filmov
tv
Inheritance In Java - Learn About One Of The Most Important Concepts In Object-oriented Programming!
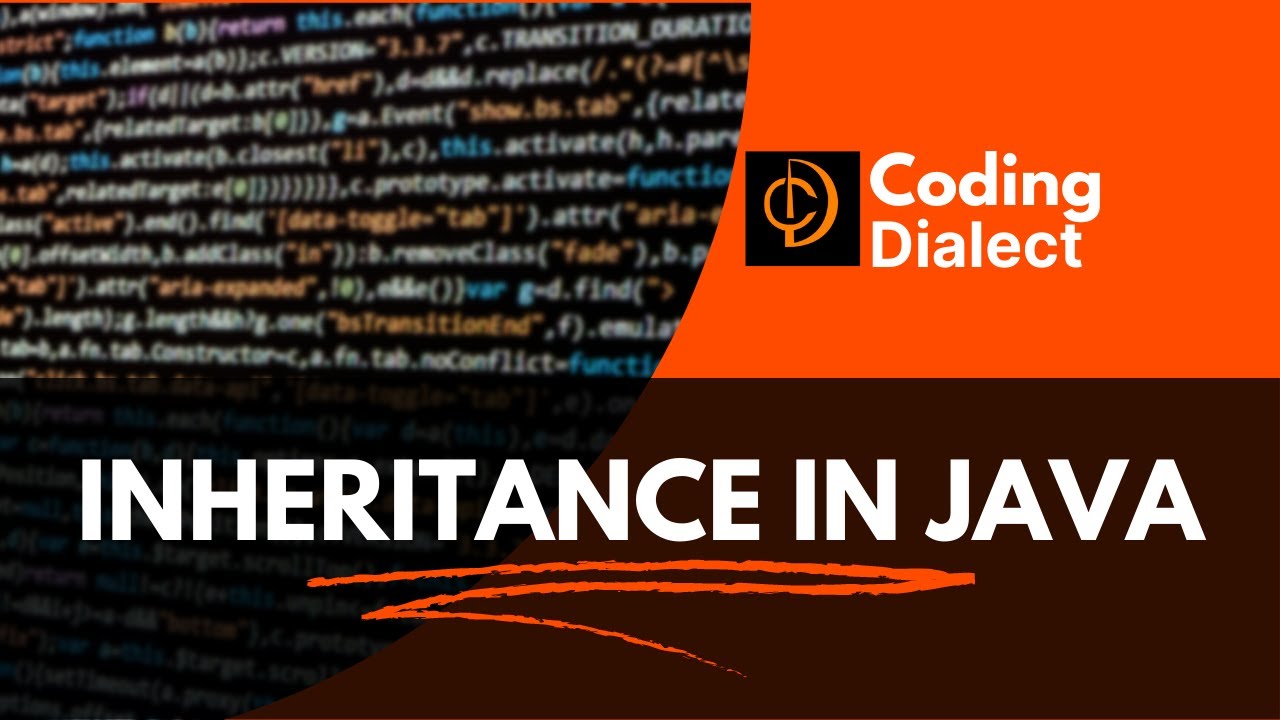
Показать описание
#JavaInheritance
#ObjectOrientedProgramming
#JavaOOP
#CodeReuse
#Superclass
#Subclass
#ExtendsKeyword
#OverrideMethod
#IsARelationship
#InheritanceHierarchy
#ClassHierarchy
#Polymorphism
#CodeOrganization
#JavaProgramming
#JavaDevelopment
Inheritance is a fundamental concept in object-oriented programming (OOP) and is a powerful mechanism provided by Java to create relationships between classes. It allows a class (called a subclass or derived class) to inherit the properties and behaviors of another class (called a superclass or base class). This enables code reuse and promotes a hierarchical organization of classes.
In Java, inheritance is achieved using the extends keyword. Here's a general syntax of how it's done:
java
Copy code
class Superclass {
// Fields, constructors, and methods of the superclass
}
class Subclass extends Superclass {
// Additional fields, constructors, and methods specific to the subclass
}
Let's take a practical example to illustrate inheritance:
java
Copy code
class Animal {
String name;
public Animal(String name) {
}
public void makeSound() {
}
}
class Dog extends Animal {
public Dog(String name) {
super(name);
}
@Override
public void makeSound() {
}
}
In this example, we have a superclass Animal with a field name and a method makeSound(). We then define a subclass Dog that extends the Animal class. The Dog class inherits the name field and the makeSound() method from the Animal class.
Note that the @Override annotation is used to indicate that the makeSound() method in the Dog class is overriding the method of the same name in the Animal class. This is optional but helps ensure that you are indeed overriding a method from the superclass.
Now, we can create objects of the Dog class and call its inherited methods:
java
Copy code
public class Main {
public static void main(String[] args) {
Dog dog = new Dog("Buddy");
}
}
When you run this code, you'll see the output:
rust
Copy code
Dog's name: Buddy
Woof! Woof!
As you can see, the Dog class inherited the name field from the Animal class, and its overridden makeSound() method produces a specific sound for dogs.
Inheritance provides a way to establish an "is-a" relationship, where a subclass is considered to be a specialized version of its superclass. It's crucial to use inheritance wisely, considering the principles of code reuse and maintaining a well-designed class hierarchy.
#ObjectOrientedProgramming
#JavaOOP
#CodeReuse
#Superclass
#Subclass
#ExtendsKeyword
#OverrideMethod
#IsARelationship
#InheritanceHierarchy
#ClassHierarchy
#Polymorphism
#CodeOrganization
#JavaProgramming
#JavaDevelopment
Inheritance is a fundamental concept in object-oriented programming (OOP) and is a powerful mechanism provided by Java to create relationships between classes. It allows a class (called a subclass or derived class) to inherit the properties and behaviors of another class (called a superclass or base class). This enables code reuse and promotes a hierarchical organization of classes.
In Java, inheritance is achieved using the extends keyword. Here's a general syntax of how it's done:
java
Copy code
class Superclass {
// Fields, constructors, and methods of the superclass
}
class Subclass extends Superclass {
// Additional fields, constructors, and methods specific to the subclass
}
Let's take a practical example to illustrate inheritance:
java
Copy code
class Animal {
String name;
public Animal(String name) {
}
public void makeSound() {
}
}
class Dog extends Animal {
public Dog(String name) {
super(name);
}
@Override
public void makeSound() {
}
}
In this example, we have a superclass Animal with a field name and a method makeSound(). We then define a subclass Dog that extends the Animal class. The Dog class inherits the name field and the makeSound() method from the Animal class.
Note that the @Override annotation is used to indicate that the makeSound() method in the Dog class is overriding the method of the same name in the Animal class. This is optional but helps ensure that you are indeed overriding a method from the superclass.
Now, we can create objects of the Dog class and call its inherited methods:
java
Copy code
public class Main {
public static void main(String[] args) {
Dog dog = new Dog("Buddy");
}
}
When you run this code, you'll see the output:
rust
Copy code
Dog's name: Buddy
Woof! Woof!
As you can see, the Dog class inherited the name field from the Animal class, and its overridden makeSound() method produces a specific sound for dogs.
Inheritance provides a way to establish an "is-a" relationship, where a subclass is considered to be a specialized version of its superclass. It's crucial to use inheritance wisely, considering the principles of code reuse and maintaining a well-designed class hierarchy.
Комментарии