filmov
tv
Understanding Why Your JavaScript Loop Doesn't Display Intermediate Results
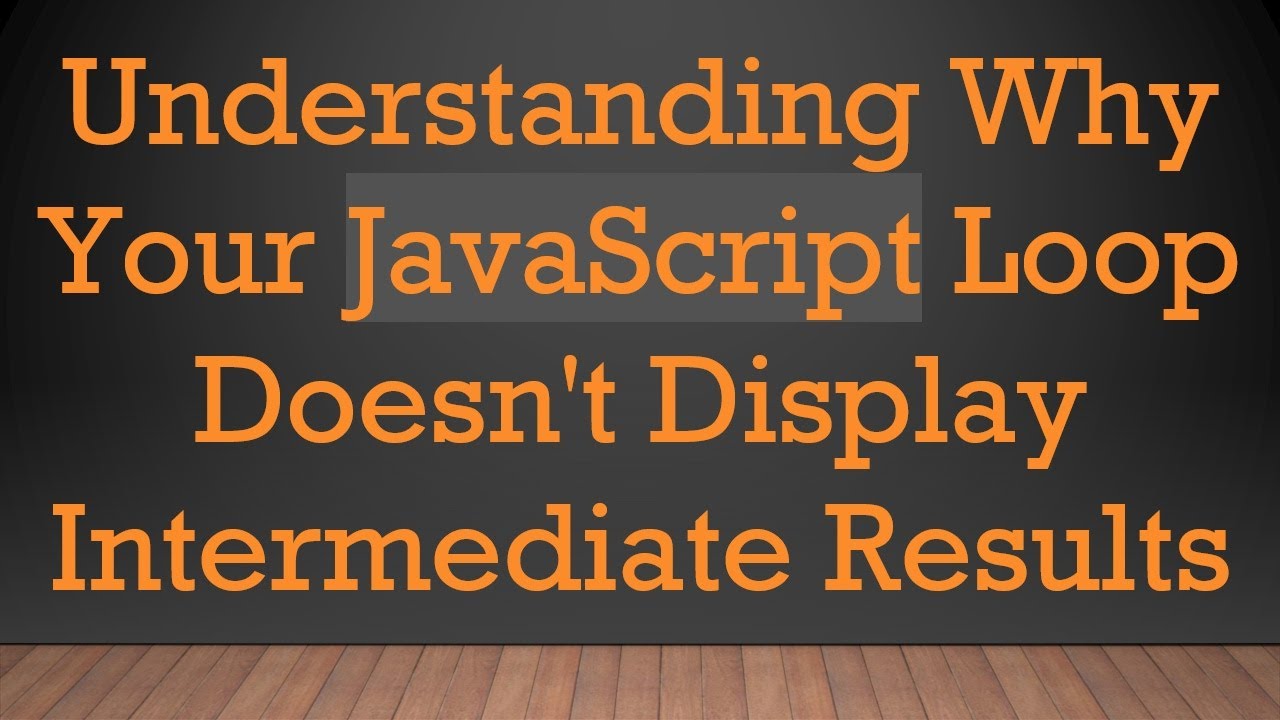
Показать описание
Learn why a `JavaScript` loop fails to show intermediate values in an HTML text input and how to fix this issue for better user experience.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Tracking a loop in JavaScript doesn't seem to work?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Why Your JavaScript Loop Doesn't Display Intermediate Results
When you're working with loops in JavaScript, it can be frustrating if you expect to see live updates as the loop progresses, but instead, you only get the final result. If you've tried something similar to the below code and are puzzled why it behaves in this way, you're not alone! Let's break down the issue and explore the solution together.
The Problem: Code Behavior
In the provided code snippet excerpts, the intent is to display the current index of a loop in a text input element. However, when running the loop:
[[See Video to Reveal this Text or Code Snippet]]
You would only see the message "End" in the text element after the loop has finished executing. So, what gives?
Understanding Synchronous Execution
The key reason you're only seeing the end message is that JavaScript executes code synchronously, which means it runs code in sequence, one after the other. Here's what this means in your context:
Blocking Execution: The loop runs and blocks the UI thread until it completes. While it's running, the browser doesn't get a chance to update the UI.
Final Output Only: Since the text input gets updated only after the loop finishes, it reflects only the last value assigned (in this case, always "End").
Possible Solutions
To resolve this and see the intermediate values as the loop progresses, you can use asynchronous programming techniques. Below are a couple of approaches.
1. Use setTimeout
One simple solution is to use setTimeout to break the loop into smaller chunks. This allows the browser to render changes in the UI while the loop processes:
[[See Video to Reveal this Text or Code Snippet]]
Here, setTimeout(update, 0) tells JavaScript to wait until the current call stack is clear before executing the next iteration, allowing the UI to update.
2. Use Promises and async/await
If you are comfortable with modern JavaScript, you could also use Promises along with async/await. Here's how that could look:
[[See Video to Reveal this Text or Code Snippet]]
The requestAnimationFrame function allows the browser to perform updates, thus preventing blocking execution.
Conclusion
Now you have a clearer understanding of why your loop didn't yield the results you wanted and how to resolve it. By introducing asynchronous behavior through techniques like setTimeout or using async/await, you can create more responsive and dynamic applications. Moving forward with this knowledge will help enhance the user experience in your JavaScript applications!
If you have further questions or need additional clarification, feel free to drop a comment below!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Tracking a loop in JavaScript doesn't seem to work?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Why Your JavaScript Loop Doesn't Display Intermediate Results
When you're working with loops in JavaScript, it can be frustrating if you expect to see live updates as the loop progresses, but instead, you only get the final result. If you've tried something similar to the below code and are puzzled why it behaves in this way, you're not alone! Let's break down the issue and explore the solution together.
The Problem: Code Behavior
In the provided code snippet excerpts, the intent is to display the current index of a loop in a text input element. However, when running the loop:
[[See Video to Reveal this Text or Code Snippet]]
You would only see the message "End" in the text element after the loop has finished executing. So, what gives?
Understanding Synchronous Execution
The key reason you're only seeing the end message is that JavaScript executes code synchronously, which means it runs code in sequence, one after the other. Here's what this means in your context:
Blocking Execution: The loop runs and blocks the UI thread until it completes. While it's running, the browser doesn't get a chance to update the UI.
Final Output Only: Since the text input gets updated only after the loop finishes, it reflects only the last value assigned (in this case, always "End").
Possible Solutions
To resolve this and see the intermediate values as the loop progresses, you can use asynchronous programming techniques. Below are a couple of approaches.
1. Use setTimeout
One simple solution is to use setTimeout to break the loop into smaller chunks. This allows the browser to render changes in the UI while the loop processes:
[[See Video to Reveal this Text or Code Snippet]]
Here, setTimeout(update, 0) tells JavaScript to wait until the current call stack is clear before executing the next iteration, allowing the UI to update.
2. Use Promises and async/await
If you are comfortable with modern JavaScript, you could also use Promises along with async/await. Here's how that could look:
[[See Video to Reveal this Text or Code Snippet]]
The requestAnimationFrame function allows the browser to perform updates, thus preventing blocking execution.
Conclusion
Now you have a clearer understanding of why your loop didn't yield the results you wanted and how to resolve it. By introducing asynchronous behavior through techniques like setTimeout or using async/await, you can create more responsive and dynamic applications. Moving forward with this knowledge will help enhance the user experience in your JavaScript applications!
If you have further questions or need additional clarification, feel free to drop a comment below!