filmov
tv
Node JS Tutorial for Beginners #8 - The Node Event Emitter
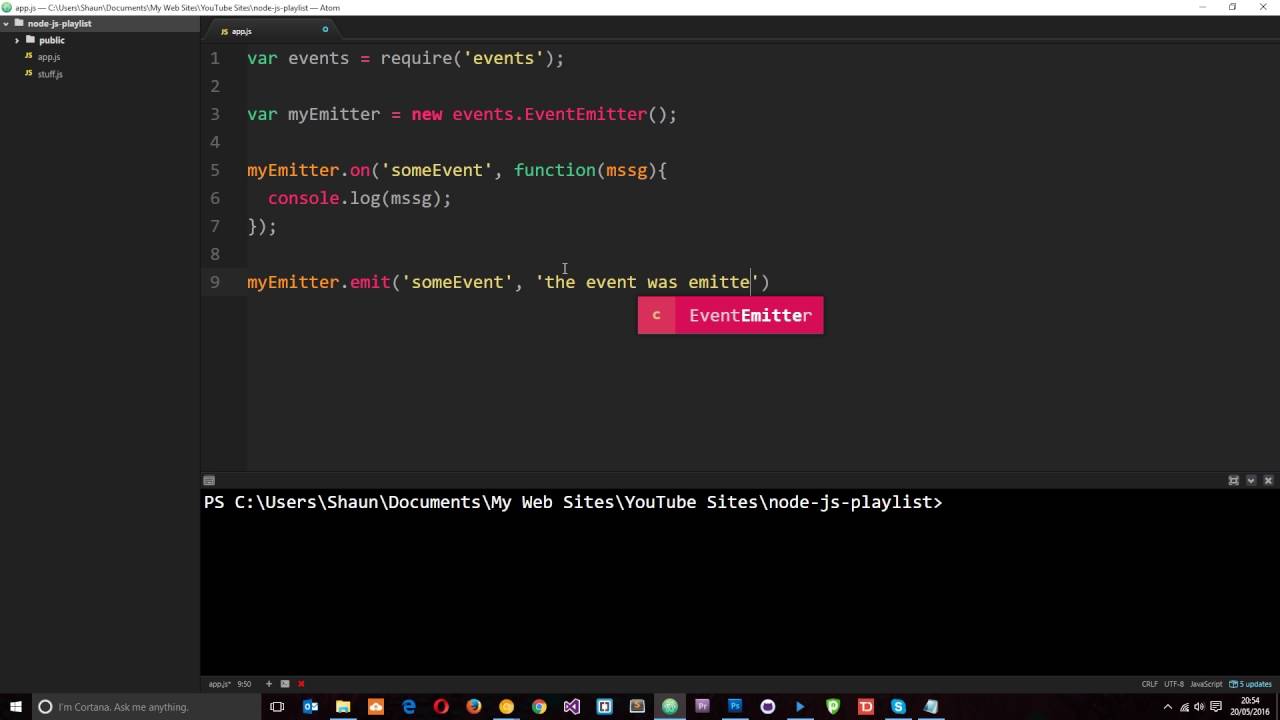
Показать описание
Yo gang, in this Node JS tutorial, I'll be introducing you to the event emitter in the Node core. The event emitter allows us to wire up custom events to our own objects and then emit those events at a later point in time.
----- COURSE LINKS:
---------------------------------------------------------------------------------------------
You can find more front-end development tutorials on CSS, HTML, JavaScript, jQuery, WordPress & more on the channel homepage...
========== JavaScript for Beginners Playlist ==========
============ CSS for Beginners Playlist =============
============== The Net Ninja =====================
================== Social Links ==================
----- COURSE LINKS:
---------------------------------------------------------------------------------------------
You can find more front-end development tutorials on CSS, HTML, JavaScript, jQuery, WordPress & more on the channel homepage...
========== JavaScript for Beginners Playlist ==========
============ CSS for Beginners Playlist =============
============== The Net Ninja =====================
================== Social Links ==================
Node.js Tutorial for Beginners: Learn Node in 1 Hour
Node.js Ultimate Beginner’s Guide in 7 Easy Steps
Learn Node.js - Full Tutorial for Beginners
Node.js Tutorial For Absolute Beginners
Node Js Tutorial for beginners in Tamil 2024 | Full Course for Beginners | 3 HRS | @Balachandra_in
Node.js Tutorial for Beginners | CRASH COURSE
What is Node.js and how it works (explained in 2 minutes)
Node.js Crash Course
Create a Jenkins CI/CD pipeline with GitHub, Node js project ! #jenkins #nodejs #github #cicd
Node.js Full Course for Beginners | Complete All-in-One Tutorial | 7 Hours
Node.js and Express.js - Full Course
Node.JS Full Course (THREE HOUR All-in-One Tutorial for Beginners)
Node JS Tutorial | Crash Course
Node Js Tutorial in Hindi 🔥🔥
Why you Shouldn't use NODE JS in 2023
Node.js Tutorial for Beginners - Getting Started with NodeJS Basics
Node.js 101 Crash Course: Learn Node.js (6 HOURS!) + Build Your First Project [2024]
Node JS Full Course - Learn Node.js in 7 Hours | Node.js Tutorial for Beginners | Edureka
What Is Node.js? | Introduction To Node.js | Node JS Tutorial For Beginners | Simplilearn
Learn Express JS In 35 Minutes
Learn Node.js & Express with Project in 2 Hours
What is Node js? | Simplified Explanation
🚀 Backend (Node JS) Series - Learn What Matters 1: Understanding the Internet
Node JS for Beginners in Tamil | Full Video
Комментарии