filmov
tv
Make a JSON string from C# Object in WebAPI ASP NET MVC 5 using JSON NET framework | Part 10
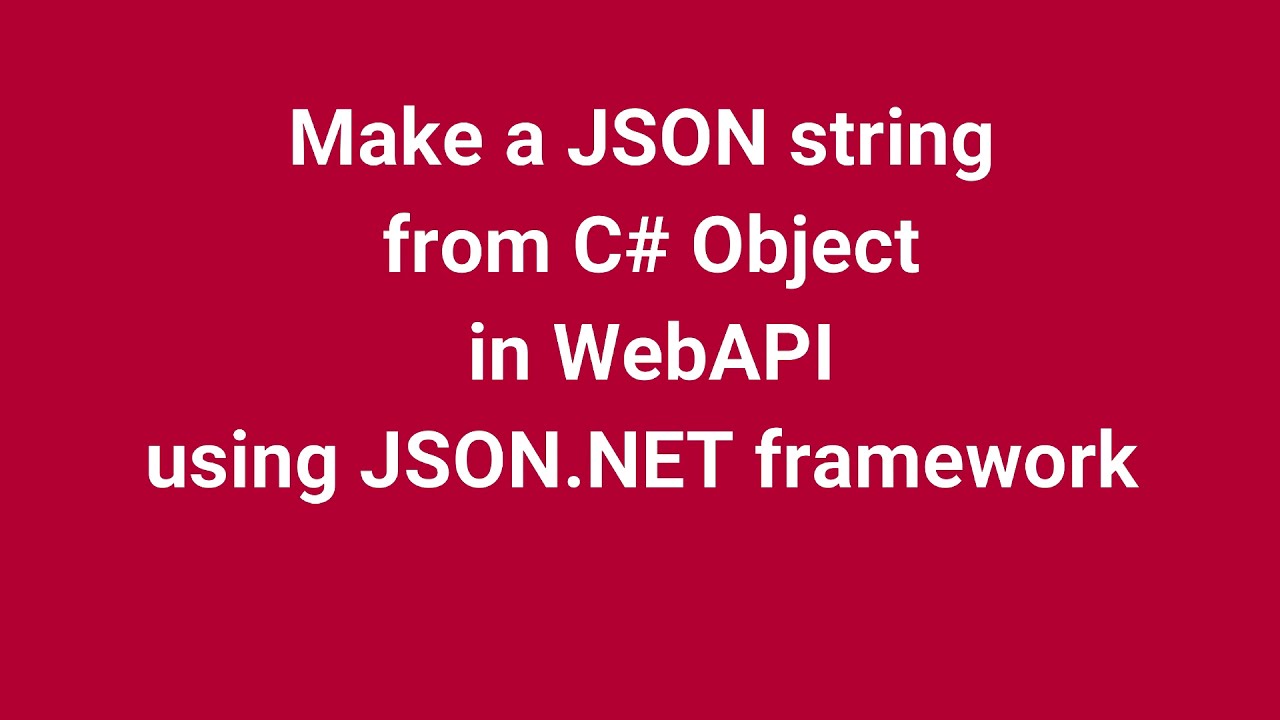
Показать описание
Here We will learn, How to make a JSON string from C# Object in WebAPI (ASP.NET MVC 5)
using JSON.NET framework
Requirement: Visual studio 2015, MVC 5, JSON.NET, SQL Server 2012 or higher version
Here we understand three type of example, static(single row and multiple rows) and dynamic(from database single row or multiple rows)
Example 1:
public class Employee
{
public int empid { get; set; }
public string Name { get; set; }
public decimal salary { get; set; }
}
Set the value:
employee obj = new employee();
obj.Name = "Adam";
Convert into JSON string:
var jsonString = Newtonsoft.Json.JsonConvert.SerializeObject(obj);
Result:
{
"empid": 106,
"Name": "Breylin",
"salary": 20000
}
Example 2:
[Route("getemployeelist")]
[HttpGet]
public IHttpActionResult getEmployeelist()
{
List put here less sign employee put here grater sign obj = new List put here less sign employee put here greatersign ();
{
empid = 101,
Name = "Adam",
salary = 2000
});
obj.Add(new employee
{
empid = 102,
Name = "Adam Mar",
salary = 25000
});
var jsonString = Newtonsoft.Json.JsonConvert.SerializeObject(obj);
Result 2--
"Employee": [
{
"empid": 106,
"Name": "Breylin",
"salary": 20000
},
{
"empid": 107,
"Name": "Breylin A",
"salary": 20000
}]
Example 3:
[Route("getemployeelistfromDataBase")]
[HttpGet]
public IHttpActionResult getemployeelistfromDataBase()
{
SampleEntities db = new SampleEntities();
var jsonString = JsonConvert.SerializeObject(result);
//return Ok(new { Employee = result });
}
Result 3---
{
"Employee": [
{
"empid": 106,
"Name": "Breylin",
"salary": 20000
},
{
"empid": 107,
"Name": "Breylin A",
"salary": 20000
},
{
"empid": 110,
"Name": "Breylin A",
"salary": 20000
},
{
"empid": 150,
"Name": "Ame",
"salary": 3000
}
]
}
using JSON.NET framework
Requirement: Visual studio 2015, MVC 5, JSON.NET, SQL Server 2012 or higher version
Here we understand three type of example, static(single row and multiple rows) and dynamic(from database single row or multiple rows)
Example 1:
public class Employee
{
public int empid { get; set; }
public string Name { get; set; }
public decimal salary { get; set; }
}
Set the value:
employee obj = new employee();
obj.Name = "Adam";
Convert into JSON string:
var jsonString = Newtonsoft.Json.JsonConvert.SerializeObject(obj);
Result:
{
"empid": 106,
"Name": "Breylin",
"salary": 20000
}
Example 2:
[Route("getemployeelist")]
[HttpGet]
public IHttpActionResult getEmployeelist()
{
List put here less sign employee put here grater sign obj = new List put here less sign employee put here greatersign ();
{
empid = 101,
Name = "Adam",
salary = 2000
});
obj.Add(new employee
{
empid = 102,
Name = "Adam Mar",
salary = 25000
});
var jsonString = Newtonsoft.Json.JsonConvert.SerializeObject(obj);
Result 2--
"Employee": [
{
"empid": 106,
"Name": "Breylin",
"salary": 20000
},
{
"empid": 107,
"Name": "Breylin A",
"salary": 20000
}]
Example 3:
[Route("getemployeelistfromDataBase")]
[HttpGet]
public IHttpActionResult getemployeelistfromDataBase()
{
SampleEntities db = new SampleEntities();
var jsonString = JsonConvert.SerializeObject(result);
//return Ok(new { Employee = result });
}
Result 3---
{
"Employee": [
{
"empid": 106,
"Name": "Breylin",
"salary": 20000
},
{
"empid": 107,
"Name": "Breylin A",
"salary": 20000
},
{
"empid": 110,
"Name": "Breylin A",
"salary": 20000
},
{
"empid": 150,
"Name": "Ame",
"salary": 3000
}
]
}
Комментарии