filmov
tv
10 Important Concepts to Learn in Python | Must know Python Concepts
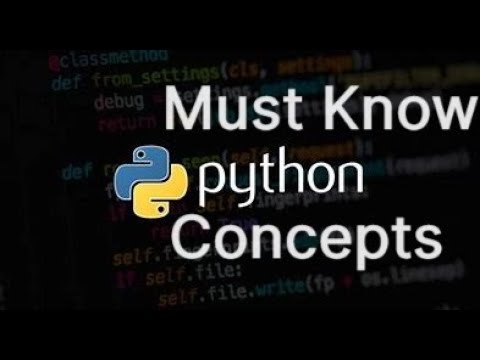
Показать описание
Map Function
Python has an inbuilt function called map() which permits us to process all the elements present in an iterable without explicitly using a looping construct.
itertools
Python has an amazing standard library called itertools which provides a number of functions that help in writing clean, fast, and memory-efficient code due to lazy evaluation.
itertools are: count(), cycle(), repeat(), accumulate(), product(), permutations(), combinations() etc.
Lambda Function
Python’s lambda functions are small anonymous functions as they do not have a name and are contained in a single line of code.
The keyword ‘def’ is used to define functions in Python but lambda functions are rather defined by the keyword ‘lambda’. They can take any number of arguments, but the number of expressions can only be one.
Syntax:lambda arguments : expression
Exception Handling
Exceptions are types of errors that occur when the program is being executed and change the normal flow of the program
try, except, and finally are used to handle exceptions in Python.
The keyword try is used to wrap a block of code that can throw errors. except is used to wrap a block of code to be executed when an exception is raised and handles the error. finally lets us execute the code no matter what.
Collections
Collections in Python are general purpose inbuilt containers like sets, tuples, dictionaries, and lists.
Collections include namedtuple() which is a function for creating tuple subclasses with named fields.
OrderedDict which is a dict subclass that remembers the order entries that were added since Python dict isn’t ordered.
Counter that is used for counting hashable objects.
ChainMap that is used for creating a single view of multiple mappings, etc
Generators
Generators in Python are a special type of function that rather than returning a single value, returns an iterator object which is a sequence of values.
It is a utility to create your own iterator function.
The keyword yield is used in the generator function instead of the return keyword.
The difference between yield and return is that return terminates the function but yield only pauses the execution of the function and returns the value against it each time.
Magic Methods
Also called double underscore methods, magic methods are special types of functions that are invoked internally.
They start and end with double underscores.
Threading
A Thread is the smallest unit or process that can be scheduled by an operating system.
Python contains the Thread class which aids in multithreaded programming.
Multithreading is mainly used to speed up the computation to a huge extent as now more than one thread will be performing tasks.
To implement threading in Python, you have to use the threading module.
Regular Expressions
Python regular expressions contain specific characters as patterns to be matched.
It is used to check if a string or a set of strings contains a specific pattern. It is extremely powerful, elegant, and concise along with being fast.
To use Python’s regular expressions, you need to import the re module which contains functions that help in pattern matching like findall(), search(), split(), etc.
Python has an inbuilt function called map() which permits us to process all the elements present in an iterable without explicitly using a looping construct.
itertools
Python has an amazing standard library called itertools which provides a number of functions that help in writing clean, fast, and memory-efficient code due to lazy evaluation.
itertools are: count(), cycle(), repeat(), accumulate(), product(), permutations(), combinations() etc.
Lambda Function
Python’s lambda functions are small anonymous functions as they do not have a name and are contained in a single line of code.
The keyword ‘def’ is used to define functions in Python but lambda functions are rather defined by the keyword ‘lambda’. They can take any number of arguments, but the number of expressions can only be one.
Syntax:lambda arguments : expression
Exception Handling
Exceptions are types of errors that occur when the program is being executed and change the normal flow of the program
try, except, and finally are used to handle exceptions in Python.
The keyword try is used to wrap a block of code that can throw errors. except is used to wrap a block of code to be executed when an exception is raised and handles the error. finally lets us execute the code no matter what.
Collections
Collections in Python are general purpose inbuilt containers like sets, tuples, dictionaries, and lists.
Collections include namedtuple() which is a function for creating tuple subclasses with named fields.
OrderedDict which is a dict subclass that remembers the order entries that were added since Python dict isn’t ordered.
Counter that is used for counting hashable objects.
ChainMap that is used for creating a single view of multiple mappings, etc
Generators
Generators in Python are a special type of function that rather than returning a single value, returns an iterator object which is a sequence of values.
It is a utility to create your own iterator function.
The keyword yield is used in the generator function instead of the return keyword.
The difference between yield and return is that return terminates the function but yield only pauses the execution of the function and returns the value against it each time.
Magic Methods
Also called double underscore methods, magic methods are special types of functions that are invoked internally.
They start and end with double underscores.
Threading
A Thread is the smallest unit or process that can be scheduled by an operating system.
Python contains the Thread class which aids in multithreaded programming.
Multithreading is mainly used to speed up the computation to a huge extent as now more than one thread will be performing tasks.
To implement threading in Python, you have to use the threading module.
Regular Expressions
Python regular expressions contain specific characters as patterns to be matched.
It is used to check if a string or a set of strings contains a specific pattern. It is extremely powerful, elegant, and concise along with being fast.
To use Python’s regular expressions, you need to import the re module which contains functions that help in pattern matching like findall(), search(), split(), etc.