filmov
tv
JavaScript Tutorial - 8 - Iterators
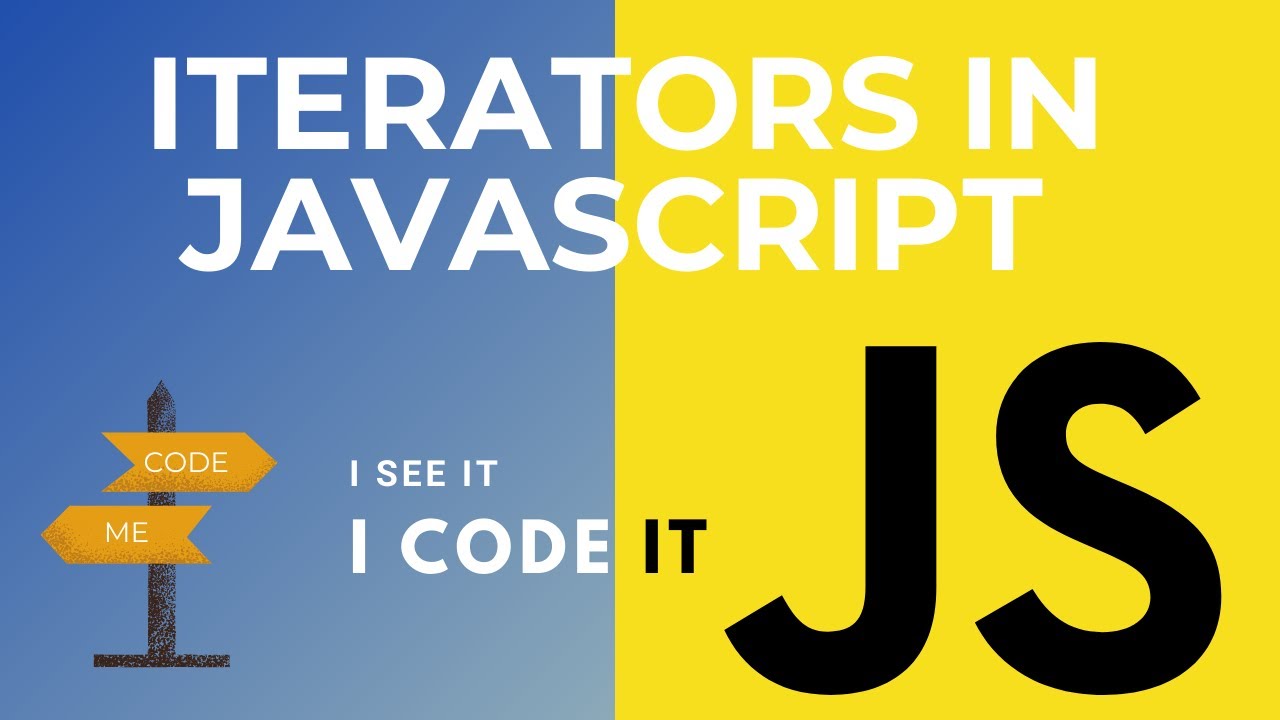
Показать описание
Introduction to Iterators
Imagine you had a grocery list and you wanted to know what each item on the list was. You’d have to scan through each row and check for the item. This common task is similar to what we have to do when we want to iterate over, or loop through, an array. One tool at our disposal is the for loop. However, we also have access to built-in array methods which make looping easier.
The built-in JavaScript array methods that help us iterate are called iteration methods, at times referred to as iterators. Iterators are methods called on arrays to manipulate elements and return values.
1. --The .forEach() Method
The first iteration method that we’re going to learn is .forEach()
const fruits = ['mango', 'papaya', 'pineapple', 'apple'];
//First Methord
function(fruitItems)
{
}
);
//Second methord
//Third Methord
function printFruits(element)
{
}
2.--The .map() Method (Return Array)
The second iterator we’re going to cover is .map(). When .map() is called on an array, it takes an argument of a callback function and returns a new array!
const animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
// Create the secretMessage array below
const bigNumbers = [100, 200, 300, 400, 500];
// Create the smallNumbers array below
{
return bigNumbers/100;
}
);
3.--The .filter() Method (Return Arrays)
Another useful iterator method is .filter(). Like .map(), .filter() returns a new array. However, .filter() returns an array of elements after filtering out certain elements from the original array. The callback function for the .filter() method should return true or false depending on the element that is passed to it. The elements that cause the callback function to return true are added to the new array. Take a look at the following example:
const randomNumbers = [375, 200, 3.14, 7, 13, 852];
// Call .filter() on randomNumbers below
randomNumbers ={
return randomNumbers =250;
}
);
const favoriteWords = ['nostalgia', 'hyperbole', 'fervent', 'esoteric', 'serene'];
// Call .filter() on favoriteWords below
favoriteWords ={ //ThisLineFavoriteWord is replacable
}
);
4.--The .findIndex() Method (Return element based on Index)
We sometimes want to find the location of an element in an array. That’s where the .findIndex() method comes in! Calling .findIndex() on an array will return the index of the first element that evaluates to true in the callback function.
