filmov
tv
How to Convert Array and Sub-array to Collection in Laravel
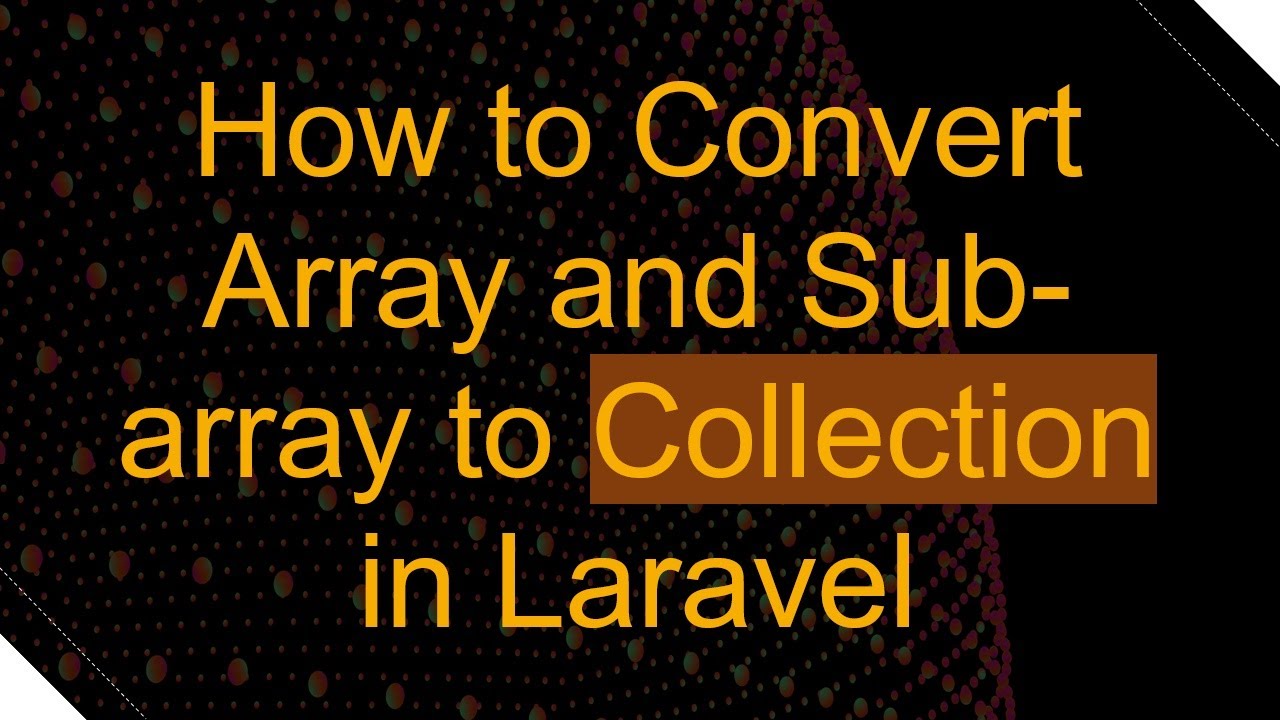
Показать описание
Learn how to efficiently convert arrays and sub-arrays into `collections` in Laravel to enhance your static data management.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to convert array and sub array to collection in Laravel?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Convert Array and Sub-array to Collection in Laravel
When developing applications using Laravel, you'll often find yourself handling various types of data structures. One common scenario is needing to convert static data, especially arrays and sub-arrays, into collections. Collections in Laravel are a powerful way to work with arrays, providing a range of helpful methods that make data manipulation more seamless. In this post, we will walk you through the process of converting your array and its sub-array to collections efficiently.
Problem Overview
Imagine you have a static data structure, such as a menu list, defined in a PHP array, like so:
[[See Video to Reveal this Text or Code Snippet]]
In this example, the menu_list contains an array of menu items, each with its own properties, and in some cases, a children property representing sub-items. You may want to convert not just the main array but also any nested sub-arrays into collections.
The Challenge
You might start with a conversion function like this:
[[See Video to Reveal this Text or Code Snippet]]
This function works fine for converting the main array elements, but it falls short when it comes to converting the children sub-array, which remains untouched. To tackle this, we'll need a recursive function.
The Solution: Recursive Function
Step 1: Create the Recursive Method
First, we need to define a method that can handle the conversion of both the main array and any nested arrays. Below is how the complete solution looks:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Explanation of the Code
Main Method: convertToCollection()
This is the entry point to convert your menu_list to a collection.
Recursive Method: menuList($list)
Here, we collect the items into a Laravel collection.
The use of map allows us to iterate through each item in the provided list.
The is_array() check identifies if the current $voucher is an array. If it is, the method calls itself recursively to convert the nested array into a collection as well.
If it's not an array, it simply returns the current voucher.
Step 3: Utilize the Collection
After defining the methods, you can now call the convertToCollection() method, and your static menu_list will be effectively converted into a nested collection format, preserving all the structure and properties.
Conclusion
By employing a recursive approach, you can effortlessly convert both arrays and sub-arrays into collections in Laravel. This not only facilitates easier access and manipulation of your data but also leverages Laravel’s powerful collection methods. Whether you are managing menu structures, configurations, or any other arrays with nested elements, this technique will streamline your data handling in Laravel.
Now, go ahead and try implementing this in your Laravel applications to see how it simplifies your data management tasks!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to convert array and sub array to collection in Laravel?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Convert Array and Sub-array to Collection in Laravel
When developing applications using Laravel, you'll often find yourself handling various types of data structures. One common scenario is needing to convert static data, especially arrays and sub-arrays, into collections. Collections in Laravel are a powerful way to work with arrays, providing a range of helpful methods that make data manipulation more seamless. In this post, we will walk you through the process of converting your array and its sub-array to collections efficiently.
Problem Overview
Imagine you have a static data structure, such as a menu list, defined in a PHP array, like so:
[[See Video to Reveal this Text or Code Snippet]]
In this example, the menu_list contains an array of menu items, each with its own properties, and in some cases, a children property representing sub-items. You may want to convert not just the main array but also any nested sub-arrays into collections.
The Challenge
You might start with a conversion function like this:
[[See Video to Reveal this Text or Code Snippet]]
This function works fine for converting the main array elements, but it falls short when it comes to converting the children sub-array, which remains untouched. To tackle this, we'll need a recursive function.
The Solution: Recursive Function
Step 1: Create the Recursive Method
First, we need to define a method that can handle the conversion of both the main array and any nested arrays. Below is how the complete solution looks:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Explanation of the Code
Main Method: convertToCollection()
This is the entry point to convert your menu_list to a collection.
Recursive Method: menuList($list)
Here, we collect the items into a Laravel collection.
The use of map allows us to iterate through each item in the provided list.
The is_array() check identifies if the current $voucher is an array. If it is, the method calls itself recursively to convert the nested array into a collection as well.
If it's not an array, it simply returns the current voucher.
Step 3: Utilize the Collection
After defining the methods, you can now call the convertToCollection() method, and your static menu_list will be effectively converted into a nested collection format, preserving all the structure and properties.
Conclusion
By employing a recursive approach, you can effortlessly convert both arrays and sub-arrays into collections in Laravel. This not only facilitates easier access and manipulation of your data but also leverages Laravel’s powerful collection methods. Whether you are managing menu structures, configurations, or any other arrays with nested elements, this technique will streamline your data handling in Laravel.
Now, go ahead and try implementing this in your Laravel applications to see how it simplifies your data management tasks!