filmov
tv
How to Shuffle an Array and Display Elements with a Button in JavaScript
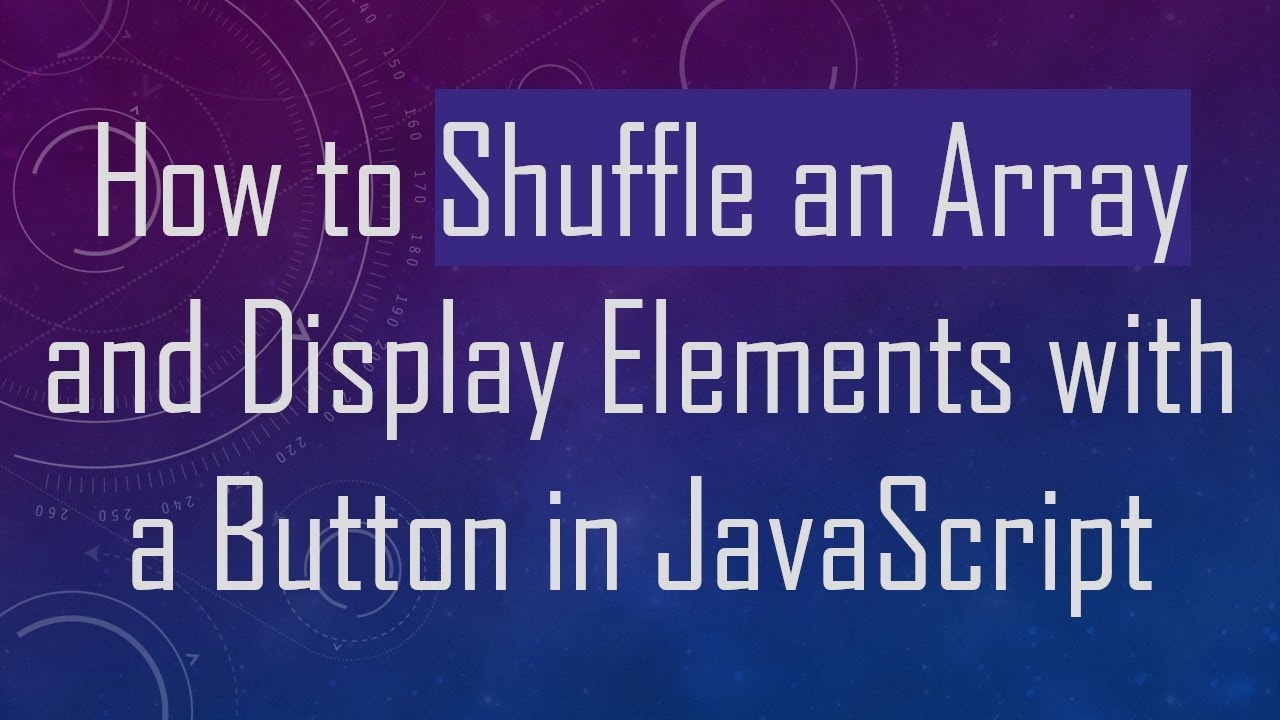
Показать описание
Discover how to shuffle an array in JavaScript and display each element on button clicks without repeats. Learn best practices for organizing your code!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Shuffle Array and Display with Button With No Repeats
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Introduction
If you've ever wanted to shuffle an array in JavaScript and display its elements one by one upon button clicks, this guide is for you! The task involves creating a user-friendly interface that allows users to reveal shuffled elements in a controlled way. In this walkthrough, we'll explain how to set up the HTML structure and implement the JavaScript functionality step-by-step.
Understanding the Problem
The objective here is to take an array (e.g., [x, y, z]), shuffle its contents, and ensure that upon each button click, a unique element from that shuffled array is displayed, without any repeats. Once all the items have been shown, users should be able to reset the shuffle and start the process over.
Solution Breakdown
HTML Structure
We first need a simple HTML layout that includes:
A paragraph to display the elements.
One button to show the next element.
Another button to reset the shuffle.
Here’s how to set up your HTML file:
[[See Video to Reveal this Text or Code Snippet]]
JavaScript Code Implementation
Next, we'll write a JavaScript function that can handle the shuffling of the array and manage the button click events.
Shuffling Function: This function will randomly rearrange the elements of the array.
Button Click Event: Set up an event listener for the "Next" button to display a new element from the shuffled array.
Reset Functionality: Allow users to reset the shuffle and start over.
Here’s the JavaScript code that achieves this:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
Array Initialization: We start with the variable arr containing the elements to be shuffled.
DOM References: We grab references to our display paragraph and buttons for interaction.
Shuffling Logic: The shuffle function implements the Fisher-Yates algorithm to ensure an even distribution of randomization.
Event Handling: We add event listeners to handle the clicking of the "Next" and "Reset" buttons, providing the desired functionality.
Conclusion
With this setup, you can now shuffle an array and display its elements one by one with button clicks in JavaScript. This kind of functionality could be useful for various applications, such as quizzes, games, or any scenario where a random yet controlled display of information is required.
Feel free to experiment with the code and customize the array content to fit your needs. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Shuffle Array and Display with Button With No Repeats
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Introduction
If you've ever wanted to shuffle an array in JavaScript and display its elements one by one upon button clicks, this guide is for you! The task involves creating a user-friendly interface that allows users to reveal shuffled elements in a controlled way. In this walkthrough, we'll explain how to set up the HTML structure and implement the JavaScript functionality step-by-step.
Understanding the Problem
The objective here is to take an array (e.g., [x, y, z]), shuffle its contents, and ensure that upon each button click, a unique element from that shuffled array is displayed, without any repeats. Once all the items have been shown, users should be able to reset the shuffle and start the process over.
Solution Breakdown
HTML Structure
We first need a simple HTML layout that includes:
A paragraph to display the elements.
One button to show the next element.
Another button to reset the shuffle.
Here’s how to set up your HTML file:
[[See Video to Reveal this Text or Code Snippet]]
JavaScript Code Implementation
Next, we'll write a JavaScript function that can handle the shuffling of the array and manage the button click events.
Shuffling Function: This function will randomly rearrange the elements of the array.
Button Click Event: Set up an event listener for the "Next" button to display a new element from the shuffled array.
Reset Functionality: Allow users to reset the shuffle and start over.
Here’s the JavaScript code that achieves this:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
Array Initialization: We start with the variable arr containing the elements to be shuffled.
DOM References: We grab references to our display paragraph and buttons for interaction.
Shuffling Logic: The shuffle function implements the Fisher-Yates algorithm to ensure an even distribution of randomization.
Event Handling: We add event listeners to handle the clicking of the "Next" and "Reset" buttons, providing the desired functionality.
Conclusion
With this setup, you can now shuffle an array and display its elements one by one with button clicks in JavaScript. This kind of functionality could be useful for various applications, such as quizzes, games, or any scenario where a random yet controlled display of information is required.
Feel free to experiment with the code and customize the array content to fit your needs. Happy coding!