filmov
tv
How to Convert Byte Array to Blob in JavaScript for Angular
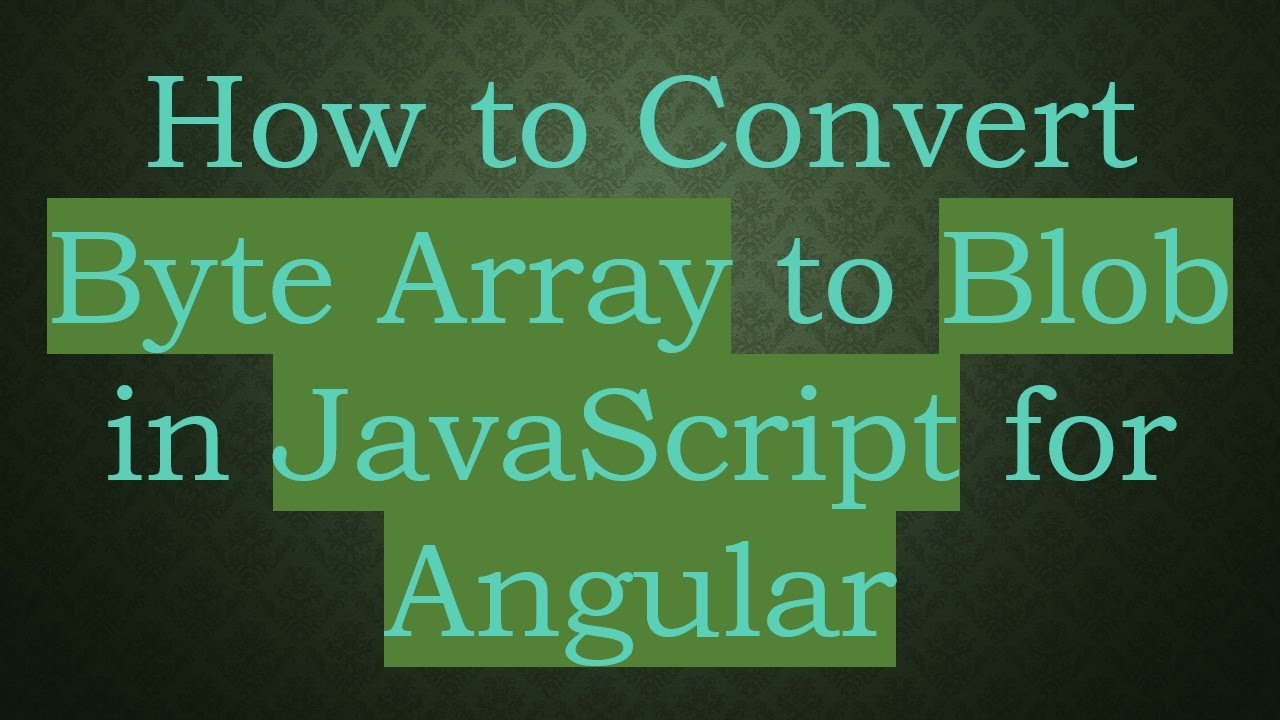
Показать описание
Discover a step-by-step guide on how to convert a byte array to a Blob in JavaScript for Angular applications, including tips and example code.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to convert byte array to blob in javascript for angular?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Convert Byte Array to Blob in JavaScript for Angular
When working with Angular applications that handle file uploads or downloads, you may often encounter a situation where you receive a file in the form of a byte array from your backend service. This commonly occurs when dealing with file formats like Excel, PDF, or images. The challenge lies in converting this byte array into a Blob object and subsequently into a file type that can be processed or downloaded by users. In this post, we will guide you through the steps needed to perform this conversion effectively.
Understanding the Problem
Receiving files as byte arrays is typical in modern web applications, especially when dealing with file uploads/downloads. When you are provided with a byte array:
It is essentially a numeric representation of binary data.
To use this data in the web environment, you need to convert it into a Blob or File type.
Why Use Blob?
Blob (Binary Large Object) is a data type that allows you to represent a file-like object of immutable, raw data. Using a Blob makes it easy to handle file uploading, displaying binary data (e.g., images) on a web page, and generating downloadable links for users.
