filmov
tv
How many times is a sorted array rotated?
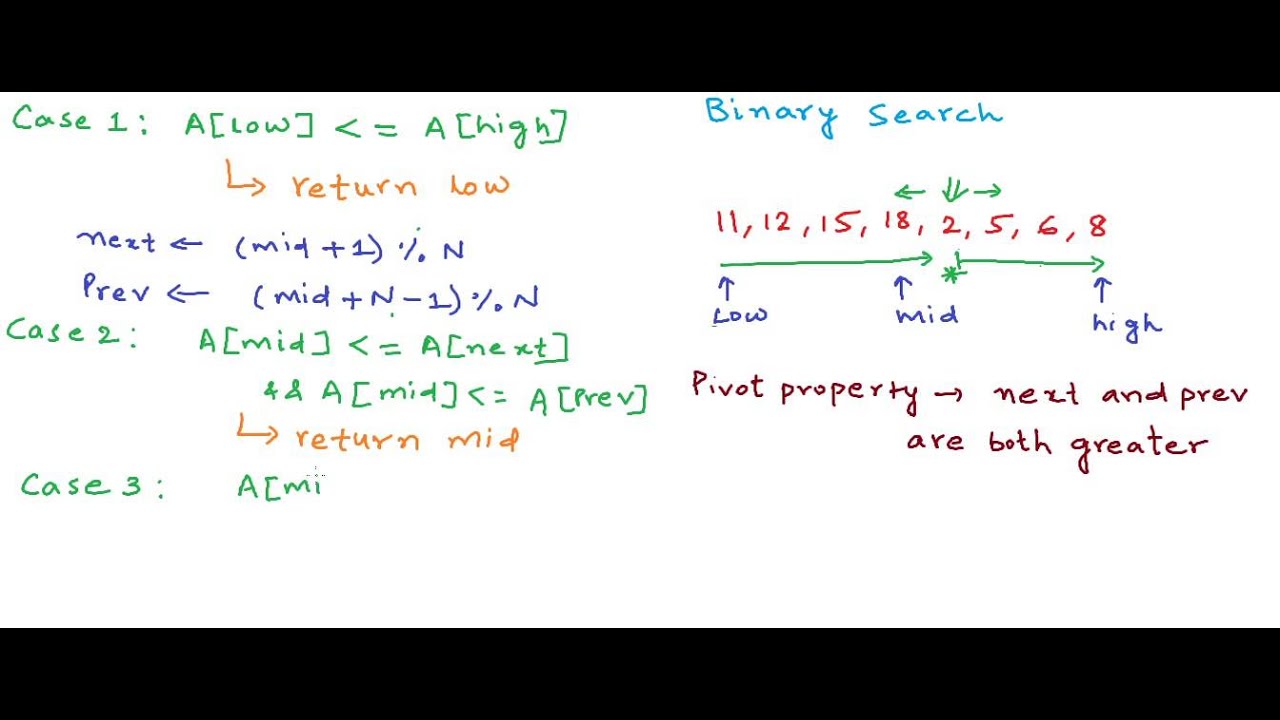
Показать описание
Tutorial Level: Intermediate
In this lesson we will be solving a programming interview question to find out the number of rotations of a sorted array in O(log n) time using binary search.
In this lesson we will be solving a programming interview question to find out the number of rotations of a sorted array in O(log n) time using binary search.
Akcent - How Many Times (Official Video)
DJ Big N, @ayrastarrofficial & @OxladeOfficial - How Many Times (Official Music Video)
Akcent - How Many Times (Lyrics)
HOW MANY TIMES DO I HAVE TO SAY IT #couple #couplecomedy #shorts
M1llionz - How Many Times feat. Lotto Ash
How Many Times
The Away Days - ''How Many Times'' (Official Music Video)
How many times does my Pokemon need to evolve to....
Joe Nester - How Many Times (Lyric Video)
How Many Times
How Many Times, How Many Lies
Elderbrook x Andhim - How Many Times
Stephen Marley - Pale Moonlight (How many times)
How Many Times (Will You Let Him Break Your Heart)
Led Zeppelin - How Many More Times (Official Audio)
Baby Money ft. Skilla Baby - How Many Times (Official Music Video)
DOPE LEMON - How Many Times (Official Visualiser)
SANCTUS REAL | HOW MANY TIMES - Official Lyric Video
So Many Times
Eliane Elias - How Many Times (Visualizer)
Aston Merrygold - How Many Times (Official Video)
How Many Times
Elderbrook & Andhim - How Many Times
Elderbrook - How Many Times
Комментарии