filmov
tv
C# tips and tricks 23 - How to serialize & deserialize object to JSON using newtonsoft.JSON library
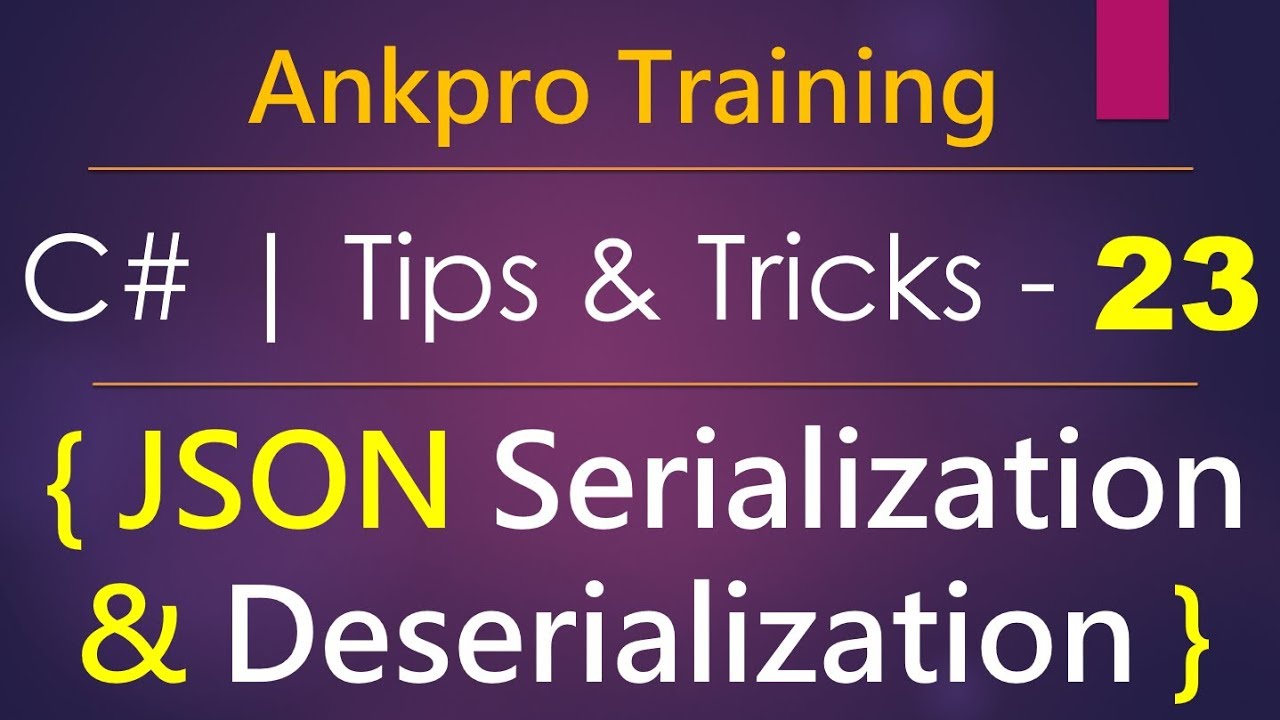
Показать описание
What is JSON?
JSON (JavaScript Object Notation) is a lightweight data-interchange format. It is easy for humans to read and write and easy for machines to parse and generate. JSON is a text format that is completely language independent.
JSON supports the following two data structures,
• Collection of name/value pairs - This Data Structure is supported by different programming languages.
• Ordered list of values - It includes array, list, vector or sequence etc.
Serialization
Serialization is the saving of the structure or state of objects or data.
The target that you save to may be in memory, on a hard-disk, or by internet protocols, or other protocols, to a local or remote database, server, etc.
Here we use SerializeObject method with JsonConvert class to do serialization.
Deserialization
Deserialization is the reading back in from the same types of storage you saved on a hard-disk, or by internet protocols, or other protocols , refresh the state of existing objects by setting their properties, field, etc.
Or you may deserialize to completely re-create classes, user interfaces, and code, instances of classes, values for properties, fields, etc.
Here we use DeserializeObject generic method with JsonConvert class to do deserialization.
NewtonSoft.Json library
World-class JSON Serializer
Serialize and deserialize any .NET object with Json.NET's powerful JSON serializer.
High Performance
50% faster than DataContractJsonSerializer, and 250% faster than JavaScriptSerializer.
Open Source
Json.NET is open source software and is completely free for commercial use.
Easy to Use
Json.NET makes the simple easy and the complex possible.
Run Anywhere
Json.NET supports Windows, Windows Store, Windows Phone, Mono, and Xamarin.
JSON Path
Query JSON with an XPath-like syntax.
CODE :(Replace lessthan with less than symbol and greaterthan with greater than symbol)
using Newtonsoft.Json;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace JSON
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Serialization");
// 1. Serialization
Movie movie = new Movie { Id = 1, Title = "Mission Impossible" };
// movie is a object let us convert this to a string by using JsonConvert.SerializeObject
string result = JsonConvert.SerializeObject(movie);
// Converts to string as {"Id":1,"Title":"Mission Impossible"}
Console.WriteLine(result);
Console.WriteLine("\nDeserialization");
// 2. Deserialization
Movie newMovie = JsonConvert.DeserializeObject lessthan Movie greaterthan (result);
// Now string is converted to object
Console.WriteLine("Id : " + newMovie.Id);
Console.WriteLine("Title : " + newMovie.Title);
Console.WriteLine("\nSerialization of collection");
// 3. Serialization of collection
List lessthan Movie greaterthan movies = new List lessthanMovie greaterthan {
new Movie{ Id=1, Title="Titanic" },
new Movie{ Id=2, Title="The martian"},
new Movie{ Id=3, Title="Black panther"} ,
new Movie{ Id=4, Title="Deadpool 2"} ,
};
string collectionResult = JsonConvert.SerializeObject(movies);
Console.WriteLine(collectionResult);
Console.WriteLine("\nDeserialization of collection");
// 4. Deserialization of collection
List lessthan Movie greaterthan newMovies = JsonConvert.DeserializeObject lessthan List lessthan Movie greaterthan greaterthan (collectionResult);
foreach (var item in newMovies)
{
Console.WriteLine("Id : "+item.Id+"\tTitle : "+item.Title);
}
}
class Movie
{
public int Id { get; set; }
public string Title { get; set; }
}
}
}
ankpro
ankpro training
C#
C sharp
Bangalore
Rajajinagar
Selenium
Coded UI
Mobile automation testing
Mobile testing
JQuery
JavaScript
.Net
C
C++
Components of the .Net framework
Hello World
Literal
Keywords
Variable
Data types
Operators
Branching
Loops
Arrays
Strings
Structures
Enums
Functions
Комментарии