filmov
tv
Ultimate Python Turtle Graphics Tutorial: Space Arena 9 (Collision Detection)
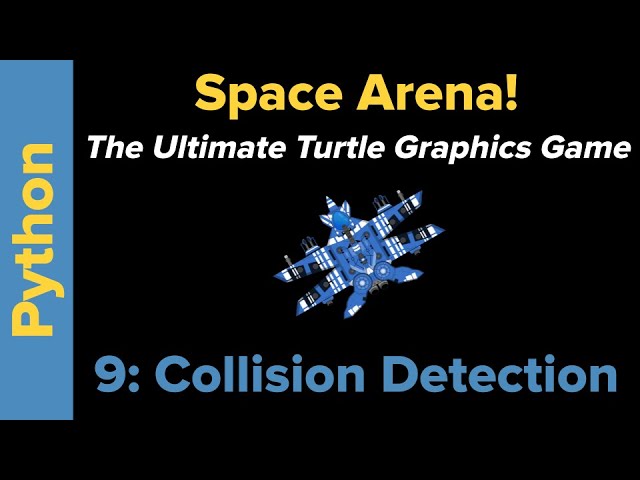
Показать описание
Welcome to the Ultimate Python Turtle Graphics Tutorial! In it, I will walk you step-by-step how to create an original shoot'em up game called Space Arena. This is an intermediate level tutorial and uses concepts such as classes, and inheritance in its design as well as some geometry to make the spaceship physics more realistic.
Collision Code:
# NOTE: YouTube doesn't allow angle brackets in descriptions so change lt to the less than symbol and gt to the greater than symbol
def is_collision(self, other):
return True
else:
return False
NEED HELP?
❤️❤️ SHOW SOME LOVE AND SUPPORT THE CHANNEL ❤️❤️
Click Join and Become a Channel Member Today!
Channel members can get preferential comment replies, early access to new content, members only live streams, and access to my private Discord.
Amazon Affiliate Links
Other Affiliate Links
LINKS
LEARN MORE PYTHON
LEARN MORE JAVA
#Python #Tutorial #Beginner
Collision Code:
# NOTE: YouTube doesn't allow angle brackets in descriptions so change lt to the less than symbol and gt to the greater than symbol
def is_collision(self, other):
return True
else:
return False
NEED HELP?
❤️❤️ SHOW SOME LOVE AND SUPPORT THE CHANNEL ❤️❤️
Click Join and Become a Channel Member Today!
Channel members can get preferential comment replies, early access to new content, members only live streams, and access to my private Discord.
Amazon Affiliate Links
Other Affiliate Links
LINKS
LEARN MORE PYTHON
LEARN MORE JAVA
#Python #Tutorial #Beginner
How to create graphics using Python turtle 🐍🐢 #coding
Ultimate Python Turtle Graphics Tutorial: Space Arena Introduction
Python Turtle Graphics 7 | multi colors designs #CodingWithKarthik
Ultimate Python Turtle Graphics Tutorial: Space Arena 8 (Player Missile)
Ultimate Python Turtle Graphics Tutorial: Space Arena 3 (Sprite Update)
Amazing Flower Design using Python turtle 🐢 #python #coding #funny #viral #trending #design
Ultimate Python Turtle Graphics Tutorial: Space Arena 2 (Sprite Class)
Python turtle graphic design😜🥰||Python coding status#shorts #shortvideo#viral #python #css #trending...
Python || turtle || flower || Adobe illustrator || #foryou #python #graphics #designs #turtle#heart
Python turtle graphic design||Python coding status🥰😍#shorts #shortvideo#viral #python #css #trending...
Python turtle graphic design🥰😍||Python coding status#shorts #shortvideo#viral #python #css #trending...
Python turtle graphic design||Python coding status🥰😍#shorts #shortvideo#viral #python #css #trending...
Python turtle graphic design||Python coding status🥰😍#shorts #shortvideo#viral #python #css #trending...
Python turtle graphic design🥰😍||Python coding status#shorts #shortvideo#viral #python #css #trending...
Ultimate Python Turtle Graphics Tutorial: Space Arena 1 (Getting Started)
Python turtle graphic design||Python coding status🥰😍#shorts#viral #shortvideo #css #trending #python...
Python turtle graphic design😜🥰||Python coding status#shorts #shortvideo#viral #python #css #trending...
Ultimate Python Turtle Graphics Tutorial: Space Arena 18 (Splash Screen)
Ultimate Python Turtle Graphics Tutorial: Space Arena 6 (Health Meter)
Ultimate Python Turtle Graphics Tutorial: Space Arena 4 (Player Class)
Ultimate Python Turtle Graphics Tutorial: Space Arena 10 (Level Setup)
Python turtle graphics design ☺️ || Python coding status 😊 #shorts #python # programming #turtle...
Ultimate Python Turtle Graphics Tutorial: Space Arena 15 (Drawing Text)
Python turtle graphic design🥰||Python coding status😍#shorts #shortvideo #trending#viral #python #css...
Комментарии