filmov
tv
Mastering for Loops in Python: Simplifying Nested Dictionary Iteration
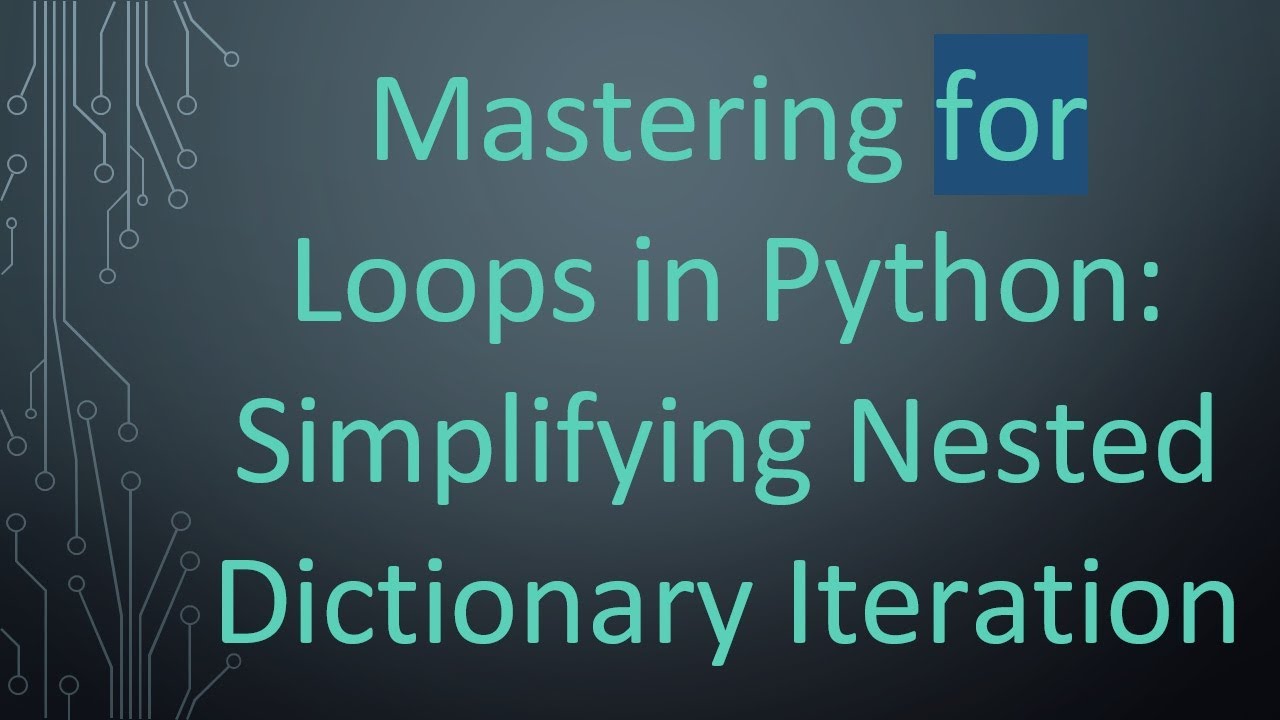
Показать описание
Discover how to efficiently loop through a nested dictionary in Python without unnecessary complexity. Learn to simplify your code while extracting critical information!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Python for loop through nested dictionary question?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering for Loops in Python: Simplifying Nested Dictionary Iteration
When working with complex data structures in Python, particularly nested dictionaries, many programmers face challenges regarding data access and iteration. A common query is: How can I efficiently loop through a nested dictionary to extract specific values without cluttering my code with unnecessary variables?
This guide will tackle that issue by providing a clean way to iterate through a nested dictionary containing personal information such as names, ages, and occupations without creating multiple additional variables within a loop. Let’s break down the solution step-by-step!
Understanding the Problem
Consider the following nested dictionary structure that contains data about multiple individuals:
[[See Video to Reveal this Text or Code Snippet]]
Your goal is to loop through this dictionary and print out the name, age, and occupation of each person. A common approach might involve using an inner loop to access these values. However, there’s a more efficient method.
The Inefficient Approach
Using an inner loop, you might write the following code:
[[See Video to Reveal this Text or Code Snippet]]
While this accomplishes the task, it can lead to more complicated and less readable code. Let’s see how we can streamline this process.
The Simplified Solution
Instead of using an inner loop, you can directly unpack the values from the nested dictionary. Here’s how:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Solution
Iterating through Values: Instead of iterating through the keys (which require a lookup for the nested values), we simply iterate through the values of the people dictionary.
Benefits of This Approach
Readability: The code is clearer and conveys its intention more effectively.
Efficiency: Eliminating unnecessary loops minimizes complexity and enhances performance when working with larger datasets.
Maintainability: This clear structure makes future changes or updates easier to implement.
Conclusion
Looping through a nested dictionary can be made significantly simpler and cleaner with the right approach. By unpacking values directly from the dictionary, you can reduce code complexity while maintaining efficiency and readability.
Next time you are faced with a similar challenge in Python, remember this technique, and you’ll find your code becoming more elegant and understandable. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Python for loop through nested dictionary question?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering for Loops in Python: Simplifying Nested Dictionary Iteration
When working with complex data structures in Python, particularly nested dictionaries, many programmers face challenges regarding data access and iteration. A common query is: How can I efficiently loop through a nested dictionary to extract specific values without cluttering my code with unnecessary variables?
This guide will tackle that issue by providing a clean way to iterate through a nested dictionary containing personal information such as names, ages, and occupations without creating multiple additional variables within a loop. Let’s break down the solution step-by-step!
Understanding the Problem
Consider the following nested dictionary structure that contains data about multiple individuals:
[[See Video to Reveal this Text or Code Snippet]]
Your goal is to loop through this dictionary and print out the name, age, and occupation of each person. A common approach might involve using an inner loop to access these values. However, there’s a more efficient method.
The Inefficient Approach
Using an inner loop, you might write the following code:
[[See Video to Reveal this Text or Code Snippet]]
While this accomplishes the task, it can lead to more complicated and less readable code. Let’s see how we can streamline this process.
The Simplified Solution
Instead of using an inner loop, you can directly unpack the values from the nested dictionary. Here’s how:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Solution
Iterating through Values: Instead of iterating through the keys (which require a lookup for the nested values), we simply iterate through the values of the people dictionary.
Benefits of This Approach
Readability: The code is clearer and conveys its intention more effectively.
Efficiency: Eliminating unnecessary loops minimizes complexity and enhances performance when working with larger datasets.
Maintainability: This clear structure makes future changes or updates easier to implement.
Conclusion
Looping through a nested dictionary can be made significantly simpler and cleaner with the right approach. By unpacking values directly from the dictionary, you can reduce code complexity while maintaining efficiency and readability.
Next time you are faced with a similar challenge in Python, remember this technique, and you’ll find your code becoming more elegant and understandable. Happy coding!