filmov
tv
Map method in JavaScript | ECMAScript 5 -#50 @Everyday-Be-Coding
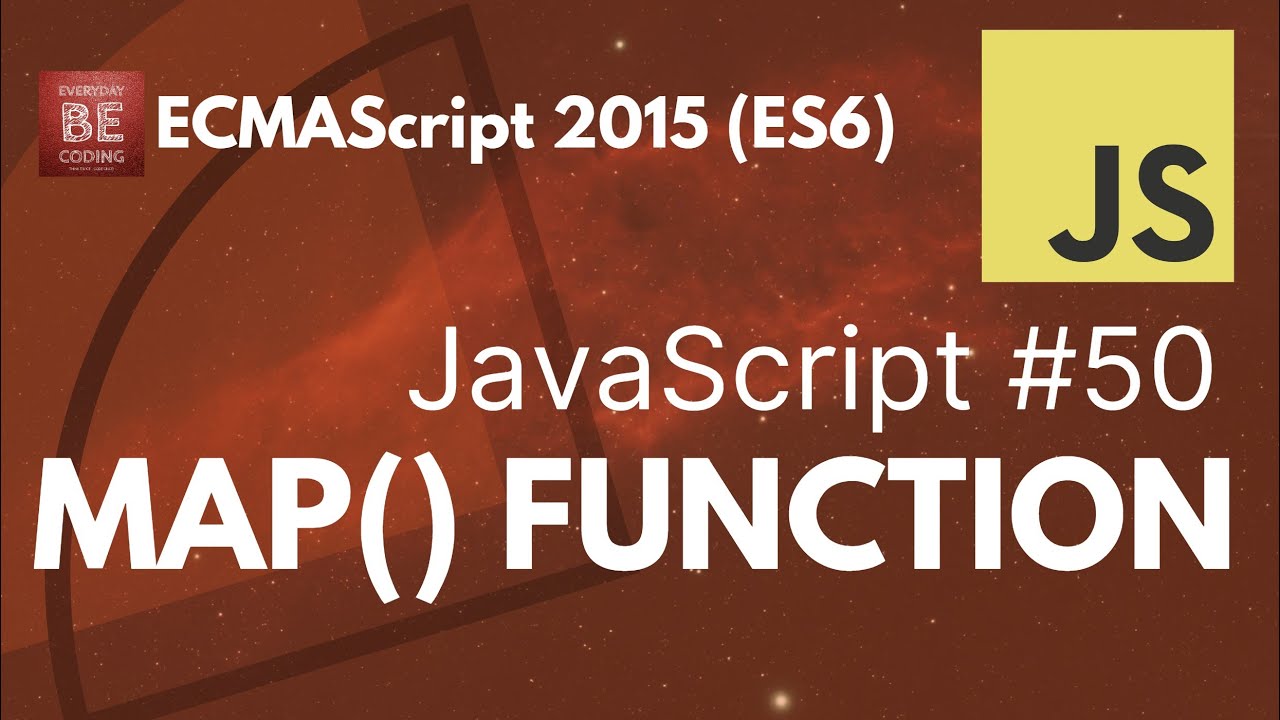
Показать описание
The map() method in JavaScript is a powerful tool for transforming elements in an array. Here's a detailed explanation along with examples and outputs:
Description:
The map() method creates a new array by calling a provided function on every element in the original array. It returns a new array with the results of the provided function applied to each element.
Example 1:
Description: Doubling each number in an array.
JavaScript code:
const numbers = [1, 2, 3, 4, 5];
return number * 2;
});
Output:
[2, 4, 6, 8, 10]
Example 2:
Description: Mapping array of strings to uppercase.
JavaScript code:
const fruits = ['apple', 'banana', 'cherry'];
});
Output:
['APPLE', 'BANANA', 'CHERRY']
Example 3:
Description: Mapping array of objects to extract specific property.
JavaScript code:
const persons = [
{ name: 'Alice', age: 30 },
{ name: 'Bob', age: 25 },
{ name: 'Charlie', age: 35 }
];
});
Output:
['Alice', 'Bob', 'Charlie']
Example 4:
Description: Mapping array of numbers to calculate square roots.
JavaScript Code :
const numbers = [4, 9, 16, 25];
});
Output:
[2, 3, 4, 5]
In each example, the map() method iterates over each element in the original array, applies the provided function to each element, and returns a new array with the transformed values. This makes map() a versatile and powerful method for array manipulation in JavaScript.
Advantages:
Concise Syntax: The map() method provides a concise syntax for transforming each element of an array without the need for explicit iteration.
Immutable: The map() method does not mutate the original array. It creates a new array with the transformed values, leaving the original array unchanged.
Functional Programming: It promotes a functional programming style by allowing you to apply a function to each element of an array, which can lead to cleaner and more declarative code.
Readability: Using map() often leads to more readable code compared to traditional loop constructs, especially for simple transformations.
Disadvantages:
Performance Overhead: While map() provides a convenient way to transform arrays, it may introduce a performance overhead compared to traditional loops, especially for large arrays or complex transformations.
Not Always Appropriate: In some cases, using map() may not be the most appropriate choice, especially if the transformation logic is complex or if side effects are involved.
Requires Function Expression: The callback function passed to map() must be a function expression, which can sometimes lead to less readable code, especially for longer or more complex transformation logic.
May Not Support Older Browsers: While map() is supported in modern JavaScript environments, older browsers may not fully support it, which can be a limitation if you need to support a wide range of browsers.
Summery
Overall, the map() method is a powerful and convenient tool for transforming arrays in JavaScript, but it's important to consider its advantages and disadvantages when deciding whether to use it in a particular context.
JavaScript arrays
Functional programming
Array methods
Data transformation
Array manipulation
JavaScript programming
ES6 features
Functional programming in JavaScript
JavaScript map function
JavaScript tutorials
#JavaScriptArrays
#ArrayMethods
#JavaScriptFunctions
#DataManipulation
#ProgrammingTutorials
#JavaScriptTips
#CodingEducation
#WebDevelopment
#LearnJavaScript
#CodeNewbie
Chapter :
00:00 Introduction to map() method
00:15 Syntax and descriptions
00:51 Example 1 - Doubling each number in an array
01:12 Example 1 - Mapping array of strings to uppercase
01:25 Example 1 - Mapping array of objects to extract specific property
01:35 Example 4 - Mapping array of numbers to calculate square roots
01:49 Advantages
02:08 Disadvantages
02:36 Summery
Thank you for watching this video
EVERYDAY BE CODING