filmov
tv
Python Exercise for Beginners #27 | For loop example : Find Factorial of Number in Python
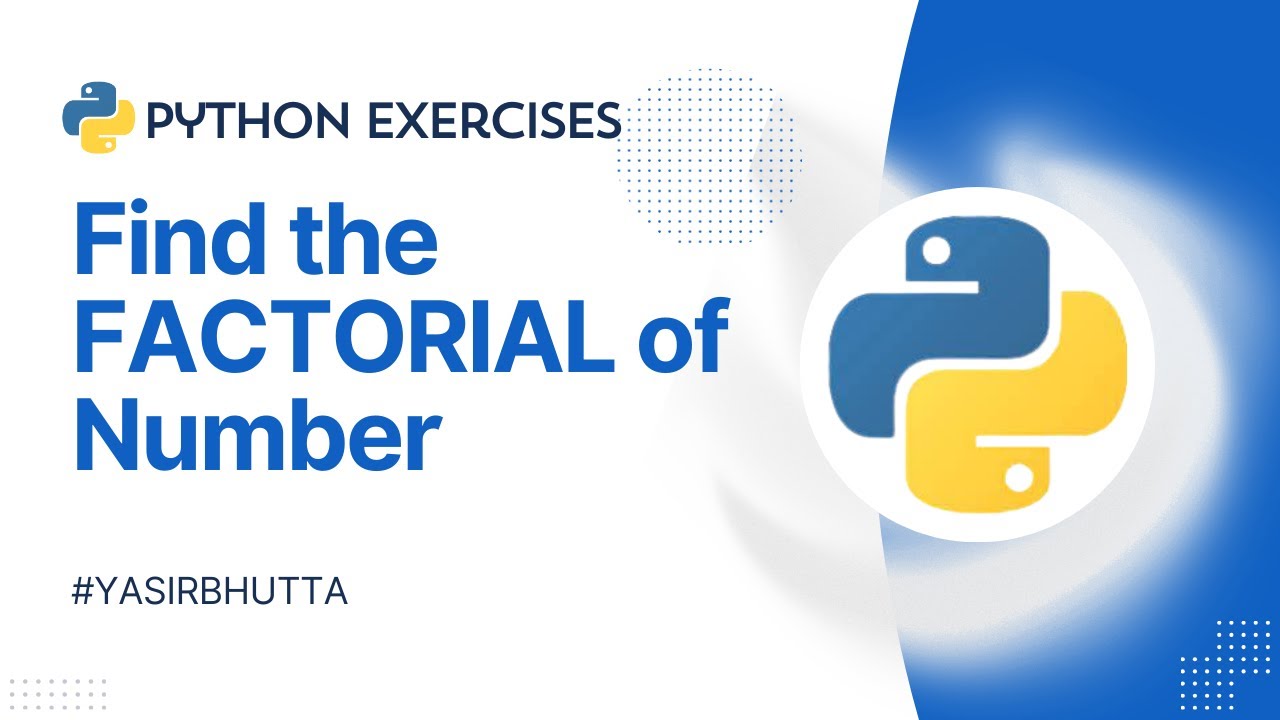
Показать описание
@yasirbhutta In this video, you'll learn how to calculate the factorial of a number in Python with a simple and beginner-friendly code example. We'll walk through the logic behind the factorial function, covering edge cases like negative numbers and the special cases of 0 and 1.
**What You'll Learn:**
- How to handle negative numbers when calculating factorials.
- Using conditional statements to manage special cases.
- Implementing a loop to multiply a series of numbers for factorial calculation.
- How to use Python's `range()` function effectively.
Explanation of Video Code:
This Python code is designed to calculate the factorial of a given number (`num`). Here's a breakdown of what each part does:
1. **Initialization:**
- `num = 0`: This initializes the variable `num` to 0. The factorial of 0 is defined as 1.
- `result = None`: The `result` variable is initialized to `None`. This variable will later store the factorial value.
