filmov
tv
Python Exercises for Beginners: if elif else example | Python Tutorial for Beginners
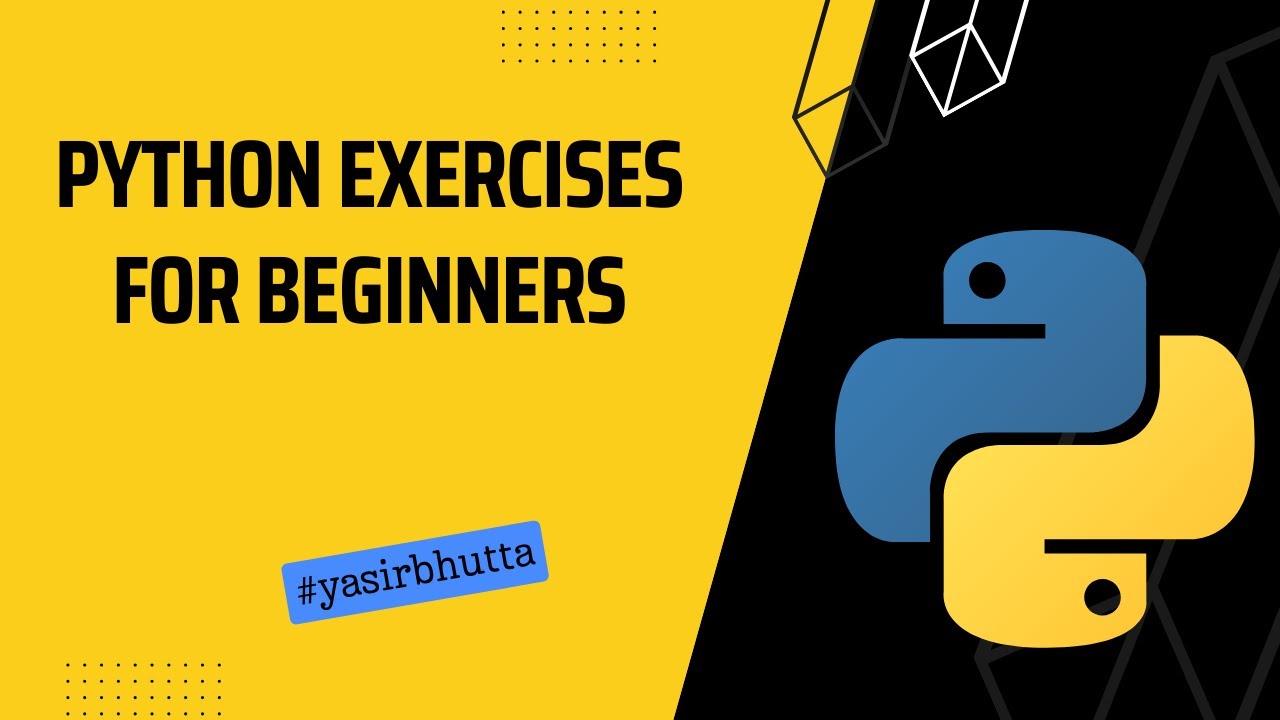
Показать описание
@yasirbhutta Welcome to our Python programming tutorial for beginners! In this video, we’ll walk you through the popular FizzBuzz coding challenge, which is a great exercise for mastering loops, conditionals, and basic arithmetic operations in Python.
What You'll Learn:
- How to use a for loop in Python.
- Understanding the modulus (%) operator to check divisibility.
- Using if, elif, and else statements to implement conditional logic.
- Breaking down the FizzBuzz problem in simple steps.
The FizzBuzz challenge is often used in coding interviews and beginner courses to sharpen your programming skills. We will explain every line of the code so you can understand the logic behind it, making it easy for you to apply in future projects.
**Code:**
n = int(input("Enter number:"))
for i in range(1, n + 1):
if i % 3 == 0 and i % 5 == 0:
print("FizzBuzz")
elif i % 3 == 0:
print("Fizz")
elif i % 5 == 0:
print("Buzz")
else:
print(i)
**Explanation: **
### 1. `n = int(input("Enter number:"))`
- **`input("Enter number:")`**: This prompts the user to enter a value. Whatever the user enters is initially treated as a string.
- **`int()`**: This converts the user input from a string to an integer.
- **`n =`**: The converted integer value is then assigned to the variable `n`.
### 2. `for i in range(1, n + 1):`
- **`for i in`**: This starts a `for` loop, which is used to iterate over a sequence of numbers.
- **`range(1, n + 1)`**: The `range()` function generates a sequence of numbers starting from `1` up to `n` (inclusive). The `n + 1` ensures that the loop includes `n` itself.
- **`i`**: During each iteration, the loop variable `i` takes on one of the values from the range (starting from `1` to `n`).
