filmov
tv
How to Update State with Conditional Logic in React: Avoiding Common Errors
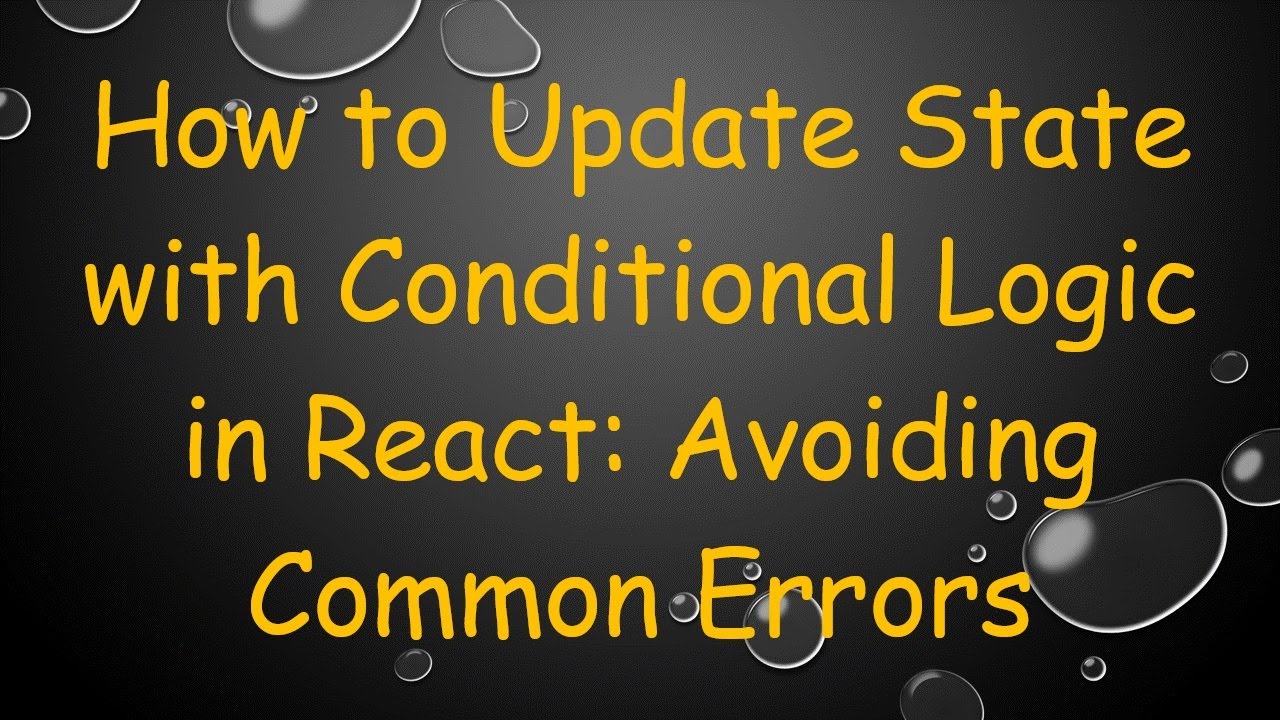
Показать описание
Learn how to effectively conditionally update an array of objects in React state without encountering errors like 'not iterable'.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: React - state contains array of objects. setState with conditional
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding State Updates in React: A Conditional Approach
In the world of React, managing state is a fundamental concept that can often lead to confusion, especially when dealing with arrays of objects. One common challenge developers face involves updating state based on object properties. This issue is highlighted in a scenario where an error occurs when attempting to loop through a state variable containing an array of objects. Let's break this down to understand the problem and how to efficiently solve it.
The Problem
Suppose you have a React component with a state variable that holds an array of objects. Here’s a simplified example of the state setup:
[[See Video to Reveal this Text or Code Snippet]]
Now, you want to update the name of a specific object within this array conditionally. You might try to implement this with a loop, like so:
[[See Video to Reveal this Text or Code Snippet]]
However, this leads to the error: TypeError: exampleState is not iterable. Why does this happen?
The Issue Explained
The error occurs primarily because of two reasons:
Unintended State Structure Change: The way you're attempting to update the state is incorrect. You’re trying to spread an object instead of the entire array which results in a confusion in the type of data being passed to setExampleState.
Multiple Updates: Furthermore, calling setExampleState multiple times inside a loop is not ideal in React. Each call to this function triggers a re-render, which is inefficient.
The Solution
To update an array of objects conditionally in a React state, you should use map to iterate over the array and return a new array with the updates you need. Here’s how you can do it correctly:
[[See Video to Reveal this Text or Code Snippet]]
Breaking Down the Solution
Here’s why this solution works better:
Using map: The map function creates a new array by calling a function on every element in the calling array. This means the original state is not mutated directly, adhering to React's data immutability principle.
Conditional Return: We check each user’s name, and if it matches ‘John’, we create a new object with the updated name. If it doesn’t match, we simply return the user unchanged.
Single State Update Call: We only call setExampleState once, which optimizes the re-rendering process in React.
Conclusion
Conditional updates in React state can be tricky, but with the correct pattern, it becomes manageable. By leveraging map and ensuring proper data structures, you can avoid common pitfalls like the 'not iterable' error. This approach not only adheres to best practices but also improves the performance of your React component through reduced re-renders.
By following the outlined method, you should find it easier to manage and update arrays of objects in your React applications. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: React - state contains array of objects. setState with conditional
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding State Updates in React: A Conditional Approach
In the world of React, managing state is a fundamental concept that can often lead to confusion, especially when dealing with arrays of objects. One common challenge developers face involves updating state based on object properties. This issue is highlighted in a scenario where an error occurs when attempting to loop through a state variable containing an array of objects. Let's break this down to understand the problem and how to efficiently solve it.
The Problem
Suppose you have a React component with a state variable that holds an array of objects. Here’s a simplified example of the state setup:
[[See Video to Reveal this Text or Code Snippet]]
Now, you want to update the name of a specific object within this array conditionally. You might try to implement this with a loop, like so:
[[See Video to Reveal this Text or Code Snippet]]
However, this leads to the error: TypeError: exampleState is not iterable. Why does this happen?
The Issue Explained
The error occurs primarily because of two reasons:
Unintended State Structure Change: The way you're attempting to update the state is incorrect. You’re trying to spread an object instead of the entire array which results in a confusion in the type of data being passed to setExampleState.
Multiple Updates: Furthermore, calling setExampleState multiple times inside a loop is not ideal in React. Each call to this function triggers a re-render, which is inefficient.
The Solution
To update an array of objects conditionally in a React state, you should use map to iterate over the array and return a new array with the updates you need. Here’s how you can do it correctly:
[[See Video to Reveal this Text or Code Snippet]]
Breaking Down the Solution
Here’s why this solution works better:
Using map: The map function creates a new array by calling a function on every element in the calling array. This means the original state is not mutated directly, adhering to React's data immutability principle.
Conditional Return: We check each user’s name, and if it matches ‘John’, we create a new object with the updated name. If it doesn’t match, we simply return the user unchanged.
Single State Update Call: We only call setExampleState once, which optimizes the re-rendering process in React.
Conclusion
Conditional updates in React state can be tricky, but with the correct pattern, it becomes manageable. By leveraging map and ensuring proper data structures, you can avoid common pitfalls like the 'not iterable' error. This approach not only adheres to best practices but also improves the performance of your React component through reduced re-renders.
By following the outlined method, you should find it easier to manage and update arrays of objects in your React applications. Happy coding!