2. **Factorial Calculation:**
- `if num less than 0:`: This checks if `num` is negative. The factorial is not defined for negative numbers, so the code prints "Factorial is not defined" if `num` is less than 0.
- `elif num == 0 or num == 1:`: This condition checks if `num` is either 0 or 1. The factorial of both 0 and 1 is 1, so the code sets `result` to 1 in this case.
- `else:`: If `num` is greater than 1, the code enters this block to calculate the factorial.
- `result = 1`: The `result` is initialized to 1.
- `for i in range(1, num + 1):`: This loop iterates over all integers from 1 to `num`.
- `result *= i`: In each iteration, `result` is multiplied by the current value of `i`, effectively calculating the factorial by repeated multiplication.
3. **Result Output:**
- `if result is not None:`: This checks if the `result` is not `None` (i.e., a factorial was calculated).
- `print(f"Factorial of {num} is {result}")`: This prints the result in a formatted string, displaying the factorial of the given number.
### Example of Execution
If `num = 5`, the code will calculate `5! = 5 * 4 * 3 * 2 * 1 = 120` and print `Factorial of 5 is 120`.
For `num = 0`, it will simply print `Factorial of 0 is 1` since the factorial of 0 is defined as 1 by convention.
For any negative `num`, it will print `Factorial is not defined`.
Whether you're just starting out or looking to strengthen your Python skills, this tutorial will guide you through the process of creating a functional factorial calculator.
📌 **Don't forget to like, share, and subscribe for more Python tutorials!**
#pythontips #python #python3 #pythonlearning #programming #coding #technology #machinelearning #pythonprogramming #datascience #tech #codinglife #development
**What You'll Learn:**
- How to handle negative numbers when calculating factorials.
- Using conditional statements to manage special cases.
- Implementing a loop to multiply a series of numbers for factorial calculation.
- How to use Python's `range()` function effectively.
Explanation of Video Code:
This Python code is designed to calculate the factorial of a given number (`num`). Here's a breakdown of what each part does:
1. **Initialization:**
- `num = 0`: This initializes the variable `num` to 0. The factorial of 0 is defined as 1.
- `result = None`: The `result` variable is initialized to `None`. This variable will later store the factorial value.
2. **Factorial Calculation:**
- `if num less than 0:`: This checks if `num` is negative. The factorial is not defined for negative numbers, so the code prints "Factorial is not defined" if `num` is less than 0.
- `elif num == 0 or num == 1:`: This condition checks if `num` is either 0 or 1. The factorial of both 0 and 1 is 1, so the code sets `result` to 1 in this case.
- `else:`: If `num` is greater than 1, the code enters this block to calculate the factorial.
- `result = 1`: The `result` is initialized to 1.
- `for i in range(1, num + 1):`: This loop iterates over all integers from 1 to `num`.
- `result *= i`: In each iteration, `result` is multiplied by the current value of `i`, effectively calculating the factorial by repeated multiplication.
3. **Result Output:**
- `if result is not None:`: This checks if the `result` is not `None` (i.e., a factorial was calculated).
- `print(f"Factorial of {num} is {result}")`: This prints the result in a formatted string, displaying the factorial of the given number.
### Example of Execution
If `num = 5`, the code will calculate `5! = 5 * 4 * 3 * 2 * 1 = 120` and print `Factorial of 5 is 120`.
For `num = 0`, it will simply print `Factorial of 0 is 1` since the factorial of 0 is defined as 1 by convention.
For any negative `num`, it will print `Factorial is not defined`.
Whether you're just starting out or looking to strengthen your Python skills, this tutorial will guide you through the process of creating a functional factorial calculator.
📌 **Don't forget to like, share, and subscribe for more Python tutorials!**
#pythontips #python #python3 #pythonlearning #programming #coding #technology #machinelearning #pythonprogramming #datascience #tech #codinglife #development
Комментарии