filmov
tv
Understanding the * Operator in Python: Unpacking Function Arguments
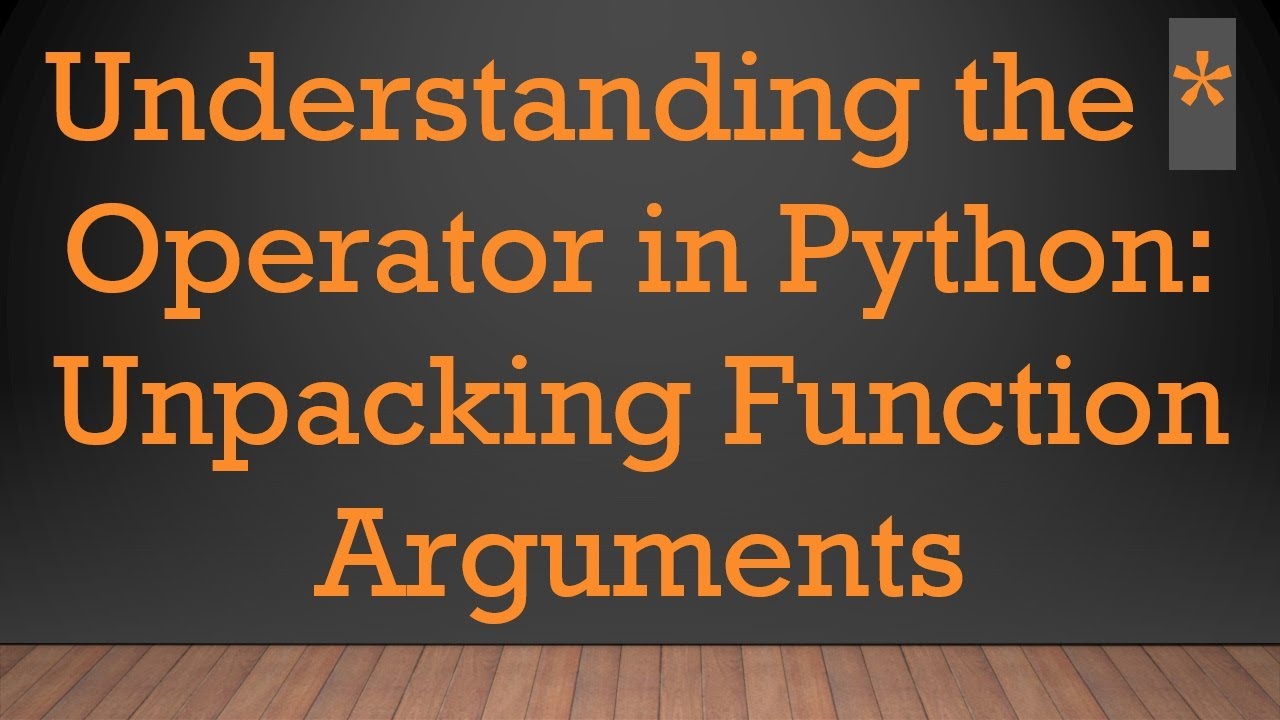
Показать описание
Discover how the `*` operator in Python unpacks arguments in function calls, enhancing your coding skills and understanding of syntax.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Putting asterisk before function call in python
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the * Operator in Python: Unpacking Function Arguments
When diving into Python, you might stumble upon unexpected syntax, particularly the asterisk (*) before a function call. This small character can cause confusion, especially for those new to Python or programming in general. In this guide, we will clarify what the * operator does and how it can be effectively utilized in your Python functions.
The Problem: Confusion Over Syntax
Many Python developers have experienced confusion when they see the * operator used before a function call. One user shared their frustration with attempting to use this syntax, leading to compilation issues. They provided a sample code but were unsure of the purpose and functionality of the asterisk in that context.
Here’s a simplified overview of the user's code for clarity:
[[See Video to Reveal this Text or Code Snippet]]
While they attempted to unpack the return values from get_ab into get_value, the misunderstanding of how * operates led to compilation errors.