### 3. `if i % 3 == 0 and i % 5 == 0:`
- **`i % 3 == 0`**: This checks if `i` is divisible by `3`. The `%` operator returns the remainder of the division, so `i % 3` gives the remainder when `i` is divided by `3`. If the remainder is `0`, it means `i` is divisible by `3`.
- **`i % 5 == 0`**: Similarly, this checks if `i` is divisible by `5`.
- **`and`**: This logical operator ensures that both conditions (divisibility by `3` and `5`) must be true. If both are true, the next line of code will be executed.
### 4. `print("FizzBuzz")`
- If `i` is divisible by both `3` and `5`, this line is executed, and the string `"FizzBuzz"` is printed to the screen.
### 5. `elif i % 3 == 0:`
- **`elif`**: This stands for "else if" and is checked only if the previous `if` condition was false.
- **`i % 3 == 0`**: This checks if `i` is divisible by `3` (but not necessarily by `5`). If true, the next line will be executed.
### 6. `print("Fizz")`
- If `i` is divisible by `3` but not by `5`, this line prints `"Fizz"`.
### 7. `elif i % 5 == 0:`
- This checks if `i` is divisible by `5` (but not by `3`). If true, the next line will be executed.
### 8. `print("Buzz")`
- If `i` is divisible by `5` but not by `3`, this line prints `"Buzz"`.
### 9. `else:`
- This block is executed only if none of the previous conditions (divisibility by `3`, `5`, or both) are met.
### 10. `print(i)`
- If `i` is not divisible by `3` or `5`, the value of `i` is printed.
### Example Execution:
- If the user enters `n = 15`, the output would be:
```
1
2
Fizz
4
Buzz
Fizz
7
8
Fizz
Buzz
11
Fizz
13
14
FizzBuzz
```
#coding #pythonlearning #pythonprogramming #programming
What You'll Learn:
- How to use a for loop in Python.
- Understanding the modulus (%) operator to check divisibility.
- Using if, elif, and else statements to implement conditional logic.
- Breaking down the FizzBuzz problem in simple steps.
The FizzBuzz challenge is often used in coding interviews and beginner courses to sharpen your programming skills. We will explain every line of the code so you can understand the logic behind it, making it easy for you to apply in future projects.
**Code:**
n = int(input("Enter number:"))
for i in range(1, n + 1):
if i % 3 == 0 and i % 5 == 0:
print("FizzBuzz")
elif i % 3 == 0:
print("Fizz")
elif i % 5 == 0:
print("Buzz")
else:
print(i)
**Explanation: **
### 1. `n = int(input("Enter number:"))`
- **`input("Enter number:")`**: This prompts the user to enter a value. Whatever the user enters is initially treated as a string.
- **`int()`**: This converts the user input from a string to an integer.
- **`n =`**: The converted integer value is then assigned to the variable `n`.
### 2. `for i in range(1, n + 1):`
- **`for i in`**: This starts a `for` loop, which is used to iterate over a sequence of numbers.
- **`range(1, n + 1)`**: The `range()` function generates a sequence of numbers starting from `1` up to `n` (inclusive). The `n + 1` ensures that the loop includes `n` itself.
- **`i`**: During each iteration, the loop variable `i` takes on one of the values from the range (starting from `1` to `n`).
### 3. `if i % 3 == 0 and i % 5 == 0:`
- **`i % 3 == 0`**: This checks if `i` is divisible by `3`. The `%` operator returns the remainder of the division, so `i % 3` gives the remainder when `i` is divided by `3`. If the remainder is `0`, it means `i` is divisible by `3`.
- **`i % 5 == 0`**: Similarly, this checks if `i` is divisible by `5`.
- **`and`**: This logical operator ensures that both conditions (divisibility by `3` and `5`) must be true. If both are true, the next line of code will be executed.
### 4. `print("FizzBuzz")`
- If `i` is divisible by both `3` and `5`, this line is executed, and the string `"FizzBuzz"` is printed to the screen.
### 5. `elif i % 3 == 0:`
- **`elif`**: This stands for "else if" and is checked only if the previous `if` condition was false.
- **`i % 3 == 0`**: This checks if `i` is divisible by `3` (but not necessarily by `5`). If true, the next line will be executed.
### 6. `print("Fizz")`
- If `i` is divisible by `3` but not by `5`, this line prints `"Fizz"`.
### 7. `elif i % 5 == 0:`
- This checks if `i` is divisible by `5` (but not by `3`). If true, the next line will be executed.
### 8. `print("Buzz")`
- If `i` is divisible by `5` but not by `3`, this line prints `"Buzz"`.
### 9. `else:`
- This block is executed only if none of the previous conditions (divisibility by `3`, `5`, or both) are met.
### 10. `print(i)`
- If `i` is not divisible by `3` or `5`, the value of `i` is printed.
### Example Execution:
- If the user enters `n = 15`, the output would be:
```
1
2
Fizz
4
Buzz
Fizz
7
8
Fizz
Buzz
11
Fizz
13
14
FizzBuzz
```
#coding #pythonlearning #pythonprogramming #programming