const animals = ['hippo', 'tiger', 'lion', 'seal', 'cheetah', 'monkey', 'salamander', 'elephant'];
animal ={
return animal === 'elephant';
}
);
animal ={
return animal[0]==='s';
}
);
---------------
The .reduce() Method
Another widely used iteration method is .reduce(). The .reduce() method returns a single value after iterating through the elements of an array, thereby reducing the array.
//First Methord
const numbers = [1, 2, 4, 10];
return accumulator + currentValue
})
//Second methord
const numbers = [1, 2, 4, 10];
return accumulator + currentValue
}, 100) // - Second argument for .reduce()
//Third methord
const newNumbers = [1, 3, 5, 7];
return accumulator + currentValue;
}, 10);
Iterator Documentation
There are many additional built-in array methods, a complete list of which is on the MDN’s Array iteration methods page.
No doubt, giving food to a hungry person is indeed a great donation, but the greatest donation of all is to give education. Food gives a momentary satisfaction where as education empowers the person for the rest of his life and enables him to earn his own food.
Let's gift education together
Imagine you had a grocery list and you wanted to know what each item on the list was. You’d have to scan through each row and check for the item. This common task is similar to what we have to do when we want to iterate over, or loop through, an array. One tool at our disposal is the for loop. However, we also have access to built-in array methods which make looping easier.
The built-in JavaScript array methods that help us iterate are called iteration methods, at times referred to as iterators. Iterators are methods called on arrays to manipulate elements and return values.
1. --The .forEach() Method
The first iteration method that we’re going to learn is .forEach()
const fruits = ['mango', 'papaya', 'pineapple', 'apple'];
//First Methord
function(fruitItems)
{
}
);
//Second methord
//Third Methord
function printFruits(element)
{
}
2.--The .map() Method (Return Array)
The second iterator we’re going to cover is .map(). When .map() is called on an array, it takes an argument of a callback function and returns a new array!
const animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
// Create the secretMessage array below
const bigNumbers = [100, 200, 300, 400, 500];
// Create the smallNumbers array below
{
return bigNumbers/100;
}
);
3.--The .filter() Method (Return Arrays)
Another useful iterator method is .filter(). Like .map(), .filter() returns a new array. However, .filter() returns an array of elements after filtering out certain elements from the original array. The callback function for the .filter() method should return true or false depending on the element that is passed to it. The elements that cause the callback function to return true are added to the new array. Take a look at the following example:
const randomNumbers = [375, 200, 3.14, 7, 13, 852];
// Call .filter() on randomNumbers below
randomNumbers ={
return randomNumbers =250;
}
);
const favoriteWords = ['nostalgia', 'hyperbole', 'fervent', 'esoteric', 'serene'];
// Call .filter() on favoriteWords below
favoriteWords ={ //ThisLineFavoriteWord is replacable
}
);
4.--The .findIndex() Method (Return element based on Index)
We sometimes want to find the location of an element in an array. That’s where the .findIndex() method comes in! Calling .findIndex() on an array will return the index of the first element that evaluates to true in the callback function.
const animals = ['hippo', 'tiger', 'lion', 'seal', 'cheetah', 'monkey', 'salamander', 'elephant'];
animal ={
return animal === 'elephant';
}
);
animal ={
return animal[0]==='s';
}
);
---------------
The .reduce() Method
Another widely used iteration method is .reduce(). The .reduce() method returns a single value after iterating through the elements of an array, thereby reducing the array.
//First Methord
const numbers = [1, 2, 4, 10];
return accumulator + currentValue
})
//Second methord
const numbers = [1, 2, 4, 10];
return accumulator + currentValue
}, 100) // - Second argument for .reduce()
//Third methord
const newNumbers = [1, 3, 5, 7];
return accumulator + currentValue;
}, 10);
Iterator Documentation
There are many additional built-in array methods, a complete list of which is on the MDN’s Array iteration methods page.
No doubt, giving food to a hungry person is indeed a great donation, but the greatest donation of all is to give education. Food gives a momentary satisfaction where as education empowers the person for the rest of his life and enables him to earn his own food.
Let's gift education together