filmov
tv
Python Interview Question asked in Tiger Analytics
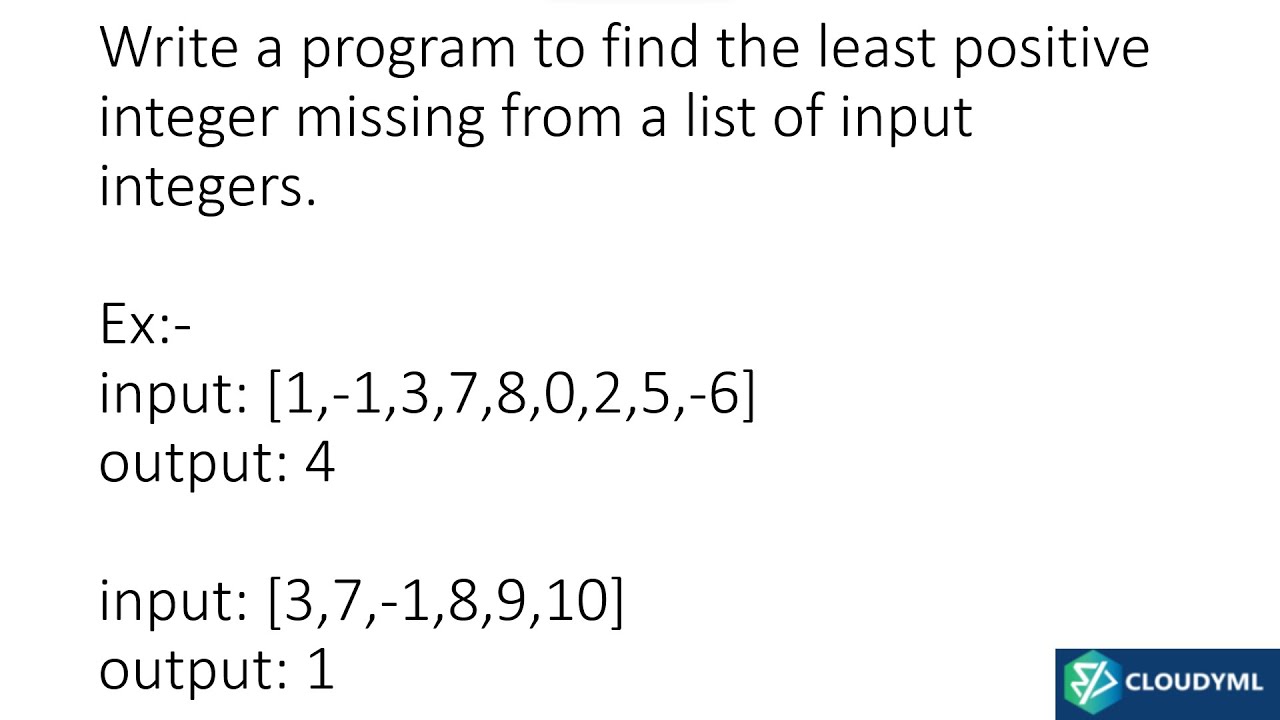
Показать описание
Check our Data Science & Analytics Courses on our website. We provide hands-on practical learning experience to our learners with 1-1 Live Doubt Clearance Support over Chat everyday and also you will learn Job Hunting Hacks. You will get projects and case studies that you can add in your resume and a lot more features are there inside this course.
➡️1. Data Science, Analytics & Job Hunting Super Combo Course (Paid Internship)
➡️1. Data Science, Analytics & Job Hunting Super Combo Course (Paid Internship)
Top 15 Python Interview Questions | Python Interview Questions And Answers | Intellipaat
Python for Coding Interviews - Everything you need to Know
Python Interview Questions and Answers - For Freshers and Experienced Candidates
Preparing for a Python Interview: 10 Things You Should Know
Most Asked Coding Interview Question (Don't Skip !!😮) #shorts
Fresher Python Mock Interview | Technical Round | PYTHON Interview for Fresher | Interview Questions
20 Mostly Asked Python Question | Top 10 Python Coding & 10 Theory Questions Asked During Interv...
Python Interview Questions | Python Interview Questions And Answers | Python Tutorial | Simplilearn
Top 5 Generative AI (Gen AI) Interview Questions | Asked in Interviews 2024
Solving Coding Interview Questions in Python on LeetCode (easy & medium problems)
Top 29 Python Interview Questions and Answers | Python Interview Questions and Answers
Most Asked Python Interview Question part 2 (Don't Skip !!😮) #shorts
Python Real Time Interview Questions & Answers Part-1
Python Mock Interview | Interview Questions for Senior Python Developers
Python Interview Questions | Python Tutorial | Intellipaat
Python Interview Questions | Python Mock Interview | Part 2
Python Technical Interviews - 5 Things you MUST KNOW
I gave 127 interviews. Top 5 Algorithms they asked me.
How to NOT Fail a Technical Interview
Python Interview Questions And Answers | Python Interview Questions | Python Training | Edureka
Python Interview Question #pythonprogramming #interview #python #programming
Top 15 Python Coding Interview Questions with Solutions - Do it Yourself
Top 100 Python Interview Questions | Python Programming | Crack Python Interview |Great Learning
HCL Interview Questions | Selenium + Python Interview| Selectors Hub
Комментарии