filmov
tv
Fix the Uncaught TypeError: Cannot set property 'data' of undefined Error in TypeScript!
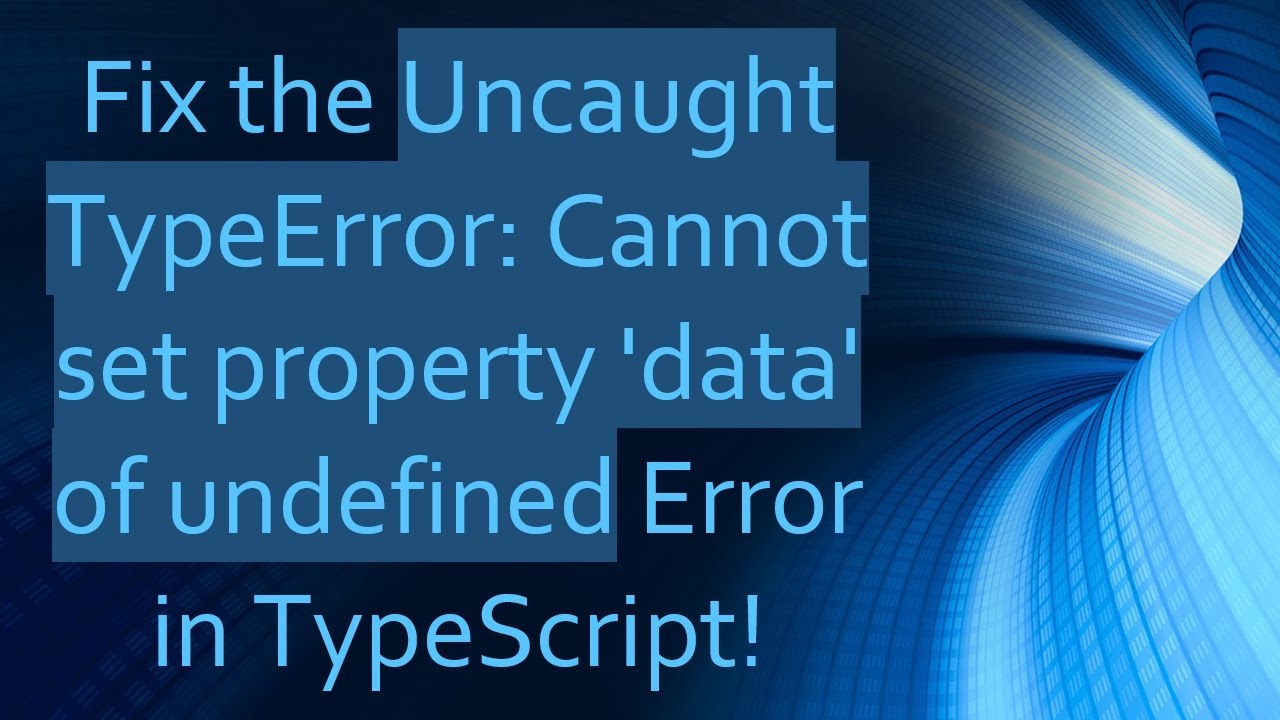
Показать описание
Learn how to solve the common TypeScript error when assigning values to an array by iterating through a JSON. Simplify your code to avoid the `undefined` issue and ensure smooth data assignment.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Unable to assign value for array
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Solving the Uncaught TypeError: Cannot set property 'data' of undefined Error in TypeScript
When working with TypeScript, you may encounter various errors that can hinder your progress. One common issue developers face is the error message that states: Uncaught TypeError: Cannot set property 'data' of undefined. This error usually occurs when you attempt to access or modify properties of an array element that may not exist yet. In this guide, we’ll discuss the underlying cause of this error and demonstrate effective solutions to fix it.
Understanding the Problem
Let's break down the scenario. You are utilizing a TypeScript functional component to assign values to an array based on data from a JSON file. Although you successfully retrieve the data, you surface an error when attempting to assign property values to an element of an array that hasn't been initialized.
Here’s a snippet from the problematic code:
[[See Video to Reveal this Text or Code Snippet]]
The core of the problem lies in the fact that you're accessing val[j] and expecting it to exist. However, if the index j exceeds the current length of the array val, you will encounter the TypeError because you're trying to modify an undefined object.
Step-by-Step Solution
Option 1: Initialize Objects for Undefined Indices
To avoid hitting undefined elements, you can modify your code to check if val[j] exists. If not, create a new object reference for that index.
Here’s how you can implement the fix:
[[See Video to Reveal this Text or Code Snippet]]
Option 2: Utilize Nullish Coalescing
If your code supports Nullish Coalescing, you can simplify the object assignment even further. This syntax allows you to provide a fallback for undefined values easily:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Encountering the error Uncaught TypeError: Cannot set property 'data' of undefined in TypeScript can be frustrating, but with a clear understanding of the issue and how to initialize your arrays correctly, you can resolve it effectively. By ensuring that your array elements are properly defined before accessing their properties, you will enhance the robustness of your code and reduce runtime errors.
If you frequently work with dynamic data from JSON files, consider employing these strategies to maintain a smooth development experience. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Unable to assign value for array
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Solving the Uncaught TypeError: Cannot set property 'data' of undefined Error in TypeScript
When working with TypeScript, you may encounter various errors that can hinder your progress. One common issue developers face is the error message that states: Uncaught TypeError: Cannot set property 'data' of undefined. This error usually occurs when you attempt to access or modify properties of an array element that may not exist yet. In this guide, we’ll discuss the underlying cause of this error and demonstrate effective solutions to fix it.
Understanding the Problem
Let's break down the scenario. You are utilizing a TypeScript functional component to assign values to an array based on data from a JSON file. Although you successfully retrieve the data, you surface an error when attempting to assign property values to an element of an array that hasn't been initialized.
Here’s a snippet from the problematic code:
[[See Video to Reveal this Text or Code Snippet]]
The core of the problem lies in the fact that you're accessing val[j] and expecting it to exist. However, if the index j exceeds the current length of the array val, you will encounter the TypeError because you're trying to modify an undefined object.
Step-by-Step Solution
Option 1: Initialize Objects for Undefined Indices
To avoid hitting undefined elements, you can modify your code to check if val[j] exists. If not, create a new object reference for that index.
Here’s how you can implement the fix:
[[See Video to Reveal this Text or Code Snippet]]
Option 2: Utilize Nullish Coalescing
If your code supports Nullish Coalescing, you can simplify the object assignment even further. This syntax allows you to provide a fallback for undefined values easily:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Encountering the error Uncaught TypeError: Cannot set property 'data' of undefined in TypeScript can be frustrating, but with a clear understanding of the issue and how to initialize your arrays correctly, you can resolve it effectively. By ensuring that your array elements are properly defined before accessing their properties, you will enhance the robustness of your code and reduce runtime errors.
If you frequently work with dynamic data from JSON files, consider employing these strategies to maintain a smooth development experience. Happy coding!