filmov
tv
Python Tutorial: How To Convert Number Types In Python
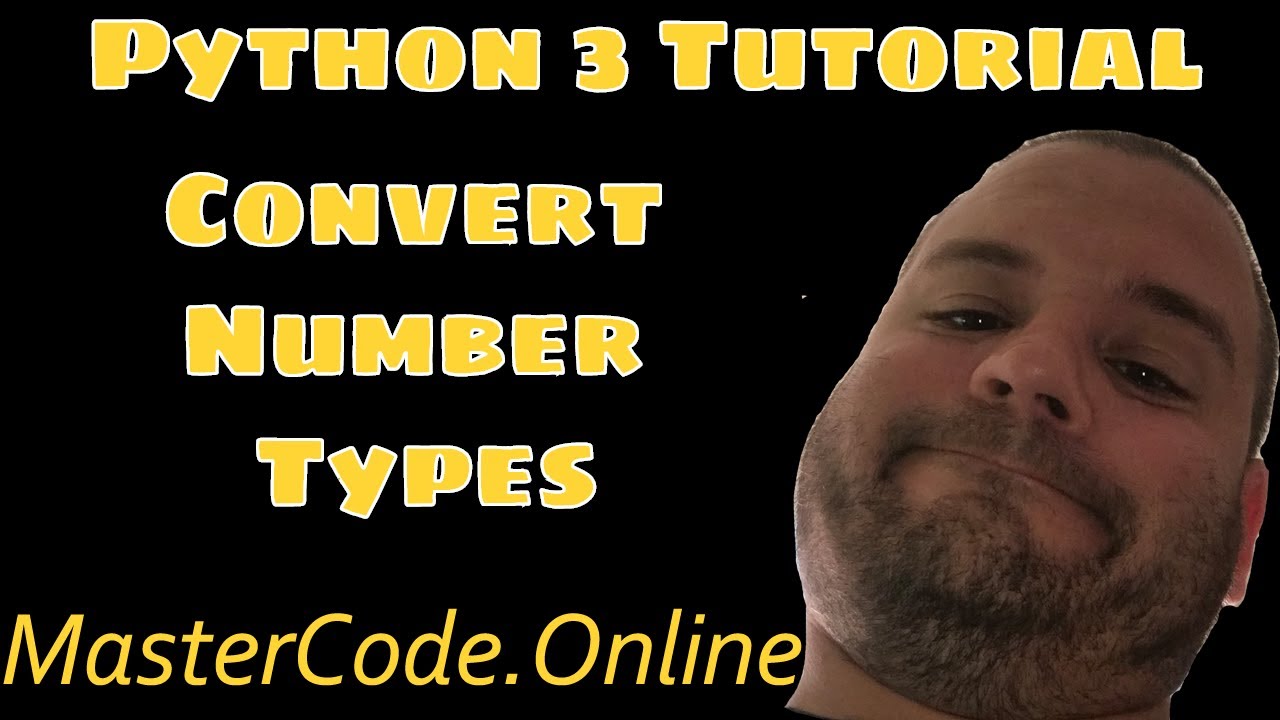
Показать описание
Be sure to like, share and comment to show your support for our tutorials.
=======================================
======================================
How To Convert Number Types in Python
When working with numbers in Python having the ability to change the type of number is very important. For example, if we had a floating point number but we need an integer it is nice to be able to switch them back and forth. In this tutorial, we will look at how to access the type information for an object and also how to change the type.
How To Access An Object's Type Information
We are able to able to access an object's type information using the built-in function type() this will return the current type information to us. Let's take a look at a few examples of this.
Examples of Built-in Function Type()
type(5)
class 'int'
type(100.4)
class 'float'
type("car")
class 'str'
type(False)
class 'bool'
How to Change Type of an Object
We will focus only on changing numbers in this tutorial since we are working with numbers. We can change a floating point number to a float using int() this number will always round down or we can change an integer to a float using float().
# Change a String to an Integer
int("3")
3
# Change a Floating Point number to an integer but always rounds down
int(6.7)
6
# Change a Floating Point Number to an integer but round up
round(6.7)
7
# Could do it this way as well but more typing not for lazy people
int(round(6.7))
7
# Convert a string to a floating point number
float("3")
3.0
# Convert an integer to a floating point number
float(6)
6.0
#Converting a string that contains a number similar to a floating point number is not possible
int("7.5")
Traceback (most recent call last):
File "stdin", line 1, in module
ValueError: invalid literal for int() with base 10: '7.5'
# The Fix
int(float("7.5"))
7
round(float("7.5"))
8
#just proving that "7.5" is a string
type("7.5")
class 'str'
Any questions about converting types in Python leave a comment below.
=======================================
======================================
How To Convert Number Types in Python
When working with numbers in Python having the ability to change the type of number is very important. For example, if we had a floating point number but we need an integer it is nice to be able to switch them back and forth. In this tutorial, we will look at how to access the type information for an object and also how to change the type.
How To Access An Object's Type Information
We are able to able to access an object's type information using the built-in function type() this will return the current type information to us. Let's take a look at a few examples of this.
Examples of Built-in Function Type()
type(5)
class 'int'
type(100.4)
class 'float'
type("car")
class 'str'
type(False)
class 'bool'
How to Change Type of an Object
We will focus only on changing numbers in this tutorial since we are working with numbers. We can change a floating point number to a float using int() this number will always round down or we can change an integer to a float using float().
# Change a String to an Integer
int("3")
3
# Change a Floating Point number to an integer but always rounds down
int(6.7)
6
# Change a Floating Point Number to an integer but round up
round(6.7)
7
# Could do it this way as well but more typing not for lazy people
int(round(6.7))
7
# Convert a string to a floating point number
float("3")
3.0
# Convert an integer to a floating point number
float(6)
6.0
#Converting a string that contains a number similar to a floating point number is not possible
int("7.5")
Traceback (most recent call last):
File "stdin", line 1, in module
ValueError: invalid literal for int() with base 10: '7.5'
# The Fix
int(float("7.5"))
7
round(float("7.5"))
8
#just proving that "7.5" is a string
type("7.5")
class 'str'
Any questions about converting types in Python leave a comment below.
Комментарии