filmov
tv
Solving the not defined Error in Nested Functions in Python
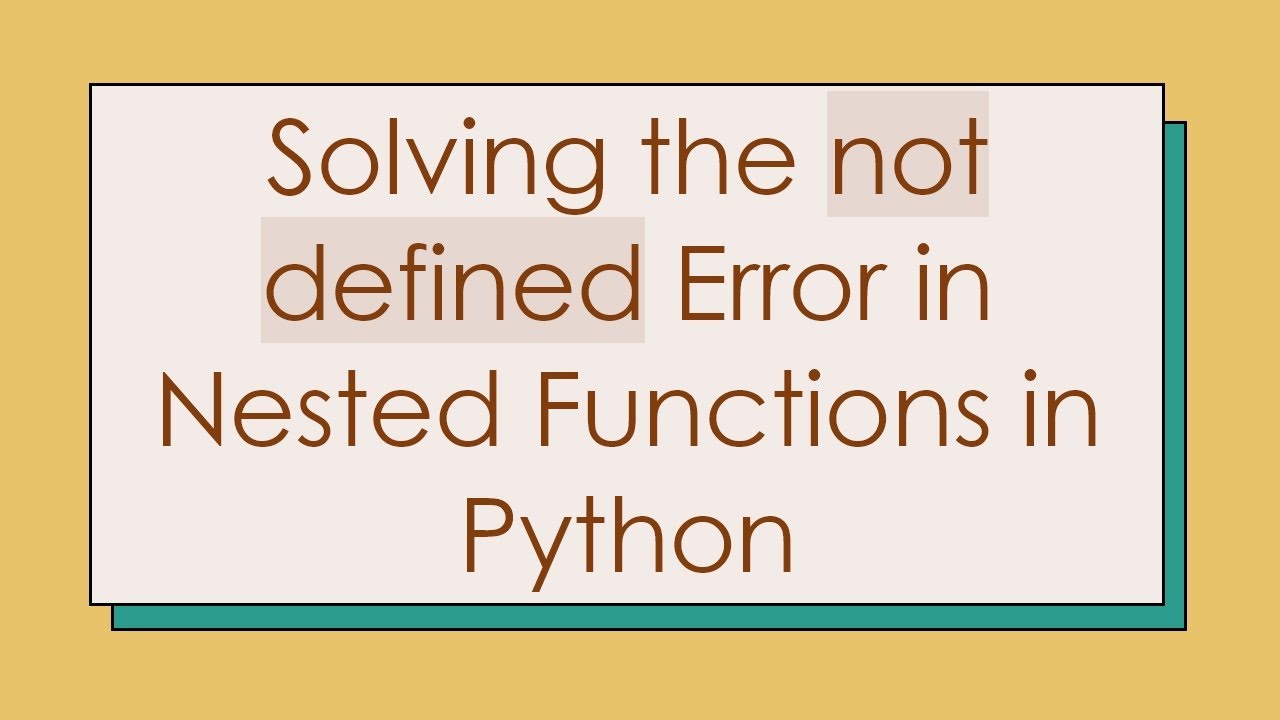
Показать описание
Learn how to fix the 'not defined' error when dealing with return values from nested functions in Python. This guide will guide you step-by-step through the solution.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Return value from nested function 'not defined'
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Solving the not defined Error in Nested Functions in Python
In Python, working with nested functions can sometimes lead to confusion, especially when dealing with return values. A common issue arises when you attempt to use variables returned from a nested function but encounter a not defined error. In this guide, we’ll break down this problem and provide a clear solution.
Understanding the Problem
Imagine you have an asynchronous function that contains a nested function. This nested function is supposed to return two values, such as a wallet and a private key. However, when you try to use one of those values outside of the nested function, you get an error indicating that the variable is not defined.
Here’s a simplified version of what the code might look like:
[[See Video to Reveal this Text or Code Snippet]]
In the above code, you’re trying to use private_key after calling get_user_wallet(), but since private_key is defined within that nested function, it is not accessible outside of it.
The Solution
To successfully retrieve the values from your nested function and avoid the not defined error, you need to ensure that you capture the return values from get_user_wallet(). Here’s how you can do that:
Step 1: Capture the Return Values
You need to assign the values returned from the get_user_wallet() function to variables. Here’s the corrected code:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Use the Values
Now that you have the private_key available in your main function, you can use it to create the view as intended. Further, you can send the response without running into issues.
Summary of the Fix:
Always remember to capture return values from nested functions.
Ensure the variables you need to use afterwards are defined in the correct scope.
Conclusion
Dealing with nested functions in Python can be tricky, particularly when it comes to managing variable scope. By understanding how to properly capture and utilize return values, you can avoid common pitfalls such as the not defined error, making your coding experience smoother and more efficient.
If you have further questions or need clarification on any topic, feel free to ask. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Return value from nested function 'not defined'
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Solving the not defined Error in Nested Functions in Python
In Python, working with nested functions can sometimes lead to confusion, especially when dealing with return values. A common issue arises when you attempt to use variables returned from a nested function but encounter a not defined error. In this guide, we’ll break down this problem and provide a clear solution.
Understanding the Problem
Imagine you have an asynchronous function that contains a nested function. This nested function is supposed to return two values, such as a wallet and a private key. However, when you try to use one of those values outside of the nested function, you get an error indicating that the variable is not defined.
Here’s a simplified version of what the code might look like:
[[See Video to Reveal this Text or Code Snippet]]
In the above code, you’re trying to use private_key after calling get_user_wallet(), but since private_key is defined within that nested function, it is not accessible outside of it.
The Solution
To successfully retrieve the values from your nested function and avoid the not defined error, you need to ensure that you capture the return values from get_user_wallet(). Here’s how you can do that:
Step 1: Capture the Return Values
You need to assign the values returned from the get_user_wallet() function to variables. Here’s the corrected code:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Use the Values
Now that you have the private_key available in your main function, you can use it to create the view as intended. Further, you can send the response without running into issues.
Summary of the Fix:
Always remember to capture return values from nested functions.
Ensure the variables you need to use afterwards are defined in the correct scope.
Conclusion
Dealing with nested functions in Python can be tricky, particularly when it comes to managing variable scope. By understanding how to properly capture and utilize return values, you can avoid common pitfalls such as the not defined error, making your coding experience smoother and more efficient.
If you have further questions or need clarification on any topic, feel free to ask. Happy coding!