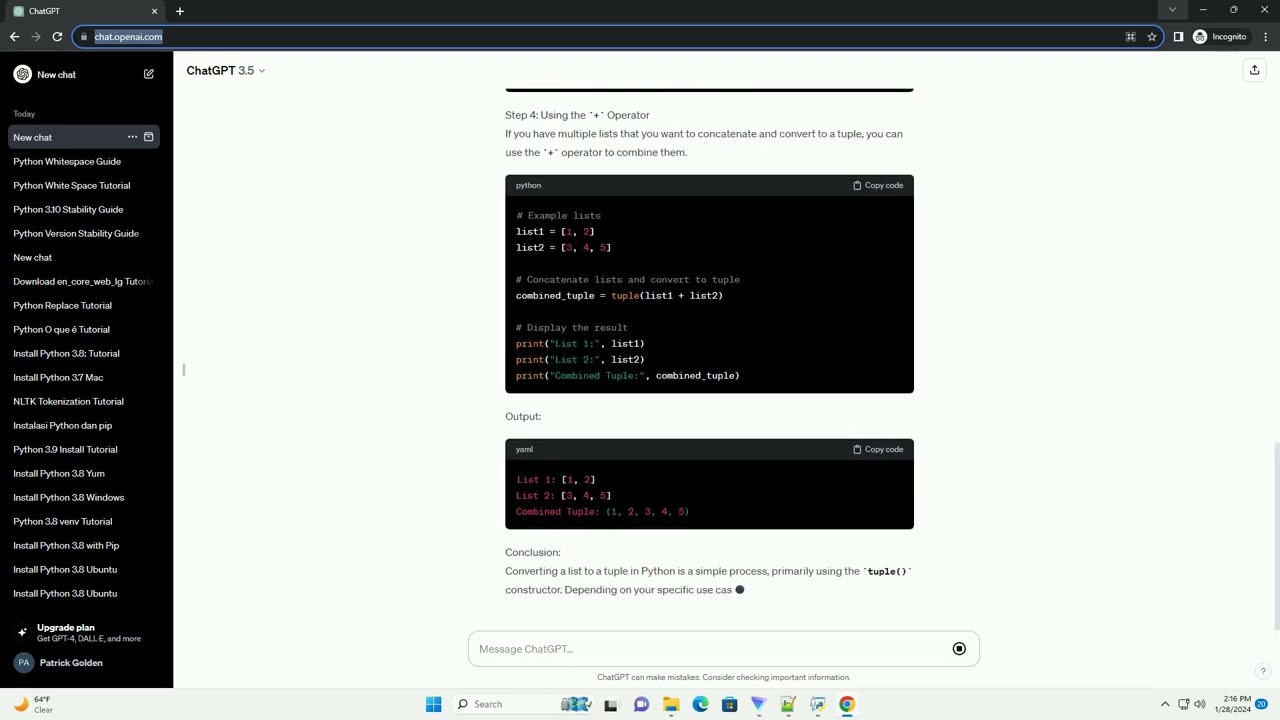
Показать описание
Title: Converting a List to a Tuple in Python: A Step-by-Step Tutorial
Introduction:
In Python, lists and tuples are two commonly used data structures. While lists are mutable, meaning you can modify their elements after creation, tuples are immutable and cannot be changed once defined. There are situations where you might need to convert a list to a tuple, either for data integrity or to meet specific programming requirements. In this tutorial, we'll explore how to convert a list to a tuple in Python with step-by-step explanations and code examples.
Step 1: Understanding Lists and Tuples
Before diving into the conversion process, let's briefly review the characteristics of lists and tuples in Python.
Step 2: Using the tuple() Constructor
The most straightforward way to convert a list to a tuple is by using the tuple() constructor. This constructor takes an iterable (like a list) as an argument and returns a tuple containing the same elements.
Output:
Step 3: The * (Unpacking) Operator
If your list contains nested lists or multiple elements, you can use the * (unpacking) operator to convert the entire list into individual elements for the tuple.
Output:
Step 4: Using the + Operator
If you have multiple lists that you want to concatenate and convert to a tuple, you can use the + operator to combine them.
Output:
Conclusion:
Converting a list to a tuple in Python is a simple process, primarily using the tuple() constructor. Depending on your specific use case, you can also utilize the * operator for unpacking or the + operator for concatenation. Understanding the immutability of tuples is crucial when choosing the appropriate data structure for your program.
ChatGPT
Introduction:
In Python, lists and tuples are two commonly used data structures. While lists are mutable, meaning you can modify their elements after creation, tuples are immutable and cannot be changed once defined. There are situations where you might need to convert a list to a tuple, either for data integrity or to meet specific programming requirements. In this tutorial, we'll explore how to convert a list to a tuple in Python with step-by-step explanations and code examples.
Step 1: Understanding Lists and Tuples
Before diving into the conversion process, let's briefly review the characteristics of lists and tuples in Python.
Step 2: Using the tuple() Constructor
The most straightforward way to convert a list to a tuple is by using the tuple() constructor. This constructor takes an iterable (like a list) as an argument and returns a tuple containing the same elements.
Output:
Step 3: The * (Unpacking) Operator
If your list contains nested lists or multiple elements, you can use the * (unpacking) operator to convert the entire list into individual elements for the tuple.
Output:
Step 4: Using the + Operator
If you have multiple lists that you want to concatenate and convert to a tuple, you can use the + operator to combine them.
Output:
Conclusion:
Converting a list to a tuple in Python is a simple process, primarily using the tuple() constructor. Depending on your specific use case, you can also utilize the * operator for unpacking or the + operator for concatenation. Understanding the immutability of tuples is crucial when choosing the appropriate data structure for your program.
ChatGPT