filmov
tv
Mastering the Art of Creating Threads in Python: Techniques and Tips
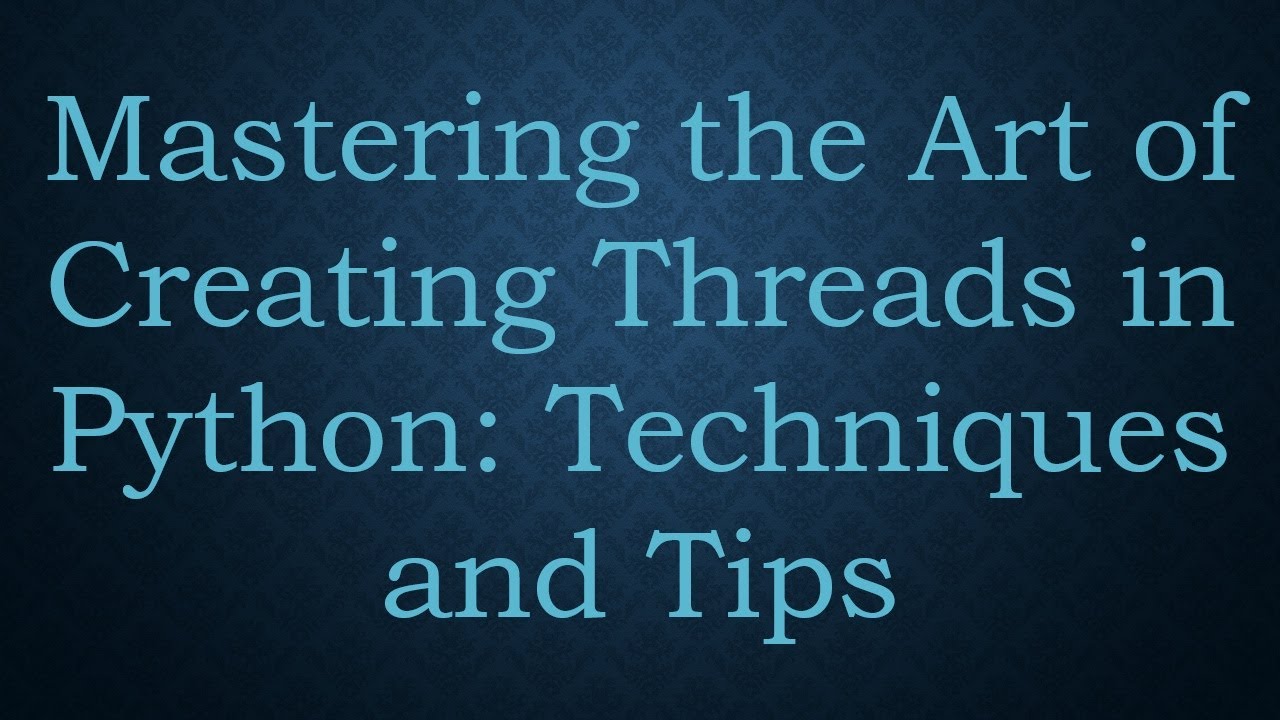
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn the essential techniques for creating threads in Python, from making single and multiple threads to effectively managing them within your applications. Unlock the power of concurrent programming today!
---
Mastering the Art of Creating Threads in Python: Techniques and Tips
Python's threading module allows developers to run multiple operations concurrently within a single process. By creating threads in Python, programmers can greatly enhance the performance and responsiveness of their applications. This guide delves into key methods for making threads in Python, offering practical insights into how to create new threads and manage multiple threads effectively.
Understanding Python Threads
In Python, a thread is an individual sequence of execution within the same application. Threads can run simultaneously, or concurrently, allowing programs to perform multiple tasks at the same time. This is particularly useful in applications that require I/O operations, such as reading and writing files or handling network requests.
Creating Threads with the threading Module
To leverage threading in Python, we use the threading module. The following is a basic example of how to create new threads in Python:
[[See Video to Reveal this Text or Code Snippet]]
In this example, the print_numbers function is executed in a separate thread, running concurrently with the main program.
Making Multiple Threads in Python
When dealing with tasks that can be split into independent units of work, creating multiple threads in Python can significantly improve performance. Here's how you can create multiple threads:
[[See Video to Reveal this Text or Code Snippet]]
This example demonstrates the creation of five worker threads, each executing the worker function with a different argument.
Best Practices for Thread Management
Creating multiple threads in Python may sometimes introduce complexity. Here are some best practices to ensure efficient thread management:
Thread Synchronization: Use locks (threading.Lock) to prevent race conditions.
Resource Management: Ensure that threads are properly terminated and resources are released.
Example of using a lock:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Creating threads in Python can greatly enhance the operations of your applications by allowing concurrent execution. Whether you're making single or multiple threads, understanding thread management is crucial. By following the techniques and best practices outlined in this guide, you'll be well-equipped to effectively implement threading in your Python projects.
Unlock the potential of concurrent programming today and elevate your applications to the next level!
---
Summary: Learn the essential techniques for creating threads in Python, from making single and multiple threads to effectively managing them within your applications. Unlock the power of concurrent programming today!
---
Mastering the Art of Creating Threads in Python: Techniques and Tips
Python's threading module allows developers to run multiple operations concurrently within a single process. By creating threads in Python, programmers can greatly enhance the performance and responsiveness of their applications. This guide delves into key methods for making threads in Python, offering practical insights into how to create new threads and manage multiple threads effectively.
Understanding Python Threads
In Python, a thread is an individual sequence of execution within the same application. Threads can run simultaneously, or concurrently, allowing programs to perform multiple tasks at the same time. This is particularly useful in applications that require I/O operations, such as reading and writing files or handling network requests.
Creating Threads with the threading Module
To leverage threading in Python, we use the threading module. The following is a basic example of how to create new threads in Python:
[[See Video to Reveal this Text or Code Snippet]]
In this example, the print_numbers function is executed in a separate thread, running concurrently with the main program.
Making Multiple Threads in Python
When dealing with tasks that can be split into independent units of work, creating multiple threads in Python can significantly improve performance. Here's how you can create multiple threads:
[[See Video to Reveal this Text or Code Snippet]]
This example demonstrates the creation of five worker threads, each executing the worker function with a different argument.
Best Practices for Thread Management
Creating multiple threads in Python may sometimes introduce complexity. Here are some best practices to ensure efficient thread management:
Thread Synchronization: Use locks (threading.Lock) to prevent race conditions.
Resource Management: Ensure that threads are properly terminated and resources are released.
Example of using a lock:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Creating threads in Python can greatly enhance the operations of your applications by allowing concurrent execution. Whether you're making single or multiple threads, understanding thread management is crucial. By following the techniques and best practices outlined in this guide, you'll be well-equipped to effectively implement threading in your Python projects.
Unlock the potential of concurrent programming today and elevate your applications to the next level!