The Solution: Unpacking with *
What Does * Do?
The asterisk (*) operator is used in Python for unpacking argument lists. This means it allows you to take collections like lists or tuples and spread their elements into the function arguments. Rather than passing a single collection object, you can unpack the values directly into a function.
Example Explained
Let's break down a more illustrative example to demonstrate the unpacking of function arguments using the asterisk:
[[See Video to Reveal this Text or Code Snippet]]
Functions Defined:
list_to_tuple takes a list and returns its first two elements as a tuple.
output takes two parameters and prints them.
List Initialization:
We define a list l containing two elements: [1, 2].
The Asterisk in Action:
When we call output(*list_to_tuple(l)), Python processes list_to_tuple(l) first, which returns the values (1, 2). The * operator then unpacks this tuple into the output function.
As a result, x becomes 1, and y becomes 2, resulting in the output 1 2 being printed.
Benefits of Unpacking
Unpacking arguments using the * operator provides several benefits:
Enhanced Readability: Makes it clearer that you're passing multiple values without creating additional variables.
Flexibility: Allows you to pass variable-length argument lists to functions seamlessly.
Conciseness: Reduces the amount of code needed by avoiding intermediate storage or multiple lines of parameter assignment.
Final Thoughts
In Python, the * operator can seem trivial but serves a powerful purpose when unpacking arguments. Understanding its use can significantly improve the clarity and efficiency of your code. Next time you see an asterisk before a function call, you'll know that it's unpacking values directly into that function's parameters.
By mastering this simple yet effective syntax, you're one step closer to being a proficient Python programmer! Keep experimenting with function calls and discover the elegance of Python.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Putting asterisk before function call in python
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the * Operator in Python: Unpacking Function Arguments
When diving into Python, you might stumble upon unexpected syntax, particularly the asterisk (*) before a function call. This small character can cause confusion, especially for those new to Python or programming in general. In this guide, we will clarify what the * operator does and how it can be effectively utilized in your Python functions.
The Problem: Confusion Over Syntax
Many Python developers have experienced confusion when they see the * operator used before a function call. One user shared their frustration with attempting to use this syntax, leading to compilation issues. They provided a sample code but were unsure of the purpose and functionality of the asterisk in that context.
Here’s a simplified overview of the user's code for clarity:
[[See Video to Reveal this Text or Code Snippet]]
While they attempted to unpack the return values from get_ab into get_value, the misunderstanding of how * operates led to compilation errors.
The Solution: Unpacking with *
What Does * Do?
The asterisk (*) operator is used in Python for unpacking argument lists. This means it allows you to take collections like lists or tuples and spread their elements into the function arguments. Rather than passing a single collection object, you can unpack the values directly into a function.
Example Explained
Let's break down a more illustrative example to demonstrate the unpacking of function arguments using the asterisk:
[[See Video to Reveal this Text or Code Snippet]]
Functions Defined:
list_to_tuple takes a list and returns its first two elements as a tuple.
output takes two parameters and prints them.
List Initialization:
We define a list l containing two elements: [1, 2].
The Asterisk in Action:
When we call output(*list_to_tuple(l)), Python processes list_to_tuple(l) first, which returns the values (1, 2). The * operator then unpacks this tuple into the output function.
As a result, x becomes 1, and y becomes 2, resulting in the output 1 2 being printed.
Benefits of Unpacking
Unpacking arguments using the * operator provides several benefits:
Enhanced Readability: Makes it clearer that you're passing multiple values without creating additional variables.
Flexibility: Allows you to pass variable-length argument lists to functions seamlessly.
Conciseness: Reduces the amount of code needed by avoiding intermediate storage or multiple lines of parameter assignment.
Final Thoughts
In Python, the * operator can seem trivial but serves a powerful purpose when unpacking arguments. Understanding its use can significantly improve the clarity and efficiency of your code. Next time you see an asterisk before a function call, you'll know that it's unpacking values directly into that function's parameters.
By mastering this simple yet effective syntax, you're one step closer to being a proficient Python programmer! Keep experimenting with function calls and discover the elegance of Python.