filmov
tv
How to Reduce Duplicate Code in Python with Inner Functions
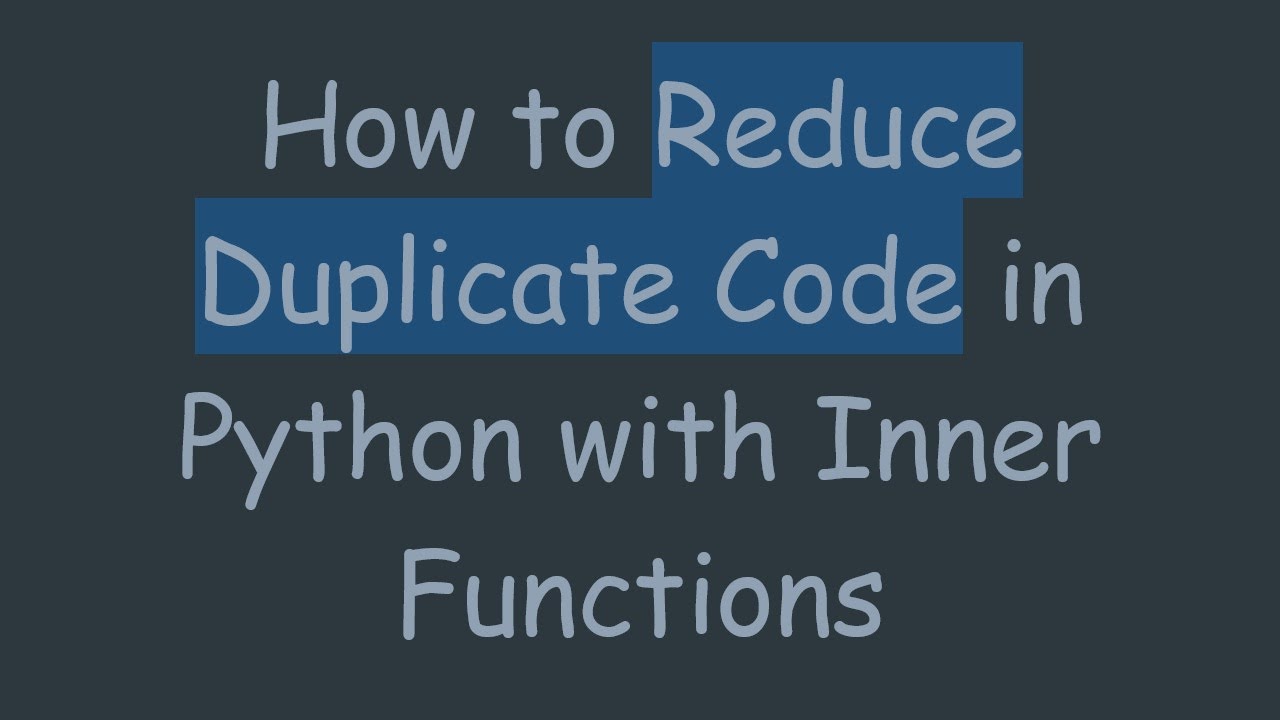
Показать описание
Learn how to efficiently manage duplicate code in Python by using inner functions to handle different object attribute names in Django.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Write a function to reduce duplicate code
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Reducing Duplicate Code in Python: Using Inner Functions
In software development, code duplication is a common issue that can lead to unnecessary complexity and maintenance challenges. This is especially true in Python programming when dealing with similar data structures or operations. If you're working with Django and have several similar operations, it may be time to consider a more efficient approach.
The Problem
Imagine you have two different querysets in your Django application: one for managers and another for team members. Both loop through their respective objects to fetch names, but due to different attribute names, code duplication arises. Here's a simplified version of your situation:
[[See Video to Reveal this Text or Code Snippet]]
Identifying the Issue
Both loops are doing essentially the same thing: formatting a name string. However, the attribute names differ (name for managers and member for team members). This creates redundancy, which is not only inefficient but also makes your code harder to maintain.
The Solution: Inner Functions
To resolve this, we can write an inner function that will abstract the name-fetching logic, allowing us to handle different object structures without repeating ourselves. Let's break down how to implement this.
Steps to Create the Inner Function
Define the Inner Function: This function will take the queryset as an argument and handle the logic for both managers and team members.
Check for Attributes: Inside the loop, the function will determine which attribute to look for based on the object's type.
Return the Formatted Name: After constructing the name string, the function can return that value.
Here's how the code looks:
[[See Video to Reveal this Text or Code Snippet]]
Advantages of This Approach
Using the inner function offers several benefits:
Code Maintainability: You change the name-fetching logic in one place rather than multiple locations.
Readability: Your loops become cleaner and easier to understand.
Reusability: The inner_fun can be reused for different querysets with minimal changes.
Conclusion
By implementing an inner function, you've significantly reduced duplicate code in your Python application. This not only streamlines your codebase but also enhances its maintainability and readability. As your application grows, such strategies will keep your code clean and efficient, making it easier for you and others to work with.
Whether you’re just starting with Python or refining your coding practices, remember the importance of eliminating redundancy. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Write a function to reduce duplicate code
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Reducing Duplicate Code in Python: Using Inner Functions
In software development, code duplication is a common issue that can lead to unnecessary complexity and maintenance challenges. This is especially true in Python programming when dealing with similar data structures or operations. If you're working with Django and have several similar operations, it may be time to consider a more efficient approach.
The Problem
Imagine you have two different querysets in your Django application: one for managers and another for team members. Both loop through their respective objects to fetch names, but due to different attribute names, code duplication arises. Here's a simplified version of your situation:
[[See Video to Reveal this Text or Code Snippet]]
Identifying the Issue
Both loops are doing essentially the same thing: formatting a name string. However, the attribute names differ (name for managers and member for team members). This creates redundancy, which is not only inefficient but also makes your code harder to maintain.
The Solution: Inner Functions
To resolve this, we can write an inner function that will abstract the name-fetching logic, allowing us to handle different object structures without repeating ourselves. Let's break down how to implement this.
Steps to Create the Inner Function
Define the Inner Function: This function will take the queryset as an argument and handle the logic for both managers and team members.
Check for Attributes: Inside the loop, the function will determine which attribute to look for based on the object's type.
Return the Formatted Name: After constructing the name string, the function can return that value.
Here's how the code looks:
[[See Video to Reveal this Text or Code Snippet]]
Advantages of This Approach
Using the inner function offers several benefits:
Code Maintainability: You change the name-fetching logic in one place rather than multiple locations.
Readability: Your loops become cleaner and easier to understand.
Reusability: The inner_fun can be reused for different querysets with minimal changes.
Conclusion
By implementing an inner function, you've significantly reduced duplicate code in your Python application. This not only streamlines your codebase but also enhances its maintainability and readability. As your application grows, such strategies will keep your code clean and efficient, making it easier for you and others to work with.
Whether you’re just starting with Python or refining your coding practices, remember the importance of eliminating redundancy. Happy coding!