filmov
tv
Java Tutorial for Beginners 2020 - Day 5 | OOP Encapsulation Inheritance Abstraction Polymorphism
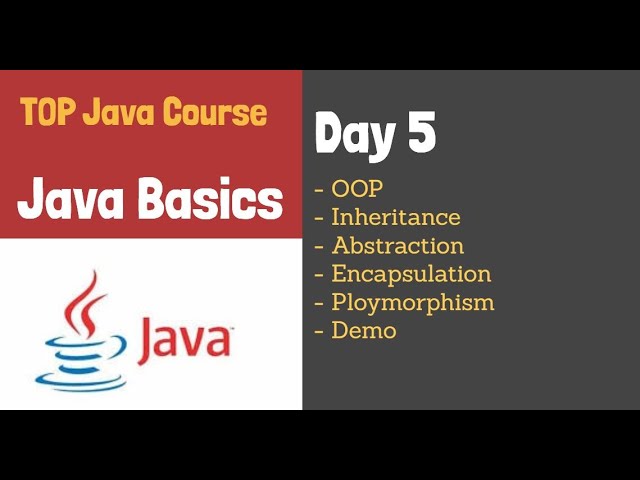
Показать описание
#learnjava #learnjavabasics #javatutorial
Java Basics Day 5 | Java Tutorial
Java Tutorial for Beginners 2020
Learn Java programming from expert software engineers.
Object Oriented Programming
Encapsulation
Inheritance
Polymorphism
Demo
An object-based application in Java is based on declaring classes, creating objects from them and interacting between these objects.
The building blocks of object-oriented programming are following :
Inheritance
Encapsulation
Abstraction
Polymorphism
Benefits of Object Oriented Programming
Improved productivity during software development
Improved software maintainability
Faster development sprints
Lower cost of development
Higher quality software
Inheritance is one such concept where the properties of one class can be inherited by the other. It helps to reuse the code and establish a relationship between different classes.
Parent class ( Super or Base class)
Child class (Subclass or Derived class )
A class which inherits the properties is known as Child Class whereas a class whose properties are inherited is known as Parent class.
Class A
{
// properties and methods
}
Class B extends A {
// properties and methods
}
Encapsulation is a mechanism where we bind the data and code together as a single unit. It also means to hide a data in order to make it safe from any modification.
In encapsulation the methods and variables of a class are well hidden and safe.
Declaring the variables of a class as private.
Providing public setter and getter methods to modify and view the variables values.
Abstraction basically deals with hiding the details and showing the essential things to the user.
Real Life example is a T.V remote control. We hide all the complexities inside the remote control and provide a user friendly interface to the user to control volume and channels.
We can achieve abstraction in two ways:
Abstract Class
Interface
Abstract class in Java contains the ‘abstract’ keyword.
If a class is declared abstract, it cannot be instantiated, which means you cannot create an object of an abstract class. Also, an abstract class can contain abstract as well as concrete methods.
Abstract methods have no body. The classes which extends the abstract class will provide a body for abstract methods
Abstract class Vehicle {
int speed;
void run(){
}
abstract void increaseSpeed(int speed);
}
class BMW extends Vehicle{
String engineType;
void increaseSpeed(int speed){
}
}
Interface in Java is a blueprint of a class or we can say it is a collection of abstract methods and static constants.
In an interface, each method is public and abstract but it does not contain any constructor.
Along with abstraction, interface also helps to achieve multiple inheritance in Java.
Interface is a contract.
Java Tutorial for Beginners 2020 - Day 4 | IO Streams, Serialization and JDBC
Java Basics Day 5 | Java Tutorial
Java Tutorial for Beginners 2020
Learn Java programming from expert software engineers.
Object Oriented Programming
Encapsulation
Inheritance
Polymorphism
Demo
An object-based application in Java is based on declaring classes, creating objects from them and interacting between these objects.
The building blocks of object-oriented programming are following :
Inheritance
Encapsulation
Abstraction
Polymorphism
Benefits of Object Oriented Programming
Improved productivity during software development
Improved software maintainability
Faster development sprints
Lower cost of development
Higher quality software
Inheritance is one such concept where the properties of one class can be inherited by the other. It helps to reuse the code and establish a relationship between different classes.
Parent class ( Super or Base class)
Child class (Subclass or Derived class )
A class which inherits the properties is known as Child Class whereas a class whose properties are inherited is known as Parent class.
Class A
{
// properties and methods
}
Class B extends A {
// properties and methods
}
Encapsulation is a mechanism where we bind the data and code together as a single unit. It also means to hide a data in order to make it safe from any modification.
In encapsulation the methods and variables of a class are well hidden and safe.
Declaring the variables of a class as private.
Providing public setter and getter methods to modify and view the variables values.
Abstraction basically deals with hiding the details and showing the essential things to the user.
Real Life example is a T.V remote control. We hide all the complexities inside the remote control and provide a user friendly interface to the user to control volume and channels.
We can achieve abstraction in two ways:
Abstract Class
Interface
Abstract class in Java contains the ‘abstract’ keyword.
If a class is declared abstract, it cannot be instantiated, which means you cannot create an object of an abstract class. Also, an abstract class can contain abstract as well as concrete methods.
Abstract methods have no body. The classes which extends the abstract class will provide a body for abstract methods
Abstract class Vehicle {
int speed;
void run(){
}
abstract void increaseSpeed(int speed);
}
class BMW extends Vehicle{
String engineType;
void increaseSpeed(int speed){
}
}
Interface in Java is a blueprint of a class or we can say it is a collection of abstract methods and static constants.
In an interface, each method is public and abstract but it does not contain any constructor.
Along with abstraction, interface also helps to achieve multiple inheritance in Java.
Interface is a contract.
Java Tutorial for Beginners 2020 - Day 4 | IO Streams, Serialization and JDBC