filmov
tv
How to Create a Nested Dictionary from Directory Files in Python with Ease
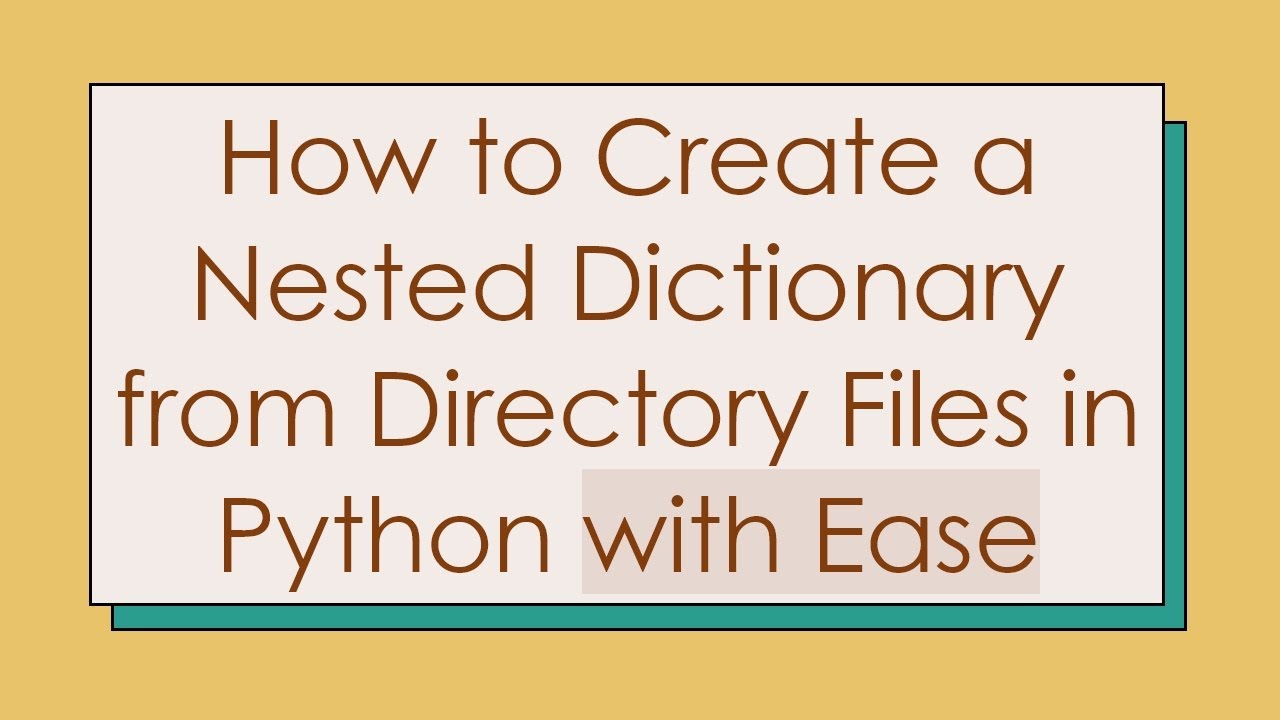
Показать описание
Learn how to efficiently create a nested dictionary from directory files in Python, allowing for better data organization and accessibility.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Create nested dictionary from directory
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Creating a Nested Dictionary from Directory Files in Python
The Problem
You have a directory filled with audio files, named in a fashion where each segment (e.g., Max, Toto, Valtteri) is followed by a sequential number (_1, _2, _3). You wish to create a nested dictionary that groups these files under their respective names, linking each with its file path. Here’s the desired output you want:
[[See Video to Reveal this Text or Code Snippet]]
The Initial Attempt
You wrote code to process the directory and nested this data, but encountered a key overwriting issue. Your original approach looked something like this:
[[See Video to Reveal this Text or Code Snippet]]
The outcome was not what you intended, as subsequent files were overwriting previous entries.
Understanding the Issue
The problem lies in the way you're assigning new values to the dictionary. Each time you loop over the files, new entries are replacing the previous ones under the same keys because of the line dict[key] = {x[:-4]: {'path': f'database/{x}'}}. This resets the value for key, removing any existing nested structure.
The Solution
To overcome this, instead of reassigning the dictionary keys, you should use the update method of the dictionary to add new entries. Here’s how you can fix your code:
Initialize an Empty Dictionary
Iterate Over the Files
Build the Nested Structure Using update
Here’s the corrected version of your code:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Solution:
The first loop checks if the main key (name) exists in the dictionary; if not, it initializes it as an empty dictionary.
The update method then adds the file names as keys, preserving all previous entries.
Conclusion
Creating a nested dictionary in Python from files in a directory is a practical way to organize audio files systematically. By understanding the pitfalls of dictionary manipulation and utilizing the update method, you can efficiently create the desired data structure without overwriting previous entries. With this approach, you can enjoy a well-organized dataset that is easier to manage and access.
For any further questions or clarifications, feel free to reach out! Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Create nested dictionary from directory
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Creating a Nested Dictionary from Directory Files in Python
The Problem
You have a directory filled with audio files, named in a fashion where each segment (e.g., Max, Toto, Valtteri) is followed by a sequential number (_1, _2, _3). You wish to create a nested dictionary that groups these files under their respective names, linking each with its file path. Here’s the desired output you want:
[[See Video to Reveal this Text or Code Snippet]]
The Initial Attempt
You wrote code to process the directory and nested this data, but encountered a key overwriting issue. Your original approach looked something like this:
[[See Video to Reveal this Text or Code Snippet]]
The outcome was not what you intended, as subsequent files were overwriting previous entries.
Understanding the Issue
The problem lies in the way you're assigning new values to the dictionary. Each time you loop over the files, new entries are replacing the previous ones under the same keys because of the line dict[key] = {x[:-4]: {'path': f'database/{x}'}}. This resets the value for key, removing any existing nested structure.
The Solution
To overcome this, instead of reassigning the dictionary keys, you should use the update method of the dictionary to add new entries. Here’s how you can fix your code:
Initialize an Empty Dictionary
Iterate Over the Files
Build the Nested Structure Using update
Here’s the corrected version of your code:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Solution:
The first loop checks if the main key (name) exists in the dictionary; if not, it initializes it as an empty dictionary.
The update method then adds the file names as keys, preserving all previous entries.
Conclusion
Creating a nested dictionary in Python from files in a directory is a practical way to organize audio files systematically. By understanding the pitfalls of dictionary manipulation and utilizing the update method, you can efficiently create the desired data structure without overwriting previous entries. With this approach, you can enjoy a well-organized dataset that is easier to manage and access.
For any further questions or clarifications, feel free to reach out! Happy coding!