Step-by-Step Solution
Let’s walk through the process of converting a byte array to a Blob in Angular using TypeScript. Below is a breakdown of the steps involved:
1. Receiving the Byte Array
When fetching your file from the backend, ensure you subscribe to your service's observable as follows:
[[See Video to Reveal this Text or Code Snippet]]
2. Understanding the Code
Base64 Decoding: The atob() function is used to decode the Base64 encoded string that represents your byte array.
Creating Uint8Array: The next line creates a new Uint8Array, which is ideal for constructing a Blob.
Creating Blob: The Blob constructor is called with the byteArray and the MIME type for Excel files.
Creating File: Finally, a new File object is created from the Blob. This File object can now be manipulated or downloaded.
3. MIME Types for Different Files
To ensure the files you handle are correctly identified, use a method to map file extensions to MIME types. Below is a simple function to determine the MIME type:
[[See Video to Reveal this Text or Code Snippet]]
4. Putting It All Together
Integrate the MIME type retrieval into your file handling workflow:
[[See Video to Reveal this Text or Code Snippet]]
This ensures that your Blob is created with the correct MIME type, making it easier for browsers and applications to handle downloads correctly.
Conclusion
Converting a byte array to a Blob in JavaScript for Angular applications is a straightforward process once you have the right approach. By following the steps outlined in this guide, you can seamlessly manage file downloads without hassle.
With this knowledge, you'll be better equipped to work with file data in your Angular applications, ensuring users have a smooth experience when handling files.
If you have any questions or need further clarification on the topic, feel free to drop a comment below!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to convert byte array to blob in javascript for angular?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Convert Byte Array to Blob in JavaScript for Angular
When working with Angular applications that handle file uploads or downloads, you may often encounter a situation where you receive a file in the form of a byte array from your backend service. This commonly occurs when dealing with file formats like Excel, PDF, or images. The challenge lies in converting this byte array into a Blob object and subsequently into a file type that can be processed or downloaded by users. In this post, we will guide you through the steps needed to perform this conversion effectively.
Understanding the Problem
Receiving files as byte arrays is typical in modern web applications, especially when dealing with file uploads/downloads. When you are provided with a byte array:
It is essentially a numeric representation of binary data.
To use this data in the web environment, you need to convert it into a Blob or File type.
Why Use Blob?
Blob (Binary Large Object) is a data type that allows you to represent a file-like object of immutable, raw data. Using a Blob makes it easy to handle file uploading, displaying binary data (e.g., images) on a web page, and generating downloadable links for users.
Step-by-Step Solution
Let’s walk through the process of converting a byte array to a Blob in Angular using TypeScript. Below is a breakdown of the steps involved:
1. Receiving the Byte Array
When fetching your file from the backend, ensure you subscribe to your service's observable as follows:
[[See Video to Reveal this Text or Code Snippet]]
2. Understanding the Code
Base64 Decoding: The atob() function is used to decode the Base64 encoded string that represents your byte array.
Creating Uint8Array: The next line creates a new Uint8Array, which is ideal for constructing a Blob.
Creating Blob: The Blob constructor is called with the byteArray and the MIME type for Excel files.
Creating File: Finally, a new File object is created from the Blob. This File object can now be manipulated or downloaded.
3. MIME Types for Different Files
To ensure the files you handle are correctly identified, use a method to map file extensions to MIME types. Below is a simple function to determine the MIME type:
[[See Video to Reveal this Text or Code Snippet]]
4. Putting It All Together
Integrate the MIME type retrieval into your file handling workflow:
[[See Video to Reveal this Text or Code Snippet]]
This ensures that your Blob is created with the correct MIME type, making it easier for browsers and applications to handle downloads correctly.
Conclusion
Converting a byte array to a Blob in JavaScript for Angular applications is a straightforward process once you have the right approach. By following the steps outlined in this guide, you can seamlessly manage file downloads without hassle.
With this knowledge, you'll be better equipped to work with file data in your Angular applications, ensuring users have a smooth experience when handling files.
If you have any questions or need further clarification on the topic, feel free to drop a comment below!