filmov
tv
ES6 and Typescript Tutorial - 35 - WeakMaps
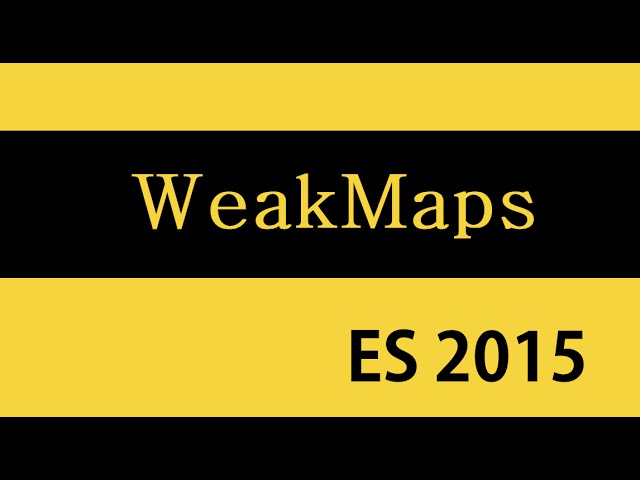
Показать описание
📱 Follow Codevolution
ES6 | ES2015 | Typescript | ES6 Tutorial | ES2015 Tutorial | Typescript Tutorial | ES6 Tutorial for Beginners | ES2015 Tutorial for Beginners | Typescript tutorial for Beginners
ES6 Tutorial: Learn Modern JavaScript in 1 Hour
ES6 and Typescript Tutorial - 1 - Introduction
ES6 and Typescript Tutorial - 21 - Class
TypeScript Tutorial for Beginners
TypeScript vs JavaScript | Guido van Rossum and Lex Fridman
ES6 and Typescript Tutorial - 23 - Class Inheritance
ES6 and Typescript Tutorial - 29 - Sets & Maps
ES6 and Typescript Tutorial - 22 - Class Body and Methods
ES6 and Typescript Tutorial - 40 - Generators
ES6 and Typescript Tutorial - 3 - Tooling Setup
ES6 and Typescript Tutorial - 36 - Symbols
ES6 and Typescript Tutorial - 4 - ScratchJS
ES6 and Typescript Tutorial - 32 - Maps
ES6 and Typescript Tutorial - 2 - Transpiler
JavaScript ES6 Arrow Functions Tutorial
ES6 and Typescript Tutorial - 30 - Sets
ES6 and Typescript Tutorial - 9 - let vs const
ES6 and Typescript Tutorial - 13 - Rest Operator
ES6 and Typescript Tutorial - 11 - lexical 'this'
ES6 and Typescript Tutorial - 41 - Enter Typescript
ES6 and Typescript Tutorial - 17 - Destructuring Array
ES6 and Typescript Tutorial - 10 - Arrow Functions
ES6 and Typescript Tutorial - 15 - Object Literals Part 1
How to Setup Node.js with TypeScript in 2023
Комментарии